JavaScript is like Python
This article presents a comparison between the syntax and fundamental programming constructs of JavaScript and Python. It aims to highlight similarities in how basic programming concepts are implemented across these two popular programming languages.
While both languages share many commonalities, making it easier for developers to switch between them or understand the other's code, there are also distinct syntactical and operational differences that one should be aware of.
It's important to approach this comparison with a light-hearted perspective and not to overemphasize the likeness or differences between JavaScript and Python. The intention is not to declare one language superior to the other but to provide a resource that can help coders who are familiar with Python to understand and transition to JavaScript more easily.
Hello World
JavaScript
// In codeguppy.com environment println('Hello, World'); // Outside codeguppy.com console.log('Hello, World');
Python
print('Hello, World')
Variables and Constants
JavaScript
let myVariable = 100; const MYCONSTANT = 3.14159;
Python
myVariable = 100 MYCONSTANT = 3.14159
String Interpolation
JavaScript
let a = 100; let b = 200; println(`Sum of ${a} and ${b} is ${a + b}`);
Python
a = 100 b = 200 print(f'Sum of {a} and {b} is {a + b}')
If Expression / Statement
JavaScript
let age = 18; if (age < 13) { println("Child"); } else if (age < 20) { println("Teenager"); } else { println("Adult"); }
Python
age = 18 if age < 13: print("Child") elif age < 20: print("Teenager") else: print("Adult")
Conditionals
JavaScript
let age = 20; let message = age >= 18 ? "Can vote" : "Cannot vote"; println(message); // Output: Can vote
Python
age = 20 message = "Can vote" if age >= 18 else "Cannot vote" print(message) # Output: Can vote
Arrays
JavaScript
// Creating an array let myArray = [1, 2, 3, 4, 5]; // Accessing elements println(myArray[0]); // Access the first element: 1 println(myArray[3]); // Access the fourth element: 4 // Modifying an element myArray[2] = 30; // Change the third element from 3 to 30 // Adding a new element myArray.push(6); // Add a new element to the end
Python
# Creating a list to represent an array my_array = [1, 2, 3, 4, 5] # Accessing elements print(my_array[0]) # Access the first element: 1 print(my_array[3]) # Access the fourth element: 4 # Modifying an element my_array[2] = 30 # Change the third element from 3 to 30 # Adding a new element my_array.append(6) # Add a new element to the end
ForEach
JavaScript
let fruits = ["apple", "banana", "cherry", "date"]; for(let fruit of fruits) println(fruit);
Python
fruits = ["apple", "banana", "cherry", "date"] for fruit in fruits: print(fruit)
Dictionaries
JavaScript
// Creating a dictionary fruit_prices = { apple: 0.65, banana: 0.35, cherry: 0.85 }; // Accessing a value by key println(fruit_prices["apple"]); // Output: 0.65
Python
# Creating a dictionary fruit_prices = { "apple": 0.65, "banana": 0.35, "cherry": 0.85 } # Accessing a value by key print(fruit_prices["apple"]) # Output: 0.65
Functions
JavaScript
function addNumbers(a, b) { return a + b; } let result = addNumbers(100, 200); println("The sum is: ", result);
Python
def add_numbers(a, b): return a + b result = add_numbers(100, 200) print("The sum is: ", result)
Tuple Return
JavaScript
function getCircleProperties(radius) { const area = Math.PI * radius ** 2; const circumference = 2 * Math.PI * radius; return [area, circumference]; // Return as an array } // Using the function const [area, circumference] = getCircleProperties(5); println(`The area of the circle is: ${area}`); println(`The circumference of the circle is: ${circumference}`);
Python
import math def getCircleProperties(radius): """Calculate and return the area and circumference of a circle.""" area = math.pi * radius**2 circumference = 2 * math.pi * radius return (area, circumference) # Using the function radius = 5 area, circumference = getCircleProperties(radius) print(f"The area of the circle is: {area}") print(f"The circumference of the circle is: {circumference}")
Variable number of arguments
JavaScript
function sumNumbers(...args) { let sum = 0; for(let i of args) sum += i; return sum; } println(sumNumbers(1, 2, 3)); println(sumNumbers(100, 200));
Python
def sum_numbers(*args): sum = 0 for i in args: sum += i return sum print(sum_numbers(1, 2, 3)) print(sum_numbers(100, 200))
Lambdas
JavaScript
const numbers = [1, 2, 3, 4, 5]; // Use map to apply a function to all elements of the array const squaredNumbers = numbers.map(x => x ** 2); println(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Python
numbers = [1, 2, 3, 4, 5] # Use map to apply a function to all elements of the list squared_numbers = map(lambda x: x**2, numbers) # Convert map object to a list to print the results squared_numbers_list = list(squared_numbers) print(squared_numbers_list) # Output: [1, 4, 9, 16, 25]
Classes
JavaScript
class Book { constructor(title, author, pages) { this.title = title; this.author = author; this.pages = pages; } describeBook() { println(`Book Title: ${this.title}`); println(`Author: ${this.author}`); println(`Number of Pages: ${this.pages}`); } }
Python
class Book: def __init__(self, title, author, pages): self.title = title self.author = author self.pages = pages def describe_book(self): print(f"Book Title: {self.title}") print(f"Author: {self.author}") print(f"Number of Pages: {self.pages}")
Usage of classes
JavaScript
// In codeguppy.com environment println('Hello, World'); // Outside codeguppy.com console.log('Hello, World');
Python
print('Hello, World')
Conclusion
We encourage you to get involved in refining this comparison. Your contributions, whether they are corrections, enhancements, or new additions, are highly valued. By collaborating, we can create a more accurate and comprehensive guide that benefits all developers interested in learning about JavaScript and Python.
Credits
This article was republished from the blog of the free coding platform https://codeguppy.com platform.
The article was influenced by similar comparisons between other programming languages:
- Kotlin is like C# https://ttu.github.io/kotlin-is-like-csharp/
- Kotlin is like TypeScript https://gi-no.github.io/kotlin-is-like-typescript/
- Swift is like Kotlin https://nilhcem.com/swift-is-like-kotlin/
- Swift is like Go http://repo.tiye.me/jiyinyiyong/swift-is-like-go/
- Swift is like Scala https://leverich.github.io/swiftislikescala/
The above is the detailed content of JavaScript is like Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
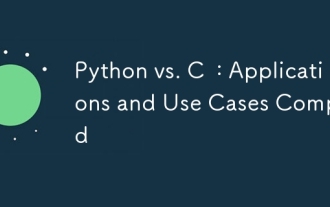
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
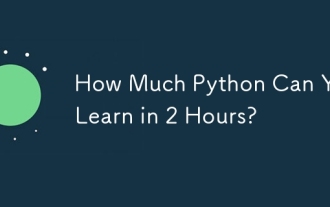
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
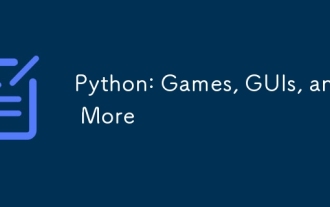
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
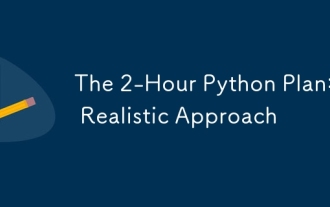
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
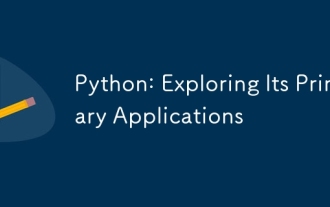
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
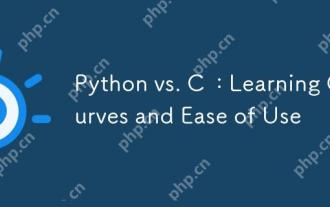
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
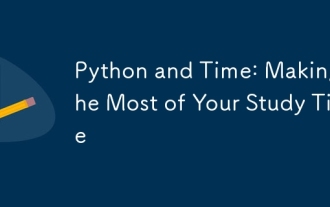
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
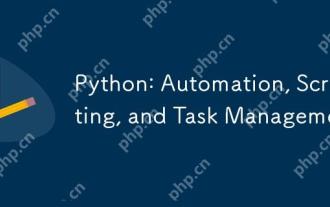
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
