Measuring JavaScript Functions' Performance
Web application performance is always crucial, especially in web development. Slow page loading speed will directly lead to user churn. As professional front-end developers, we must pay attention to performance optimization. Many traditional web performance optimization methods, such as reducing requests, using CDNs, and avoiding writing blocking renderings, are still valid today. However, as more and more web applications use JavaScript, validating code efficiency has become crucial.
Suppose you have a function that is functional but suspects it is not efficient and plan to make improvements. How to prove this assumption? What are the best practices for testing JavaScript function performance? Generally, the best way is to use the built-in performance.now()
function to measure the time before and after the function is executed. This article will discuss how to measure code execution time and tricks to avoid some common pitfalls.
Key Points
- Performance in web development is critical and can be improved by verifying code speed. The best way to test the performance of JavaScript functions is to use the built-in
performance.now()
function to measure the time before and after the function is executed.
The high resolution time API provides a function called - which returns an
now()
object. This provides an accurate description of the time passed, making it more accurate than the built-inDOMHighResTimeStamp
object.Date
When measuring the execution time of the function, be careful not to accidentally measure unimportant content. Make sure that the only operation to measure between two - is the execution of the function in question.
performance.now()
It is a common error to measure the function execution time only once. Execution time varies greatly based on various factors, so it is recommended to repeat the function and calculate the average or median time. - Context is important when comparing the speed of different functions. The speed of a function varies according to the specific task it performs. Therefore, in order to write faster web applications, it is important to make measurements and comparisons.
performance.now()The high resolution time API provides a function called
which returns an now()
object. This is a floating point number that reflects the current time in milliseconds, and is accurate to one thousandth of a millisecond. Individually, this number is not very valuable to your analysis, but the difference between two such numbers can accurately describe how much time has passed. In addition to being more precise than the built-in DOMHighResTimeStamp
object, it is also "monotonous". This means, simply put, it is not affected by the system (such as your laptop operating system) periodically correcting system time. To put it simply, defining two instances of and calculating the difference does not represent the elapsed time. The mathematical definition of "monotonic" is that
(for functions or quantities) changes in such a way that it either never decreases or never increases Date
. Another way to explain it is to imagine using it during a period of time during which the clock is toggling forward or backward. For example, when your country's clocks all agree to skip an hour to maximize the sunshine during the day. If you want to create an Date
instance one hour before the clock is dialed back, and then another instance afterwards, look at the difference, it will show something like "1 hour 3 seconds 123 milliseconds". Using the two instances of
, the difference will be "3 seconds 123 milliseconds and 456789 thousandths of a millisecond". In this section, I will not go into detail about this API. So if you want to learn more and see some examples of it using, I suggest you read the article Discovering the High Resolution Time API. Now that you've learned what the high-resolution time API is and how to use it, let's dive into some potential pitfalls. But before doing this, let's define a function called Date
, which we will use for the rest of this article. Date
performance.now()
makeHash()
The execution of this function can be measured as follows:
function makeHash(source) { var hash = 0; if (source.length === 0) return hash; for (var i = 0; i < source.length; i++) { var char = source.charCodeAt(i); hash = ((hash << 5) - hash) + char; hash = hash & hash; // Convert to 32bit integer } return hash; }
If you run this code in your browser, you should see something like the following:
var t0 = performance.now(); var result = makeHash('Peter'); var t1 = performance.now(); console.log('Took', (t1 - t0).toFixed(4), 'milliseconds to generate:', result);
The following shows a live demonstration of this code:
<code>Took 0.2730 milliseconds to generate: 77005292</code>
With this example in mind, let's start the discussion.
Trail #1 – Unimportant Content
In the example above, you can notice that between one
and another
, the only thing we do is call the function and assign its value to the variable performance.now()
. This gives us the time it takes to execute the function without anything else. This measurement can also be performed as follows: performance.now()
makeHash()
result
There is a live demonstration of this code snippet below:
var t0 = performance.now(); console.log(makeHash('Peter')); // 不好的主意! var t1 = performance.now(); console.log('Took', (t1 - t0).toFixed(4), 'milliseconds');
But in this case, we will measure the time it takes to call the function makeHash('Peter')
and the time it takes to send and print that output on the console. We don't know how long it takes for these two operations to take. You only know the combination time. Furthermore, the time required to send and print out varies greatly depending on what is happening in the browser or even in the browser at that time.
Maybe you know exactly how slow it is. However, executing multiple functions is equally wrong, even if each function does not involve any I/O. For example:
console.log
Similarly, we don't know how execution time is allocated. Is it a variable assignment, a
function makeHash(source) { var hash = 0; if (source.length === 0) return hash; for (var i = 0; i < source.length; i++) { var char = source.charCodeAt(i); hash = ((hash << 5) - hash) + char; hash = hash & hash; // Convert to 32bit integer } return hash; }
toLowerCase()
Trail #2 – Measure only oncetoString()
Compiler warm-up time (for example, the time when the code is compiled into bytecode)
- The main thread is busy dealing with other things we don't realize are going on
- Your computer's CPU(s) is busy with certain things that will slow down the entire browser
- A incremental improvement method is to execute the function repeatedly, as follows:
The following shows a live demonstration of this example:
var t0 = performance.now(); var result = makeHash('Peter'); var t1 = performance.now(); console.log('Took', (t1 - t0).toFixed(4), 'milliseconds to generate:', result);
The risk of this approach is that our browser JavaScript engine may perform secondary optimizations, which means that the second time the function is called with the same input, it can benefit from remembering the first output, And simply use it again. To solve this problem, you can use many different input strings instead of repeatedly sending the same input string (such as "Peter"). Obviously, the problem with testing with different inputs is that the function we are measuring naturally takes different time. Perhaps some inputs will result in longer execution time than others.
Trail #3 – Over-dependence on average
In the previous section, we learned that it is a good habit to repeat something and ideally use different inputs. However, we must remember that the problem with different inputs is that execution time may be much longer than all other inputs. So let's step back and send the same input. Suppose we send the same input ten times and print out the time it takes each time. The output may look like this:
function makeHash(source) {
var hash = 0;
if (source.length === 0) return hash;
for (var i = 0; i < source.length; i++) {
var char = source.charCodeAt(i);
hash = ((hash << 5) - hash) + char;
hash = hash & hash; // Convert to 32bit integer
}
return hash;
}
Copy after loginCopy after loginCopy after login
function makeHash(source) { var hash = 0; if (source.length === 0) return hash; for (var i = 0; i < source.length; i++) { var char = source.charCodeAt(i); hash = ((hash << 5) - hash) + char; hash = hash & hash; // Convert to 32bit integer } return hash; }
Note that the first time, the number is completely different from the other nine times. This is most likely because the JavaScript engine in our browser has done some optimizations and requires some warm-up. We can hardly avoid this, but we can consider some good remedies to prevent false conclusions. One way is to calculate the time average for the last nine times. Another more practical approach is to collect all the results and calculate the median. Basically, this is all the results in order and select the middle one. This is where performance.now()
is so useful, because you can get a number you can use as you like. Let's try again, but this time we will use a median function:
var t0 = performance.now(); var result = makeHash('Peter'); var t1 = performance.now(); console.log('Took', (t1 - t0).toFixed(4), 'milliseconds to generate:', result);
Trail #4 – Compare functions in predictable order
We have learned that it is always a good idea to measure something multiple times and average it. Furthermore, the last example tells us that it is better to use the median rather than the average. Now, in fact, a good use for measuring the execution time of a function is to understand which of several functions is faster. Suppose we have two functions that take the same type of input and produce the same result, but internally they work differently. Suppose we want a function that returns true or false if a string exists in an array of other strings, but this is case-insensitive. In other words, we cannot use Array.prototype.indexOf
because it is case-insensitive. This is an implementation like this:
<code>Took 0.2730 milliseconds to generate: 77005292</code>
We immediately noticed that this can be improved because the haystack.forEach
loop always traverses all elements, even if we have an early match. Let's try to write a better version using a good old for loop.
var t0 = performance.now(); console.log(makeHash('Peter')); // 不好的主意! var t1 = performance.now(); console.log('Took', (t1 - t0).toFixed(4), 'milliseconds');
Let's see which one is faster now. We do this by running each function 10 times and collecting all measurements:
var t0 = performance.now(); var name = 'Peter'; var result = makeHash(name.toLowerCase()).toString(); var t1 = performance.now(); console.log('Took', (t1 - t0).toFixed(4), 'milliseconds to generate:', result);
We run it and get the following output:
var t0 = performance.now(); for (var i = 0; i < 10; i++) { makeHash('Peter'); } var t1 = performance.now(); console.log('Took', ((t1 - t0) / 10).toFixed(4), 'milliseconds to generate');
The following shows a live demonstration of this example:
[The CodePen link should be inserted here, since I can't access external websites, it cannot be provided]
What exactly happened? The first function is three times faster. This shouldn't have happened!
The explanation is simple, but subtle. The first function using haystack.forEach
benefits from some low-level optimizations in the browser's JavaScript engine, and we don't get these optimizations when we use array indexing techniques. It proves our point: you will never know unless you measure it!
Conclusion
While we were trying to demonstrate how to get precise execution time in JavaScript using performance.now()
, we stumbled upon a benchmark scenario where our intuition was exactly the opposite of the conclusions we came from with our empirical results . The point is that if you want to write faster web applications, your JavaScript code needs to be optimized. Because computers are (almost) living things, they are unpredictable and surprising. The most reliable way to know that our code improvements will result in faster execution speeds is measurement and comparison. We never know which piece of code is faster, and if we have multiple ways to do the same thing, another reason is that context is important. In the previous section, we performed a case-insensitive string search, looking for a string in a 26 other strings. If we had to look for a string in 100,000 other strings, the conclusion would most likely be completely different. The list above is not exhaustive, as there are more pitfalls to be aware of. For example, measuring impractical scenarios or only taking measurements on a JavaScript engine. But it is certain that performance.now()
is a huge asset for JavaScript developers who want to write faster and better web applications. Last but not least, remember that measuring execution time only produces one dimension of "better code". Memory and code complexity also need to be considered. What about you? Have you ever used this function to test the performance of your code? If not, how do you proceed at this stage? Please share your thoughts in the comments below. Let's start the discussion!
(The FAQs part is omitted, because it is highly duplicated with the above content, just keep the key points.)
The above is the detailed content of Measuring JavaScript Functions' Performance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
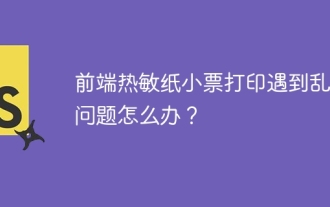
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
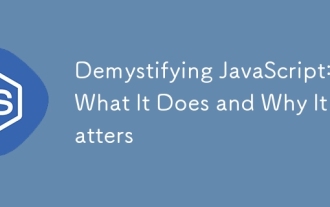
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
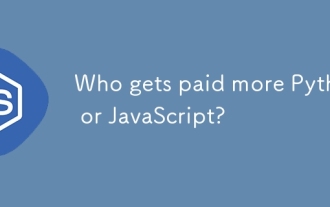
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
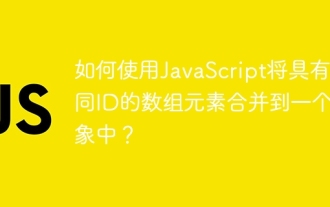
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
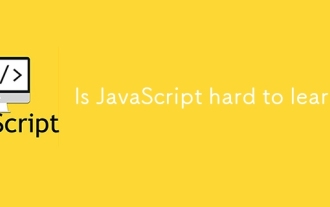
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
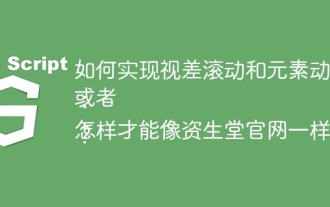
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
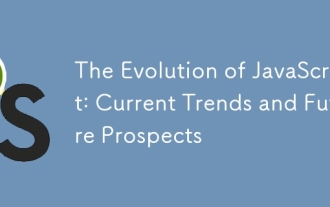
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
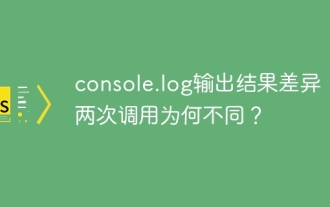
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
