


How Can I Pass an Array as Arguments to a Java Method with Variable Arguments?
Passing an Array as Arguments to a Method with Variable Arguments in Java
Problem
The goal is to create a Java method that takes an array of arguments and passes each individual argument to a method with variable arguments. However, the problem arises when the method signature declares an Object[] instead of separate arguments, leading to the array being treated as a single argument.
Solution
Yes, it is possible to pass an array as arguments to a method with variable arguments in Java. This is made possible by the syntactic sugar of T... which is equivalent to T[].
Code Example
The following example illustrates how to achieve this:
public static String ezFormat(Object... args) { String format = new String(new char[args.length]) .replace("", "[ %s ]"); return String.format(format, args); } public static void main(String... args) { System.out.println(ezFormat("A", "B", "C")); // prints "[ A ][ B ][ C ]" }
Varargs Gotchas
Passing Null
Be aware that passing null to a varargs parameter may result in unexpected behavior. To avoid errors, you can pass null as an array or as a single argument.
Adding Extra Arguments
When passing an array to a varargs parameter and adding extra arguments, it is necessary to create a new array that accommodates both the extra argument and the original array elements.
Passing Array of Primitives
Varargs only works with reference types. To pass an array of primitives, convert them to their corresponding wrapper classes (e.g., int[] to Integer[]).
The above is the detailed content of How Can I Pass an Array as Arguments to a Java Method with Variable Arguments?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
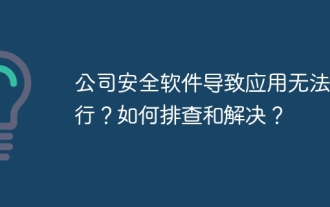
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
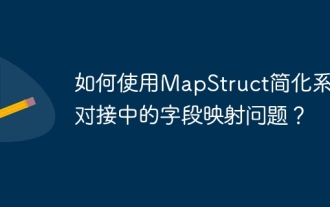
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
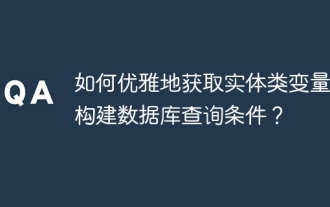
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
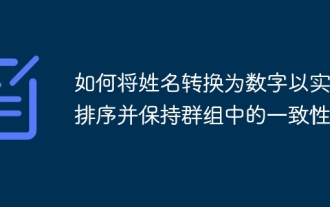
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
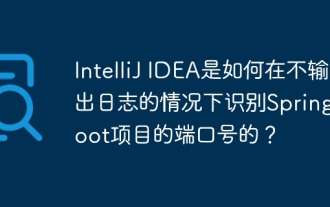
Start Spring using IntelliJIDEAUltimate version...
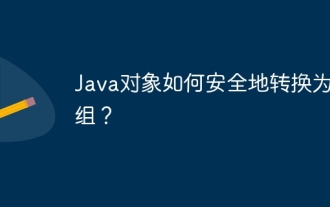
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
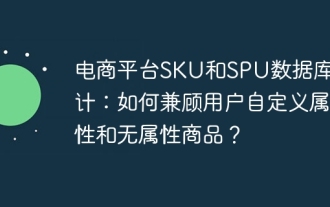
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
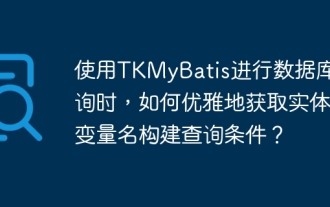
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
