


How to Efficiently Spawn Multiple Object Instances Concurrently in Python?
Spawning Multiple Instances of the Same Object Concurrently in Python
In programming, it is often necessary to create multiple instances of the same object simultaneously. This can be particularly useful in game development, where developers may need to create multiple enemies or projectiles on the screen at the same time.
Initially, beginner programmers may attempt to use functions like sleep() to control the timing of these object spawns. However, this approach can lead to issues where the previously spawned objects are overwritten by subsequent ones.
To address this issue, a more effective approach is to measure the time elapsed in the game loop and create objects based on the elapsed time. This ensures that objects are spawned at the appropriate intervals and do not overwrite one another.
Option 1: Using pygame.time.get_ticks()
One method for measuring elapsed time is to use the pygame.time.get_ticks() function. This function returns the number of milliseconds that have passed since pygame was initialized.
object_list = [] time_interval = 500 # 500 milliseconds == 0.1 seconds next_object_time = 0 while run: # [...] current_time = pygame.time.get_ticks() if current_time > next_object_time: next_object_time += time_interval object_list.append(Object())
In this example, a list of objects, object_list, is kept track of. The time_interval variable determines the time between each object spawn. The next_object_time variable keeps track of the time at which the next object should be spawned.
When the current time exceeds the next object time, a new object is appended to the object_list, and the next object time is updated.
Option 2: Using the Pygame Event Queue
Another option for spawning objects at regular intervals is to use the Pygame event queue. This approach involves creating a custom event and using the pygame.time.set_timer() function to repeatedly add the event to the queue.
object_list = [] time_interval = 500 # 500 milliseconds == 0.1 seconds timer_event = pygame.USEREVENT+1 pygame.time.set_timer(timer_event, time_interval)
In this example, a custom event, timer_event, is created. The time_interval variable determines the time between each event. The pygame.time.set_timer() function is used to add the timer_event to the event queue at the specified time interval.
When the timer_event occurs, a new object is added to the object_list. This approach ensures that objects are spawned at regular intervals, even if the game loop experiences slowdowns or interruptions.
Conclusion
By understanding the limitations of using functions like sleep() and adopting more advanced techniques like measuring elapsed time or using the event queue, programmers can effectively spawn multiple instances of the same object concurrently in Python. This approach is essential for creating complex and engaging games or other applications that require the simultaneous management of multiple objects.
The above is the detailed content of How to Efficiently Spawn Multiple Object Instances Concurrently in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










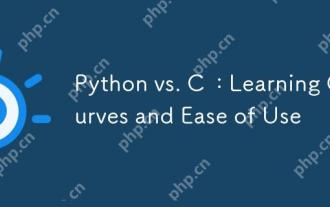
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
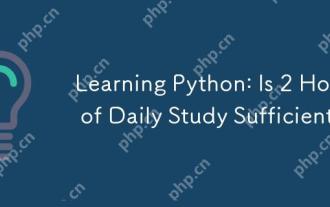
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
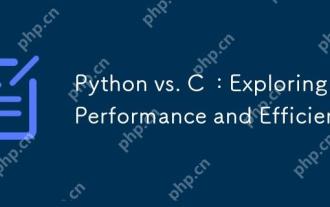
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
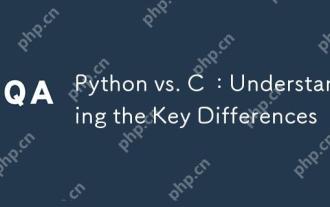
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
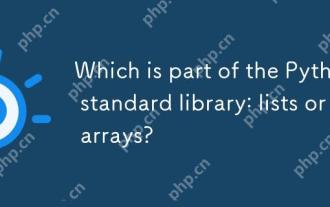
Pythonlistsarepartofthestandardlibrary,whilearraysarenot.Listsarebuilt-in,versatile,andusedforstoringcollections,whereasarraysareprovidedbythearraymoduleandlesscommonlyusedduetolimitedfunctionality.
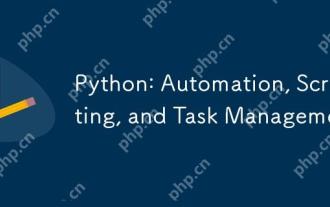
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
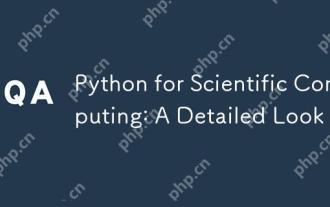
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
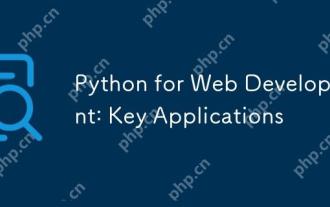
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
