Easy way to find the Time Complexity of an Algorithm
Time complexity is considered one of the toughest topics for beginners who are just starting with Problem-Solving. Here, I am providing the time complexity analysis cheat sheet. I hope this helps. Please let me know if you have any questions.
Time Complexity Analysis Cheatsheet
Quick Reference Table
O(1) - Constant time O(log n) - Logarithmic (halving/doubling) O(n) - Linear (single loop) O(n log n) - Linearithmic (efficient sorting) O(n²) - Quadratic (nested loops) O(2ⁿ) - Exponential (recursive doubling) O(n!) - Factorial (permutations)
Identifying Patterns
1. O(1) - Constant
# Look for: - Direct array access - Basic math operations - Fixed loops - Hash table lookups # Examples: arr[0] x + y for i in range(5) hashmap[key]
2. O(log n) - Logarithmic
# Look for: - Halving/Doubling - Binary search patterns - Tree traversal by level # Examples: while n > 0: n = n // 2 left, right = 0, len(arr)-1 while left <= right: mid = (left + right) // 2
3. O(n) - Linear
# Look for: - Single loops - Array traversal - Linear search - Hash table building # Examples: for num in nums: # O(1) operation total += num for i in range(n): # O(1) operation arr[i] = i
4. O(n log n) - Linearithmic
# Look for: - Efficient sorting - Divide and conquer - Tree operations with traversal # Examples: nums.sort() sorted(nums) merge_sort(nums)
5. O(n²) - Quadratic
# Look for: - Nested loops - Simple sorting - Matrix traversal - Comparing all pairs # Examples: for i in range(n): for j in range(n): # O(1) operation # Pattern finding for i in range(n): for j in range(i+1, n): # Compare pairs
6. O(2ⁿ) - Exponential
# Look for: - Double recursion - Power set - Fibonacci recursive - All subsets # Examples: def fib(n): if n <= 1: return n return fib(n-1) + fib(n-2) def subsets(nums): if not nums: return [[]] result = subsets(nums[1:]) return result + [nums[0:1] + r for r in result]
Common Operations Time Complexity
Array/List Operations
# O(1) arr[i] # Access arr.append(x) # Add end arr.pop() # Remove end # O(n) arr.insert(i, x) # Insert middle arr.remove(x) # Remove by value arr.index(x) # Find index min(arr), max(arr) # Find min/max
Dictionary/Set Operations
# O(1) average d[key] # Access d[key] = value # Insert key in d # Check existence d.get(key) # Get value # O(n) len(d) # Size d.keys() # Get keys d.values() # Get values
String Operations
# O(n) s + t # Concatenation s.find(t) # Substring search s.replace(old, new) # Replace ''.join(list) # Join # O(n²) potential s += char # Repeated concatenation
Loop Analysis
Single Loop
# O(n) for i in range(n): # O(1) operations # O(n/2) = O(n) for i in range(0, n, 2): # Skip elements still O(n)
Nested Loops
# O(n²) for i in range(n): for j in range(n): # O(1) operations # O(n * m) for i in range(n): for j in range(m): # Different sizes # O(n²/2) = O(n²) for i in range(n): for j in range(i, n): # Triangular still O(n²)
Multiple Loops
# O(n + m) for i in range(n): # O(1) for j in range(m): # O(1) # O(n + n²) = O(n²) for i in range(n): # O(1) for i in range(n): for j in range(n): # O(1)
Recursive Analysis
Linear Recursion
# O(n) def factorial(n): if n <= 1: return 1 return n * factorial(n-1)
Binary Recursion
# O(2ⁿ) def fibonacci(n): if n <= 1: return n return fibonacci(n-1) + fibonacci(n-2)
Divide & Conquer
# O(n log n) def mergeSort(arr): if len(arr) <= 1: return arr mid = len(arr) // 2 left = mergeSort(arr[:mid]) right = mergeSort(arr[mid:]) return merge(left, right)
Optimization Red Flags
Hidden Loops
# String operations for c in string: newStr += c # O(n²) # List comprehension [x for x in range(n) for y in range(n)] # O(n²)
Built-in Functions
len() # O(1) min(), max() # O(n) sorted() # O(n log n) list.index() # O(n)
Tips for Analysis
- Count nested loops
- Check recursive branching
- Consider hidden operations
- Look for divide & conquer
- Check built-in function complexity
- Consider average vs worst case
- Watch for loop variables
- Consider input constraints
Thank you for reading, please give the thumbs up on the post if you found this helpful. Cheers!
The above is the detailed content of Easy way to find the Time Complexity of an Algorithm. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
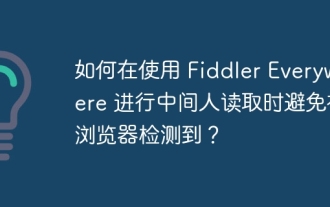
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
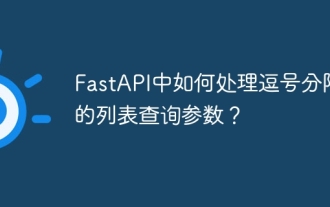
Fastapi ...
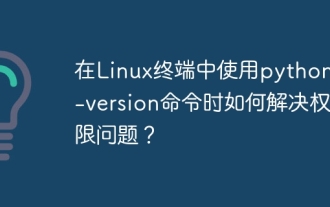
Using python in Linux terminal...
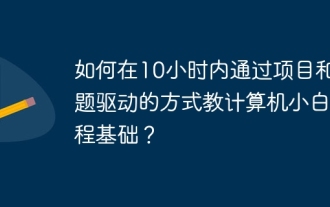
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
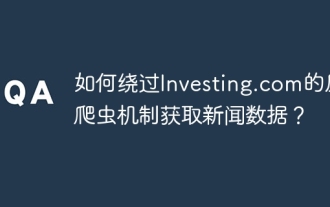
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
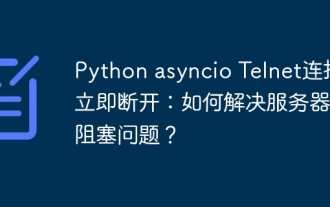
About Pythonasyncio...
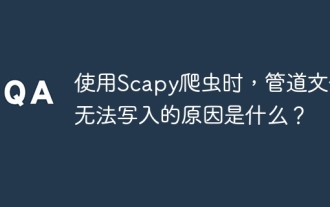
Discussion on the reasons why pipeline files cannot be written when using Scapy crawlers When learning and using Scapy crawlers for persistent data storage, you may encounter pipeline files...
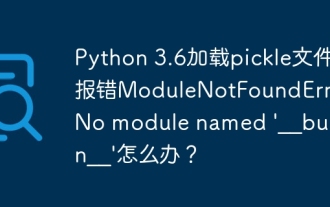
Loading pickle file in Python 3.6 environment error: ModuleNotFoundError:Nomodulenamed...
