


How to convert JSON strings to Python dictionaries using json.loads()?
Converting JSON Strings to Dictionaries in Python
In Python, JavaScript Object Notation (JSON) data is frequently encountered as hierarchical structures within strings. To work with JSON data effectively, you may encounter the need to convert a JSON string into a Python dictionary, which is a native Python data structure.
Using json.loads()
The standard Python library provides the json module, which houses the function json.loads(). This function takes a JSON string and parses it into a Python object, typically a dictionary. For instance:
<code class="python">import json json_string = '{"name": "John Doe", "age": 30}' python_dict = json.loads(json_string)</code>
After executing this code, python_dict becomes a dictionary with two key-value pairs: "name" maps to "John Doe" and "age" maps to 30.
Case Study: Extracting Dictionary Values
Your example JSON string is a nested dictionary with a complex structure. To convert it into a dictionary and access its values, follow these steps:
<code class="python">import json json_string = '...' # Your JSON string here # Parse the JSON string json_dict = json.loads(json_string) # Retrieve the "title" value from the outermost dictionary title = json_dict['glossary']['title'] print(title) # Output: "example glossary"</code>
In this example, json_dict becomes a nested dictionary after parsing the JSON string. You can then use dictionary access notation to navigate through the levels and retrieve the desired value, assigned to the title variable.
Additional Considerations
- Double-check the syntax of your JSON string for validity.
- The json.loads() function does not validate the JSON data; it assumes it's well-formed.
- You can also convert Python dictionaries to JSON strings using json.dumps().
The above is the detailed content of How to convert JSON strings to Python dictionaries using json.loads()?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










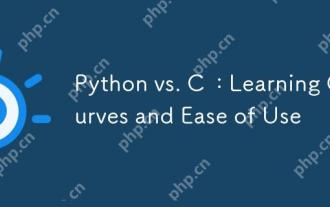
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
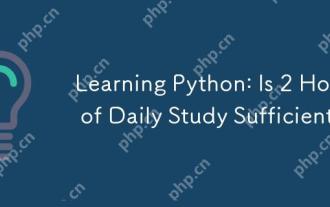
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
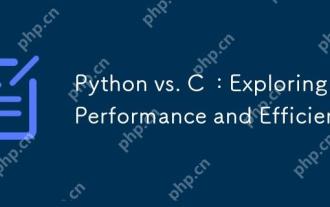
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
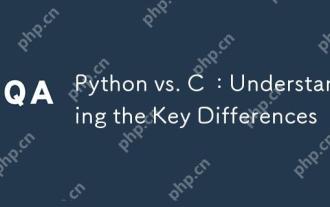
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
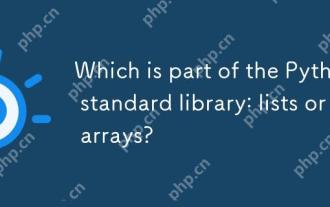
Pythonlistsarepartofthestandardlibrary,whilearraysarenot.Listsarebuilt-in,versatile,andusedforstoringcollections,whereasarraysareprovidedbythearraymoduleandlesscommonlyusedduetolimitedfunctionality.
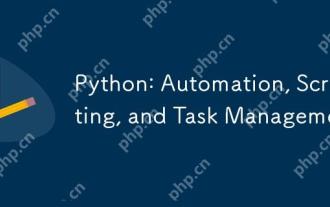
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
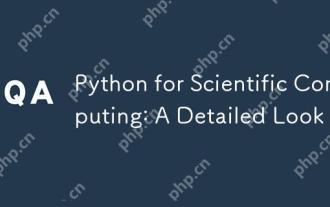
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
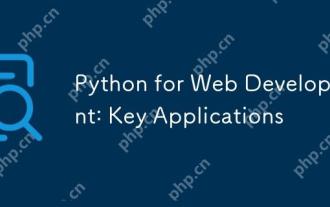
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
