How do I use cursors in PL/SQL to process multiple rows of data?
How to Use Cursors in PL/SQL to Process Multiple Rows of Data
Cursors in PL/SQL provide a mechanism to process data row by row from a SQL query's result set. They act as a pointer to a result set, allowing you to fetch and manipulate individual rows. To use a cursor, you first declare it, then open it to execute the query, fetch rows one at a time, and finally close it. Here's a breakdown:
-
Declaration: You declare a cursor using the
CURSOR
keyword, followed by a name and the SQL query. The query should select the columns you need to process.DECLARE CURSOR emp_cursor IS SELECT employee_id, last_name, salary FROM employees WHERE department_id = 10; BEGIN -- Cursor operations will go here END; /
Copy after login Opening: The
OPEN
statement executes the query associated with the cursor and positions the cursor before the first row.OPEN emp_cursor;
Copy after loginFetching: The
FETCH
statement retrieves a row from the result set and places the values into variables. You need to declare variables that match the data types of the columns selected in the cursor's query.DECLARE employee_id employees.employee_id%TYPE; last_name employees.last_name%TYPE; salary employees.salary%TYPE; CURSOR emp_cursor IS ...; -- as declared above BEGIN OPEN emp_cursor; LOOP FETCH emp_cursor INTO employee_id, last_name, salary; EXIT WHEN emp_cursor%NOTFOUND; -- Process the fetched row here DBMS_OUTPUT.PUT_LINE('Employee ID: ' || employee_id || ', Name: ' || last_name || ', Salary: ' || salary); END LOOP; CLOSE emp_cursor; END; /
Copy after loginClosing: The
CLOSE
statement releases the resources held by the cursor. It's crucial to close cursors to prevent resource leaks.CLOSE emp_cursor;
Copy after login
The emp_cursor%NOTFOUND
attribute is checked after each FETCH
. When no more rows are available, it becomes TRUE
, and the loop terminates. This is the standard way to iterate through the rows returned by a cursor.
What are the Different Types of Cursors Available in PL/SQL and When Should I Use Each One?
PL/SQL offers several types of cursors, each with its strengths and weaknesses:
- Implicit Cursors: These are automatically created by PL/SQL when you execute a single
SELECT INTO
statement. They are hidden from the programmer and are automatically managed by the PL/SQL engine. Use them for simple queries retrieving a single row. If the query returns more than one row, it raises aTOO_MANY_ROWS
exception. - Explicit Cursors: These are declared and managed explicitly by the programmer (as shown in the previous section). They provide more control over the retrieval and processing of multiple rows, handling various scenarios effectively. Use them for complex queries or when processing multiple rows.
- Ref Cursors: These are cursors that can be passed as parameters to procedures or functions. They allow dynamic SQL and more flexibility in handling data across different parts of your application. Use them for stored procedures that need to return result sets without knowing the exact structure of the data beforehand.
The choice depends on your needs: Use implicit cursors for simple single-row retrievals, explicit cursors for more complex multi-row processing with clear control, and ref cursors for dynamic SQL and procedure/function parameter passing.
How Can I Efficiently Handle Large Datasets Using Cursors in PL/SQL to Avoid Performance Issues?
Processing large datasets with cursors can be inefficient if not handled carefully. Here are some strategies to improve performance:
- Bulk Processing: Avoid row-by-row processing whenever possible. Use techniques like
FORALL
statements to perform operations on multiple rows at once. This significantly reduces context switching between the PL/SQL engine and the database server. - Minimize Cursor Operations: Limit the number of times you open and close cursors. Opening and closing a cursor has overhead. Try to process as much data as possible within a single cursor.
- Appropriate Indexing: Ensure that appropriate indexes exist on the tables involved in your queries to speed up data retrieval. The query used in the cursor definition is a regular SQL query, so indexing principles apply normally.
- Optimize Queries: Write efficient SQL queries for your cursors. Avoid
SELECT *
, instead specify only the columns needed. Use appropriateWHERE
clauses to filter data effectively. - Fetch in Batches: Instead of fetching one row at a time, fetch multiple rows in a batch using a loop and an array. This reduces the number of round trips to the database.
- Consider other methods: For very large datasets, consider using other techniques such as pipelined table functions or materialized views to improve performance beyond what is possible with cursors.
Can I Use FOR Loops with Cursors in PL/SQL to Simplify My Code and Improve Readability When Processing Multiple Rows?
Yes, you can and should often use FOR
loops with cursors to simplify your code and enhance readability. The FOR
loop implicitly handles the opening, fetching, and closing of the cursor, making the code more concise and easier to understand. This is especially beneficial when dealing with explicit cursors.
Instead of the manual LOOP
and FETCH
as shown before, you can use:
DECLARE CURSOR emp_cursor IS SELECT employee_id, last_name, salary FROM employees WHERE department_id = 10; BEGIN FOR emp_rec IN emp_cursor LOOP DBMS_OUTPUT.PUT_LINE('Employee ID: ' || emp_rec.employee_id || ', Name: ' || emp_rec.last_name || ', Salary: ' || emp_rec.salary); END LOOP; END; /
This FOR
loop automatically handles cursor iteration. The emp_rec
record variable automatically receives the values from each row fetched from the cursor. This approach is cleaner, more readable, and less prone to errors compared to manually managing the cursor. It's the preferred method for most cursor-based row processing in PL/SQL.
The above is the detailed content of How do I use cursors in PL/SQL to process multiple rows of data?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
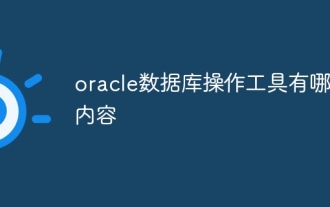
In addition to SQL*Plus, there are tools for operating Oracle databases: SQL Developer: free tools, interface friendly, and support graphical operations and debugging. Toad: Business tools, feature-rich, excellent in database management and tuning. PL/SQL Developer: Powerful tools for PL/SQL development, code editing and debugging. Dbeaver: Free open source tool, supports multiple databases, and has a simple interface.
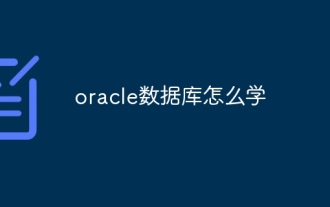
There are no shortcuts to learning Oracle databases. You need to understand database concepts, master SQL skills, and continuously improve through practice. First of all, we need to understand the storage and management mechanism of the database, master the basic concepts such as tables, rows, and columns, and constraints such as primary keys and foreign keys. Then, through practice, install the Oracle database, start practicing with simple SELECT statements, and gradually master various SQL statements and syntax. After that, you can learn advanced features such as PL/SQL, optimize SQL statements, and design an efficient database architecture to improve database efficiency and security.
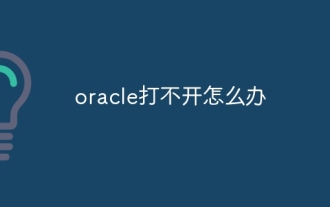
Solutions to Oracle cannot be opened include: 1. Start the database service; 2. Start the listener; 3. Check port conflicts; 4. Set environment variables correctly; 5. Make sure the firewall or antivirus software does not block the connection; 6. Check whether the server is closed; 7. Use RMAN to recover corrupt files; 8. Check whether the TNS service name is correct; 9. Check network connection; 10. Reinstall Oracle software.
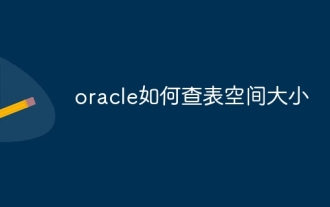
To query the Oracle tablespace size, follow the following steps: Determine the tablespace name by running the query: SELECT tablespace_name FROM dba_tablespaces; Query the tablespace size by running the query: SELECT sum(bytes) AS total_size, sum(bytes_free) AS available_space, sum(bytes) - sum(bytes_free) AS used_space FROM dba_data_files WHERE tablespace_
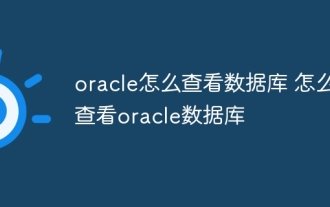
To view Oracle databases, you can use SQL*Plus (using SELECT commands), SQL Developer (graphy interface), or system view (displaying internal information of the database). The basic steps include connecting to the database, filtering data using SELECT statements, and optimizing queries for performance. Additionally, the system view provides detailed information on the database, which helps monitor and troubleshoot. Through practice and continuous learning, you can deeply explore the mystery of Oracle database.

The procedures, functions and packages in OraclePL/SQL are used to perform operations, return values and organize code, respectively. 1. The process is used to perform operations such as outputting greetings. 2. The function is used to calculate and return a value, such as calculating the sum of two numbers. 3. Packages are used to organize relevant elements and improve the modularity and maintainability of the code, such as packages that manage inventory.
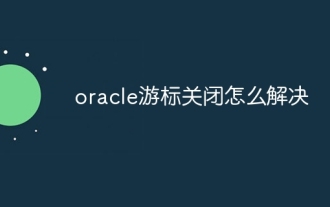
The method to solve the Oracle cursor closure problem includes: explicitly closing the cursor using the CLOSE statement. Declare the cursor in the FOR UPDATE clause so that it automatically closes after the scope is ended. Declare the cursor in the USING clause so that it automatically closes when the associated PL/SQL variable is closed. Use exception handling to ensure that the cursor is closed in any exception situation. Use the connection pool to automatically close the cursor. Disable automatic submission and delay cursor closing.
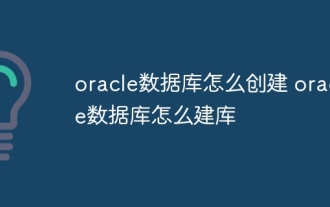
To create an Oracle database, the common method is to use the dbca graphical tool. The steps are as follows: 1. Use the dbca tool to set the dbName to specify the database name; 2. Set sysPassword and systemPassword to strong passwords; 3. Set characterSet and nationalCharacterSet to AL32UTF8; 4. Set memorySize and tablespaceSize to adjust according to actual needs; 5. Specify the logFile path. Advanced methods are created manually using SQL commands, but are more complex and prone to errors. Pay attention to password strength, character set selection, tablespace size and memory
