


PHP data structure: exploration of tree structure, mastering the organization of hierarchical data
Tree structure is a non-linear structure that organizes data hierarchically, and can be represented and traversed recursively or iteratively in PHP. Representation methods include recursion (using class) and iteration (using array); traversal methods include recursive traversal and iterative traversal (using stack). In the actual case, the file system directory tree is efficiently organized using a tree structure to facilitate browsing and obtaining information.
PHP tree structure exploration: a powerful tool for hierarchical data organization
The tree structure is a non-linear data structure. Organizing data in a hierarchical manner is very suitable for data that needs to express hierarchical relationships. In PHP, tree structures can be represented and traversed using either recursion or iteration.
Representing a tree structure
There are two main ways to represent a tree structure in PHP:
Recursive representation:
class Node { public $value; public $children = []; public function __construct($value) { $this->value = $value; } public function addChild(Node $child) { $this->children[] = $child; } }
Iterative representation (using array):
$tree = [ 'value' => 'Root', 'children' => [ [ 'value' => 'Child 1', 'children' => [] ], [ 'value' => 'Child 2', 'children' => [ 'value' => 'Grandchild' ] ] ] ];
Traversing the tree structure
The following two methods can be used Traverse the tree structure:
Recursive traversal:
function traverseRecursively($node) { echo $node->value . PHP_EOL; foreach ($node->children as $child) { traverseRecursively($child); } }
Iterative traversal (using stack):
function traverseIteratively($node) { $stack = [$node]; while (!empty($stack)) { $current = array_pop($stack); echo $current->value . PHP_EOL; foreach (array_reverse($current->children) as $child) { $stack[] = $child; } } }
Practical case: File system directory tree
Consider a file system directory tree, where each directory contains subdirectories and files. This data structure can be organized and represented efficiently using a tree structure.
class Directory { public $name; public $children = []; public function __construct($name) { $this->name = $name; } public function addChild(Node $child) { $this->children[] = $child; } } $root = new Directory('/'); $dir1 = new Directory('dir1'); $dir2 = new Directory('dir2'); $dir3 = new Directory('dir3'); $file1 = new File('file1.txt'); $file2 = new File('file2.php'); $dir1->addChild($file1); $dir2->addChild($file2); $root->addChild($dir1); $root->addChild($dir2); $root->addChild($dir3); traverseRecursively($root);
By using the tree structure, we can easily browse and organize the file system directory tree and obtain the required information efficiently.
The above is the detailed content of PHP data structure: exploration of tree structure, mastering the organization of hierarchical data. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
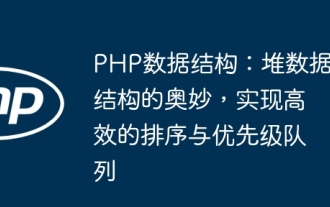
The heap data structure in PHP is a tree structure that satisfies the complete binary tree and heap properties (the parent node value is greater/less than the child node value), and is implemented using an array. The heap supports two operations: sorting (extracting the largest element from small to large) and priority queue (extracting the largest element according to priority). The properties of the heap are maintained through the heapifyUp and heapifyDown methods respectively.
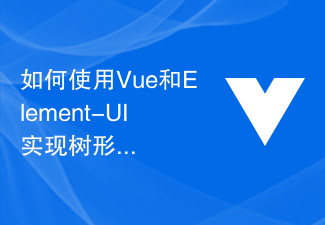
How to use Vue and Element-UI to implement tree-structured data display Introduction: In modern web applications, tree-structured data display is a very common requirement. As a very popular front-end framework, Vue.js, combined with Element-UI, a powerful UI library, can easily realize tree-structured data display. This article will introduce how to use Vue and Element-UI to implement this function, and provide code examples for readers' reference. 1. Preliminary knowledge: Starting to use Vue and El
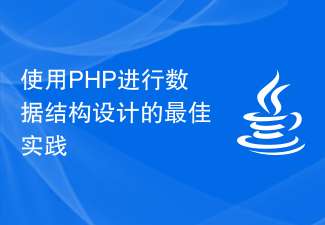
As one of the most widely used programming languages, PHP also has its own advantages and best practices when designing data structures. When designing data structures, PHP developers need to consider some key factors, including data type, performance, code readability, and reusability. The following will introduce the best practices for data structure design using PHP. Selection of data types Data types are one of the key factors in data structure design because they affect program performance, memory usage, and code readability. In PHP, there is
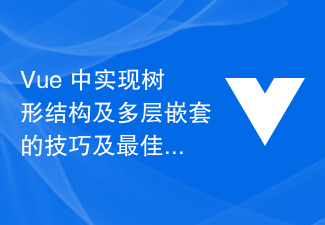
Vue is a popular JavaScript framework that provides many convenient tools and features for developing dynamic applications. One common function is to display tree-structured data. In this article, we will explore how to implement tree structures and multi-level nesting in Vue, and share best practices. Tree Structure A tree structure is a hierarchical structure that consists of parent nodes and child nodes. In Vue, we can use recursive components to display tree-structured data. First, we need to define a tree component. Should
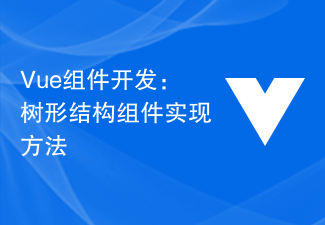
Vue component development: tree structure component implementation method, specific code examples are required 1. Introduction In Web development, tree structure is a common data display method, often used to display menus, file directories and other data. As a popular front-end framework, Vue provides a convenient component-based development method, making the implementation of tree-structure components simple and reusable. This article will introduce how to use Vue to develop a tree structure component and provide specific code examples. 2. Implementation Ideas To implement a tree structure component, you generally need to consider the following points:
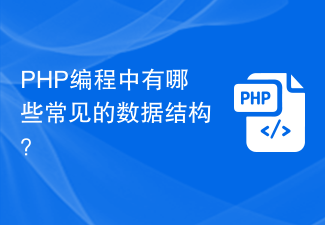
In the PHP programming language, data structure is a very important concept. It is a method used to organize and store data in program design. PHP has various data structure capabilities, such as arrays, linked lists, stacks, etc., making it highly valuable in actual programming. In this article, we will introduce several common data structures in PHP programming so that programmers can master them proficiently and apply them flexibly. Array Array is a basic data type in PHP programming. It is an ordered collection composed of the same type of data and can be stored under a single variable name.
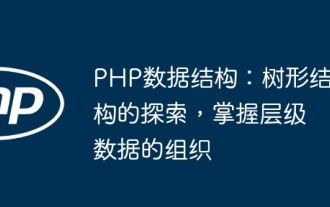
The tree structure is a non-linear structure that organizes data hierarchically, and can be represented and traversed recursively or iteratively in PHP. Representation methods include recursion (using class) and iteration (using array); traversal methods include recursive traversal and iterative traversal (using stack). In the actual case, the file system directory tree is efficiently organized using a tree structure to facilitate browsing and obtaining information.
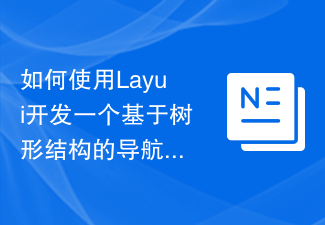
How to use Layui to develop a tree-structure-based navigation menu Navigation menu is one of the common components in web development, and tree-structure-based navigation menu can provide better user experience and functional integrity. This article will introduce how to use the Layui framework to develop a navigation menu based on a tree structure and provide specific code examples. 1. Preparation work Before starting development, you need to confirm that the Layui framework has been installed and that the relevant Layui resource files have been correctly introduced into the required HTML page. 2. Count
