CORS Misconfigurations in Laravel: Risks and Fixes
Cross-origin resource sharing (CORS) configuration errors in Laravel: risks and fixes
Cross-Origin Resource Sharing (CORS) is an important security mechanism that controls how external domains access resources on a web server. If configured incorrectly, it can expose your Laravel application to critical vulnerabilities that could lead to unauthorized data access or malicious attacks. In this article, we’ll explore what CORS configuration errors are, their impact, and how to fix them in Laravel.
We will also show how our Free Website Security Scanner tool can help you identify these vulnerabilities.
What is CORS? Why is it important?
CORS is a protocol that allows a server to define which domains can access its resources. It is typically implemented using the following HTTP headers:
- Access-Control-Allow-Origin
- Access-Control-Allow-Methods
- Access-Control-Allow-Headers
If configured correctly, CORS can prevent unauthorized external domains from making malicious requests to your web application. However, if configured incorrectly, it can allow unexpected access and compromise the security of your application.
Impact of CORS configuration errors
The following are potential risks of CORS misconfiguration:
- Data Breach: Malicious websites could access sensitive user data.
- Cross-site request forgery (CSRF): An attacker can perform actions on behalf of an authenticated user.
- Script injection: Exploiting JavaScript APIs to perform unauthorized actions.
Detecting CORS configuration errors in Laravel
You can manually check for CORS configuration errors using curl or browser developer tools. However, a faster and more effective way is to use our free website security checker tool.
Here is a screenshot of our tool interface:
Screenshot of the Free Tools web page where you can access the security assessment tool.
You can visit https://www.php.cn/link/82f82644bda7a260970fbd52a4c96528 to quickly scan your website and identify CORS issues.
Common CORS configuration errors in Laravel
Here are examples of common CORS configuration errors and how to fix them:
*Example 1: Allow all sources ()**
This is one of the most dangerous configuration errors:
<code>return [ 'paths' => ['*'], 'allowed_methods' => ['*'], 'allowed_origins' => ['*'], 'allowed_origins_patterns' => [], 'allowed_headers' => ['*'], 'exposed_headers' => [], 'max_age' => 0, 'supports_credentials' => false, ]; </code>
This configuration allows any domain to access your resources, putting your application at risk for CSRF and other attacks.
Fix: Limit sources and methods
Update your config/cors.php file to specify only trusted domains and methods:
<code>return [ 'paths' => ['*'], 'allowed_methods' => ['*'], 'allowed_origins' => ['*'], 'allowed_origins_patterns' => [], 'allowed_headers' => ['*'], 'exposed_headers' => [], 'max_age' => 0, 'supports_credentials' => false, ]; </code>
Test fix
After making these changes, test your CORS configuration using our Website Vulnerability Assessment Report (generated by our free tool) to check for website vulnerabilities. Here is a sample screenshot:
An example of a vulnerability assessment report generated by our free tool, providing insights into possible vulnerabilities.
This report highlights vulnerabilities (including CORS configuration errors) and recommends possible fixes.
Other tips for secure CORS configuration in Laravel
- *Avoid using the wildcard: **Always limit sources, headers and methods to specific values.
- Enable credential support only when necessary: Set supports_credentials to true only if your application requires it.
- Scan your application regularly: Use automated tools like ours to detect configuration errors and other vulnerabilities.
Conclusion
If unresolved, CORS configuration errors can pose significant risks to your Laravel application. By understanding common pitfalls and applying the correct configuration, you can protect your web applications from unauthorized access and potential attacks.
To make this process easier, take advantage of our free Website Security Checker tool. It provides comprehensive vulnerability reporting so you can quickly identify and fix CORS-related issues.
Stay proactive and secure your Laravel applications effectively!
The above is the detailed content of CORS Misconfigurations in Laravel: Risks and Fixes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
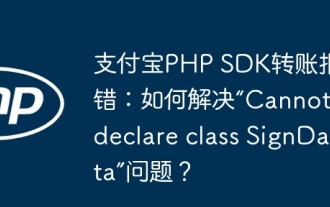
Alipay PHP...
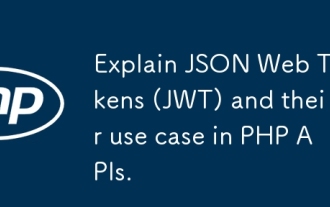
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
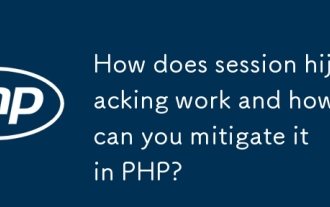
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
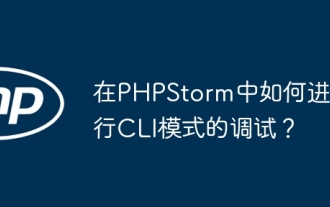
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
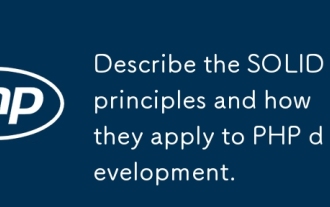
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
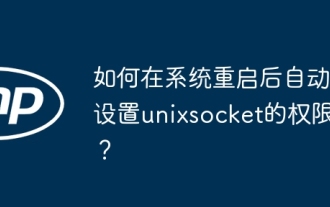
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
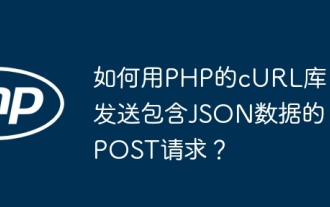
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
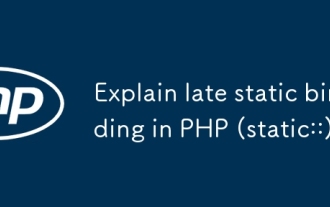
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
