


How to Safely Encrypt and Decrypt Strings Using Passwords in Python?
Securely Encrypting and Decrypting Strings with Passwords in Python
Python's cryptography library is a comprehensive toolkit for encrypting and decrypting data. To encrypt strings using a password, you can leverage the Fernet class, which provides robust encryption and includes essential features such as a timestamp, HMAC signature, and base64 encoding.
Fernet with Password
<code class="python">from cryptography.fernet import Fernet, FernetException password = 'mypass' fernet = Fernet(password.encode()) encrypted_message = fernet.encrypt(b'John Doe') decrypted_message = fernet.decrypt(encrypted_message) print(encrypted_message) # Encrypted string print(decrypted_message.decode()) # 'John Doe'</code>
Fernet keeps encrypted data safe by applying multiple layers of encryption and ensuring message integrity with an HMAC signature.
Password-Derived Key Generation for Fernet
While using a password directly with Fernet is convenient, it's more secure to generate a key using a password. This approach involves deriving a secret key from the password and salt using a key derivation function.
<code class="python">import secrets from cryptography.fernet import Fernet from cryptography.hazmat.backends import default_backend from cryptography.hazmat.primitives import hashes from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC backend = default_backend() salt = secrets.token_bytes(16) # Generate a unique salt password = 'mypass'.encode() # Convert password to bytes kdf = PBKDF2HMAC( algorithm=hashes.SHA256(), length=32, salt=salt, iterations=100000, backend=backend ) key = b64e(kdf.derive(password)) # Derive the secret key fernet = Fernet(key) encrypted_message = fernet.encrypt(b'John Doe')</code>
This method enhances security by adding an additional layer of protection to the encryption process with a strong key derived from your password and a unique salt.
Other Encryption Approaches
Beyond Fernet, you may consider alternatives depending on your specific requirements:
Base64 Obscuring: For basic obfuscation, base64 encoding can be used without encryption. However, this doesn't provide any actual security, just obscurity.
HMAC Signature: If your goal is data integrity, use HMAC signatures to ensure the data hasn't been tampered with.
AES-GCM Encryption: AES-GCM uses Galois/Counter Mode block encryption to provide both encryption and integrity guarantees, similar to Fernet but without its user-friendly features.
The above is the detailed content of How to Safely Encrypt and Decrypt Strings Using Passwords in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
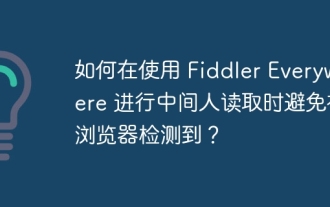
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
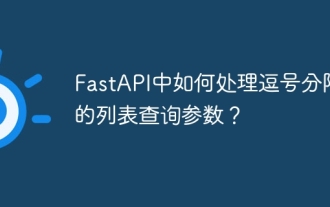
Fastapi ...
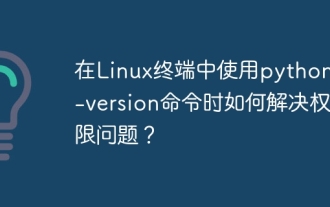
Using python in Linux terminal...
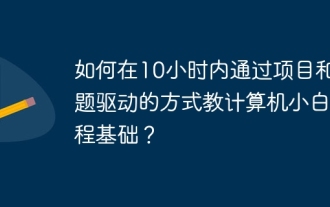
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
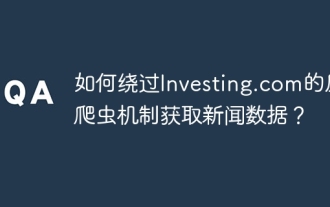
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
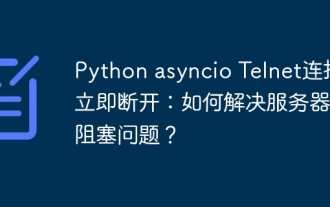
About Pythonasyncio...
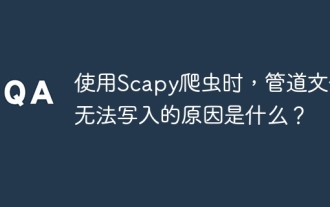
Discussion on the reasons why pipeline files cannot be written when using Scapy crawlers When learning and using Scapy crawlers for persistent data storage, you may encounter pipeline files...
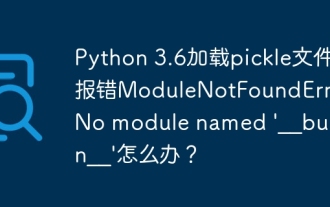
Loading pickle file in Python 3.6 environment error: ModuleNotFoundError:Nomodulenamed...
