How Can I Import CSV Data into a List of Tuples in Python?
Importing CSV Data into a List in Python
The task at hand is to import data from a CSV file into a list of tuples. A CSV file typically consists of rows of data separated by commas (,). In this case, each row represents a data point with two elements: a string and a category. The desired output is a list of tuples, where each tuple contains the string and the category from each row in the CSV file.
To accomplish this task in Python, we can utilize the widely used csv module. Consider the following steps:
- Open the CSV File: Open the CSV file for reading using the open() function, ensuring that newline='' is specified to avoid any line ending issues.
- Create a CSV Reader Object: Instantiating a csv.reader object with the opened file as the argument initializes a reader object.
- Read Rows into a List: Using the read() method of the reader object, read each row of data into a list. Each row is a list of values, where the first value represents the string, and the second value represents the category.
- Convert List of Lists to List of Tuples: If you wish to have a list of tuples instead of lists, you can employ list comprehension or the zip() function to achieve this transformation.
Here's an illustrative code snippet:
import csv with open('file.csv', newline='') as f: reader = csv.reader(f) data = list(reader) print(data) # [ # ['This is the first line', 'Line1'], # ['This is the second line', 'Line2'], # ['This is the third line', 'Line3'] # ]
This code will effectively import the CSV data into a list of tuples, meeting the desired output format.
The above is the detailed content of How Can I Import CSV Data into a List of Tuples in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
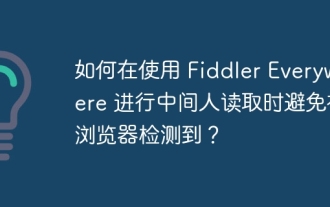
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
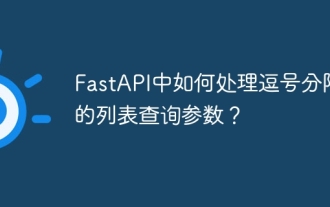
Fastapi ...
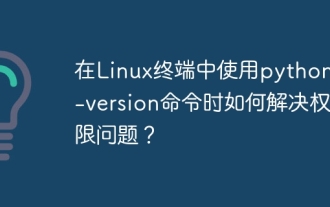
Using python in Linux terminal...
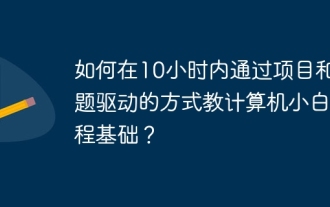
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
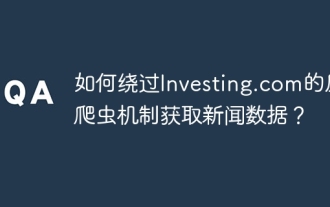
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
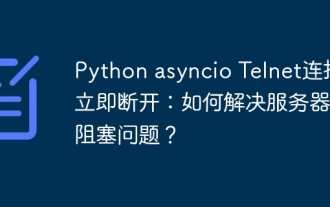
About Pythonasyncio...
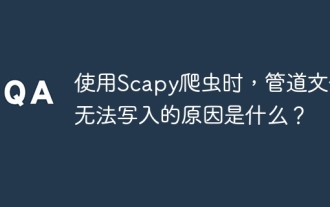
Discussion on the reasons why pipeline files cannot be written when using Scapy crawlers When learning and using Scapy crawlers for persistent data storage, you may encounter pipeline files...
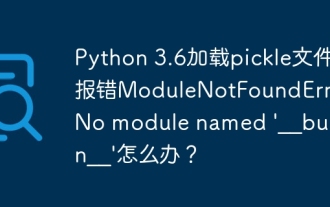
Loading pickle file in Python 3.6 environment error: ModuleNotFoundError:Nomodulenamed...
