Flask Authentication With LDAP
This tutorial demonstrates Flask application user authentication via LDAP. We'll build a simple app with a home page and login page, verifying credentials against an LDAP server. Successful authentication grants access; otherwise, an error message displays. Basic Flask, LDAP, Flask-Login, and virtualenv
familiarity is assumed.
LDAP Server: We'll use Forum Systems' public LDAP test server for simplicity; no local server setup is needed.
Dependencies: Install necessary packages:
pip install ldap3 Flask-WTF flask-sqlalchemy Flask-Login
Application Structure:
<code>flask_app/ my_app/ - __init__.py auth/ - __init__.py - models.py - views.py static/ - css/ - js/ templates/ - base.html - home.html - login.html - run.py</code>
static
contains Bootstrap CSS and JS.
The Application:
flask_app/my_app/init.py:
from flask import Flask from flask_sqlalchemy import SQLAlchemy from flask_login import LoginManager app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:////tmp/test.db' app.config['WTF_CSRF_SECRET_KEY'] = 'random key for form' app.config['LDAP_PROVIDER_URL'] = 'ldap://ldap.forumsys.com:389/' app.config['LDAP_PROTOCOL_VERSION'] = 3 db = SQLAlchemy(app) app.secret_key = 'randon_key' login_manager = LoginManager() login_manager.init_app(app) login_manager.login_view = 'auth.login' ctx = app.test_request_context() ctx.push() from my_app.auth.views import auth app.register_blueprint(auth) db.create_all()
Configures the app, initializes extensions, and creates the database.
flask_app/my_app/auth/models.py:
import ldap3 from flask_wtf import Form from wtforms import StringField, PasswordField from wtforms import validators from my_app import db, app def get_ldap_connection(): conn = ldap3.initialize(app.config['LDAP_PROVIDER_URL']) return conn class User(db.Model): id = db.Column(db.Integer, primary_key=True) username = db.Column(db.String(100)) def __init__(self, username, password): self.username = username @staticmethod def try_login(username, password): conn = get_ldap_connection() conn.simple_bind_s( 'cn=%s,ou=mathematicians,dc=example,dc=com' % username, password ) def is_authenticated(self): return True def is_active(self): return True def is_anonymous(self): return False def get_id(self): return self.id class LoginForm(Form): username = StringField('Username', [validators.DataRequired()]) password = PasswordField('Password', [validators.DataRequired()])
Defines the User
model and LoginForm
, handling LDAP authentication and Flask-Login requirements.
flask_app/my_app/auth/views.py:
import ldap3 from flask import (request, render_template, flash, redirect, url_for, Blueprint, g) from flask_login import (current_user, login_user, logout_user, login_required) from my_app import login_manager, db auth = Blueprint('auth', __name__) from my_app.auth.models import User, LoginForm @login_manager.user_loader def load_user(id): return User.query.get(int(id)) @auth.before_request def get_current_user(): g.user = current_user @auth.route('/') @auth.route('/home') def home(): return render_template('home.html') @auth.route('/login', methods=['GET', 'POST']) def login(): if current_user.is_authenticated: flash('Already logged in.') return redirect(url_for('auth.home')) form = LoginForm(request.form) if request.method == 'POST' and form.validate(): username = request.form['username'] password = request.form['password'] try: User.try_login(username, password) except: flash('Invalid credentials.', 'danger') return render_template('login.html', form=form) user = User.query.filter_by(username=username).first() if not user: user = User(username=username, password=password) db.session.add(user) db.commit() login_user(user) flash('Login successful!', 'success') return redirect(url_for('auth.home')) if form.errors: flash(form.errors, 'danger') return render_template('login.html', form=form) @auth.route('/logout') @login_required def logout(): logout_user() return redirect(url_for('auth.home'))
Handles routing, login/logout logic, and user interaction.
flask_app/my_app/templates/base.html:
<!DOCTYPE html> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Flask LDAP Authentication</title> <link rel="stylesheet" href="https://www.php.cn/link/0bbfd30c6d7efe2fff86061e79c010db'static',%20filename='css/bootstrap.min.css')%20%7D%7D"> <link rel="stylesheet" href="https://www.php.cn/link/0bbfd30c6d7efe2fff86061e79c010db'static',%20filename='css/main.css')%20%7D%7D"> <nav class="navbar navbar-inverse navbar-fixed-top" role="navigation"> <div class="container"> <div class="navbar-header"> <a class="navbar-brand" href="https://www.php.cn/link/0bbfd30c6d7efe2fff86061e79c010db'auth.home')%20%7D%7D">Flask LDAP Demo</a> </div> </div> </nav> <div class="container"> <div> {% for category, message in get_flashed_messages(with_categories=true) %} <div class="alert alert-{{category}} alert-dismissable"> <button type="button" class="close" data-dismiss="alert" aria-hidden="true">×</button> {{ message }} </div> {% endfor %} </div> {% block container %}{% endblock %} </div> {% block scripts %}{% endblock %}
Base template for consistent layout.
flask_app/my_app/templates/home.html:
{% extends 'base.html' %} {% block container %} <h1 id="Flask-LDAP-Authentication-Demo">Flask LDAP Authentication Demo</h1> {% if current_user.is_authenticated %} <h3 id="Welcome-current-user-username">Welcome, {{ current_user.username }}!</h3> <a href="https://www.php.cn/link/0bbfd30c6d7efe2fff86061e79c010db'auth.logout')%20%7D%7D">Logout</a> {% else %} <a href="https://www.php.cn/link/0bbfd30c6d7efe2fff86061e79c010db'auth.login')%20%7D%7D">Login with LDAP</a> {% endif %} {% endblock %}
Home page content.
flask_app/my_app/templates/login.html:
{% extends 'base.html' %} {% block container %} <div class="top-pad"> <form method="POST" action="https://www.php.cn/link/0bbfd30c6d7efe2fff86061e79c010db'auth.login')%20%7D%7D" role="form"> {{ form.csrf_token }} <div class="form-group">{{ form.username.label }}: {{ form.username() }}</div> <div class="form-group">{{ form.password.label }}: {{ form.password() }}</div> <button type="submit" class="btn btn-primary">Submit</button> </form> </div> {% endblock %}
Login form.
flask_app/run.py:
from my_app import app app.run(debug=True)
Runs the application.
Running the Application:
Execute python run.py
and access https://www.php.cn/link/a7ef1270d275a7879bf01af8385dbd91. Test users: riemann, gauss, euler, euclid (password: password
).
This revised response provides a more structured and detailed explanation of the code, improving readability and understanding. The code itself is largely the same, but the presentation is significantly enhanced for clarity.
The above is the detailed content of Flask Authentication With LDAP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










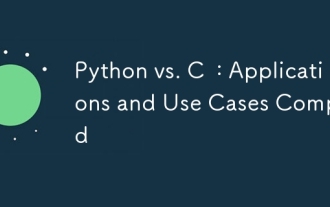
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
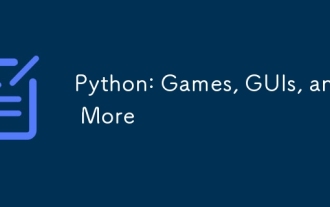
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
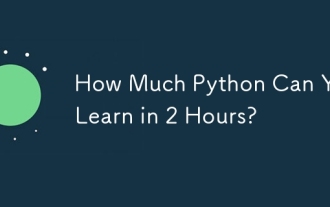
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
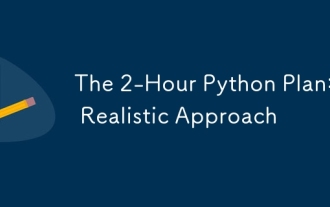
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
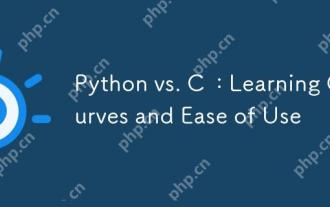
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
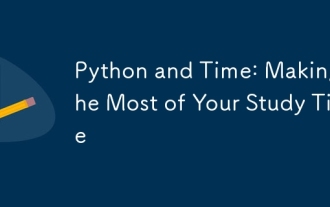
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
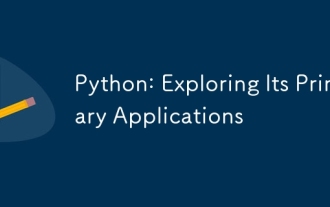
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
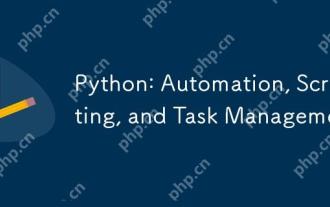
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
