Construct K Palindrome Strings
1400. Construct K Palindrome Strings
Difficulty: Medium
Topics: Hash Table, String, Greedy, Counting
Given a string s and an integer k, return true if you can use all the characters in s to construct k palindrome strings or false otherwise.
Example 1:
- Input: s = "annabelle", k = 2
- Output: true
-
Explanation: You can construct two palindromes using all characters in s.
- Some possible constructions "anna" "elble", "anbna" "elle", "anellena" "b"
Example 2:
- Input: s = "leetcode", k = 3
- Output: false
- Explanation: It is impossible to construct 3 palindromes using all the characters of s.
Example 3:
- Input: s = "true", k = 4
- Output: true
- Explanation: The only possible solution is to put each character in a separate string.
Constraints:
- 1 <= s.length <= 105
- s consists of lowercase English letters.
- 1 <= k <= 105
Hint:
- If the s.length < k we cannot construct k strings from s and answer is false.
- If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false.
- Otherwise you can construct exactly k palindrome strings and answer is true (why ?).
-
Palindrome Characteristics:
- A palindrome is a string that reads the same forwards and backwards.
- For an even-length palindrome, all characters must appear an even number of times.
- For an odd-length palindrome, all characters except one must appear an even number of times (the character that appears an odd number of times will be in the center).
-
Necessary Conditions:
- If the length of s is less than k, it's impossible to form k strings, so return false.
- The total number of characters that appear an odd number of times must be at most k to form k palindromes. This is because each palindrome can have at most one character with an odd count (the center character for odd-length palindromes).
- Count the frequency of each character in the string.
- Count how many characters have an odd frequency.
- If the number of odd frequencies exceeds k, return false (because it's impossible to form k palindromes).
- Frequency Count: We use an associative array $freq to count the occurrences of each character in the string.
- Odd Count: We check how many characters have odd occurrences. This will help us determine if we can form palindromes.
- Condition Check: If the number of characters with odd frequencies is greater than k, it's impossible to form k palindromes, so we return false. Otherwise, we return true.
- Counting the frequencies takes O(n), where n is the length of the string.
- Checking the odd frequencies takes O(m), where m is the number of distinct characters (at most 26 for lowercase English letters).
- The overall time complexity is O(n m), which simplifies to O(n) in this case.
- If k is greater than the length of s, we return false.
- If all characters have even frequencies, we can always form a palindrome, so the result depends on whether k is possible.
- GitHub
Solution:
We need to consider the following points:
Key Observations:
Approach:
Let's implement this solution in PHP: 1400. Construct K Palindrome Strings
<?php /** * @param String $s * @param Integer $k * @return Boolean */ function canConstruct($s, $k) { ... ... ... /** * go to ./solution.php */ } // Test cases var_dump(canConstruct("annabelle", 2)); // Output: true var_dump(canConstruct("leetcode", 3)); // Output: false var_dump(canConstruct("true", 4)); // Output: true ?>
Explanation:
Time Complexity:
Edge Cases:
Contact Links
If you found this series helpful, please consider giving the repository a star on GitHub or sharing the post on your favorite social networks ?. Your support would mean a lot to me!
If you want more helpful content like this, feel free to follow me:
The above is the detailed content of Construct K Palindrome Strings. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
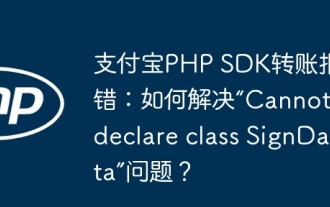
Alipay PHP...
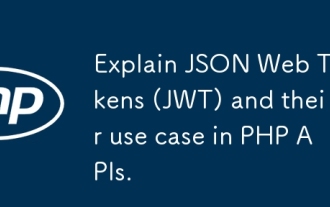
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
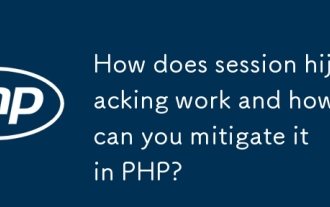
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
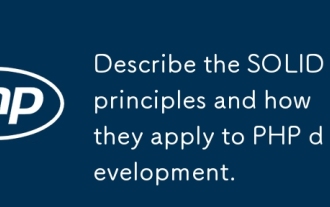
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
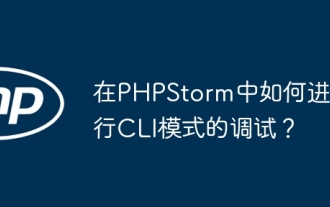
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
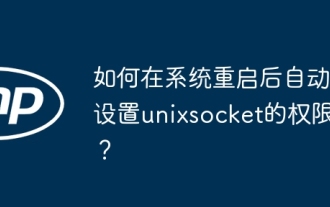
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
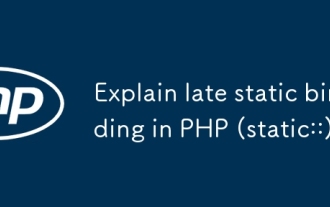
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
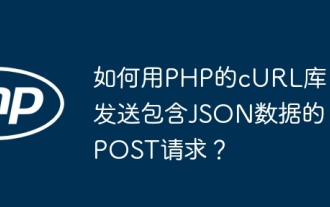
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
