


How does the collaboration of C++ and assembly language improve performance in embedded applications?
To optimize the performance of embedded systems, C and assembly language can be used collaboratively. Specific strategies include: function-specific assembly language optimization, inline assembly, and compiler inlining. For example, rewriting the assembly language version of the sorting algorithm can take advantage of the underlying hardware instructions and fine-tune it, resulting in significant performance improvements.
Optimizing performance in embedded systems: C and assembly language collaboration
Embedded systems have strict requirements for performance optimization . By combining C and assembly language, we can significantly improve the efficiency of our applications.
Complementarity of C with Assembly Language
C is known for its high-level abstraction and object-oriented nature, making it ideal for implementing complex algorithms and data structures. However, when it comes to low-level operations and time-critical tasks, assembly language remains a key tool for optimizing performance.
Collaboration Strategy
To take full advantage of C and assembly language, you can use collaboration strategy. Here are common approaches:
- Function-specific assembly language optimization:Replace time-critical code segments in C functions with hand-optimized assembly language.
- Inline Assembly: Embed assembly language directly in C code to access CPU-specific instructions or registers.
- Compiler inlining: Force the compiler to insert assembly language into the generated code by using compiler flags to mark specific functions or blocks of code as inline.
Practical Case
Consider the following example of sorting an array in an embedded system:
// C++ 代码,使用 std::sort() std::sort(arr, arr + n);
We can rewrite the sorting algorithm by Optimize this code snippet for assembly language:
// 汇编语言快速排序 mov eax, [esp + 4] ; 数组的首地址 mov ebx, [esp + 8] ; 数组的长度 .loop: mov esi, ebx ; 操作数索引 mov edi, ebx ; 分区点索引 .loop2: cmp esi, edi ; 比较操作数和分区点 jle .l1 inc esi ; 递增操作数索引 jmp .loop2 ; 下一个操作数 .l1: mov eax, [eax + esi * 4] mov ebx, [eax + edi * 4] mov [eax + esi * 4], ebx mov [eax + edi * 4], eax inc edi ; 递增分区点索引 dec esi ; 递增操作数索引 cmp esi, 0 ; 是否还需要分区? jle .loop2 ; 跳到下一个分区 mov ecx, edi ; 计算左子数组的长度 dec edi ; 计算右子数组的长度 cmp ecx, 0 ; 是否有左子数组? jle .no_left ; 跳过排序左子数组 mov eax, [esp + 4] ; 数组的首地址 sub eax, edi * 4 ; 计算左子数组的首地址 push eax ; 将左子数组的首地址压栈 push ecx ; 将左子数组的长度压栈 call .loop ; 递归排序左子数组 .no_left: pop ecx ; 弹出右子数组的长度 push eax ; 将数组的首地址压栈 push ecx ; 将右子数组的长度压栈 call .loop ; 递归排序右子数组
By rewriting the sorting algorithm into assembly language, we can take advantage of the underlying hardware instructions to fine-tune performance.
Conclusion
By combining C and assembly language, embedded systems developers can achieve the high performance and low-level control required for complex applications. By following a collaborative strategy, we can combine the strengths of each language to optimize code and meet real-time constraints.
The above is the detailed content of How does the collaboration of C++ and assembly language improve performance in embedded applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










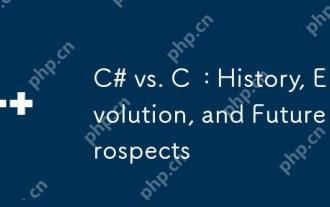
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
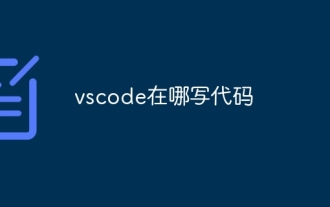
Writing code in Visual Studio Code (VSCode) is simple and easy to use. Just install VSCode, create a project, select a language, create a file, write code, save and run it. The advantages of VSCode include cross-platform, free and open source, powerful features, rich extensions, and lightweight and fast.
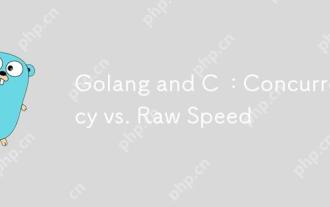
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
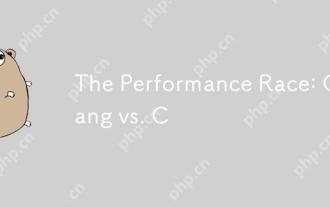
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
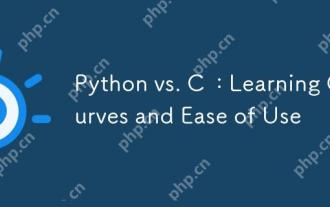
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
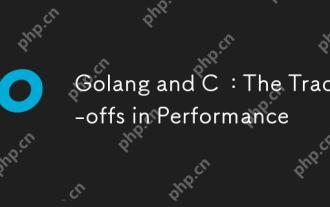
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
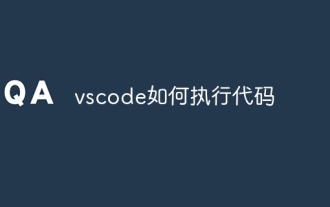
Executing code in VS Code only takes six steps: 1. Open the project; 2. Create and write the code file; 3. Open the terminal; 4. Navigate to the project directory; 5. Execute the code with the appropriate commands; 6. View the output.
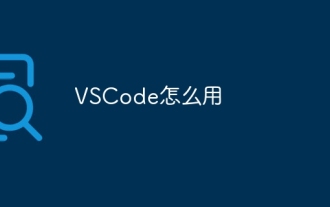
Visual Studio Code (VSCode) is a cross-platform, open source and free code editor developed by Microsoft. It is known for its lightweight, scalability and support for a wide range of programming languages. To install VSCode, please visit the official website to download and run the installer. When using VSCode, you can create new projects, edit code, debug code, navigate projects, expand VSCode, and manage settings. VSCode is available for Windows, macOS, and Linux, supports multiple programming languages and provides various extensions through Marketplace. Its advantages include lightweight, scalability, extensive language support, rich features and version
