


Integration of data access layer design and domain-driven design in Java framework
Integrating the Data Access Layer (DAL) in the Java framework with Domain Driven Design (DDD) creates a robust and scalable data access layer. The integration process involves: defining the domain model to represent entities in the business domain; creating a DAO repository to encapsulate the data access operations of a specific aggregate; using query methods, using Java 8 lambda or method references to specify query conditions; processing transactions, using @Transactional Annotations mark methods to indicate that they should be executed within a transaction.
The integration of data access layer design and domain-driven design in the Java framework
In the Java framework, the data access layer (DAL ) is responsible for handling the interaction between the application and the database. Domain-driven design (DDD) is a software design paradigm that emphasizes building systems using domain concepts to improve code maintainability and understandability.
Merge DAL and DDD to create a robust and scalable data access layer that can be seamlessly integrated with business logic.
Practical case: Using Spring Data JPA and Spring Boot
Spring Data JPA is a library in the Spring framework used to interact with JPA (Java Persistence API). It provides an abstraction layer that simplifies data access. Spring Boot is a simplified development toolkit built on the Spring framework.
To apply DDD concepts to the Spring Data JPA data access layer, you can follow the following steps:
1. Define the domain model
The domain model is Core concepts of DDD. It represents entities, value objects, and aggregates in the business domain. In Spring Data JPA, these concepts can be represented using JPA entities.
2. Create a DAO repository
Create a DAO (Data Access Object) repository to encapsulate aggregate-specific data access operations. Spring Data JPA provides annotations for creating repositories such as @Repository
and @PersistenceContext
.
3. Use the query method
You can use the query method of Spring Data JPA to query and modify the database. These methods use lambda expressions or method references in Java 8 to specify query criteria.
4. Processing Transactions
Use the @Transactional
annotation to mark methods to indicate that they should be executed within a transaction. Spring Boot handles transaction management, providing automatic rollback and commit capabilities.
Sample code:
// 定义实体 @Entity public class Customer { @Id @GeneratedValue private Long id; private String name; private String address; } // 定义存储库 public interface CustomerRepository extends JpaRepository<Customer, Long> {} // 使用查询方法 public List<Customer> findByName(String name); // 使用事务 @Transactional public void save(Customer customer);
By following the above steps, you can design a data access layer that incorporates DAL and DDD principles to improve the robustness of your application. Maintainability and scalability.
The above is the detailed content of Integration of data access layer design and domain-driven design in Java framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










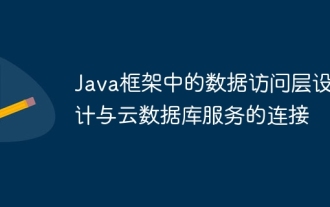
The data access layer in the Java framework is responsible for the interaction between the application and the database. To ensure reliability, DAO should follow the principles of single responsibility, loose coupling and testability. The performance and availability of Java applications can be enhanced by leveraging cloud database services such as Google Cloud SQL or Amazon RDS. Connecting to a cloud database service involves using a dedicated JDBC connector and socket factory to securely interact with the managed database. Practical cases show how to use JDBC or ORM framework to implement common CRUD operations in Java framework.
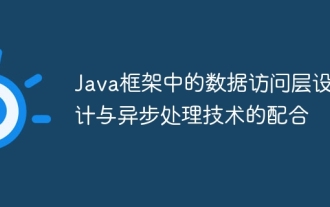
Combined with data access layer (DAO) design and asynchronous processing technology, application performance can be effectively improved in the Java framework. DAO is responsible for handling interactions with the database and follows the single responsibility principle; asynchronous processing technologies such as thread pools, CompletableFuture and ReactorPattern can avoid blocking the main thread. Combining the two, such as finding the user asynchronously via a CompletableFuture, allows the application to perform other tasks simultaneously, thus improving response times. Practical cases show the specific steps of using SpringBoot, JPA and CompletableFuture to implement an asynchronous data access layer for developers to refer to to improve application performance.
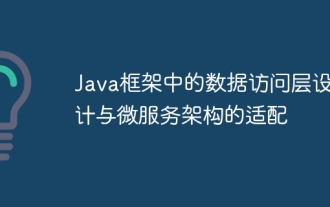
In order to implement the data access layer in the microservice architecture, you can follow the DDD principle and separate domain objects from data access logic. By adopting a service-oriented architecture, DAL can provide API services through standard protocols such as REST or gRPC, enabling reusability and observability. Taking SpringDataJPA as an example, you can create a service-oriented DAL and use JPA-compatible methods (such as findAll() and save()) to operate on data, thereby improving the scalability and flexibility of the application.
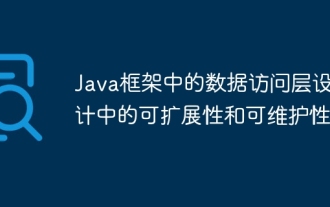
Following the principles of scalability and maintainability, the Java framework data access layer can achieve: Scalability: Abstract data access layer: Separate logic and database implementation Support multiple databases: Respond to changes in requirements Use a connection pool: Manage connections to prevent exhaustion Maintainability: Clear naming convention: Improves readability Separation of queries and code: Enhances clarity and maintainability Use logging: Eases debugging and tracing system behavior
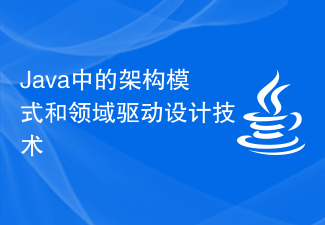
Java is one of the most widely used programming languages. It not only has object-oriented features, but also provides many powerful architectural patterns and design technologies. Among them, Domain-driven Design (DDD) is a very popular technology. , and has a wide range of applications in actual development. This article will introduce some common Java architectural patterns and domain-driven design techniques. 1. Architecture pattern MVC (Model-View-Controller)M
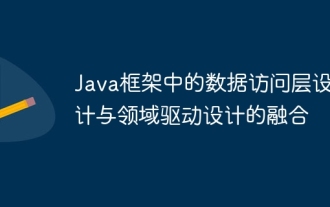
Integrating the Data Access Layer (DAL) in the Java framework with Domain Driven Design (DDD) can create a robust and scalable data access layer. The integration process involves: defining the domain model to represent entities in the business domain; creating a DAO repository to encapsulate the data access operations of specific aggregates; using query methods, using Java8lambda or method references to specify query conditions; processing transactions, using @Transactional annotation tags methods to indicate that they should be executed within a transaction.
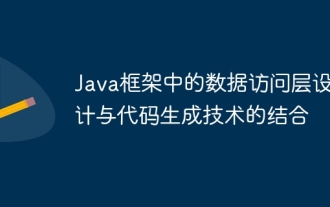
Combining data access layer design and code generation technology, Java developers can create a maintainable, scalable and consistent data access layer (DAL). The following steps illustrate practical cases of SpringBoot and MyBatisGenerator: Install the MyBatisGenerator plug-in. Create a model package to store entity classes. Create a mapper package to store MyBatis mapping files. Run the MyBatisGenerator command to generate DAL. Configure MyBatis mapper in SpringBoot application.
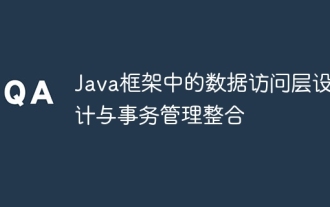
How to design Data Access Layer (DAL) in Java framework and integrate it with transaction management? 1. Follow DAL design principles: loose coupling, interface-driven, and reusable. 2. Use SpringDataJPA to simplify access to the database. 3. Use SpringTransactional annotations to manage transactions and ensure data integrity.
