Parsing of custom objects in js
This article mainly introduces the analysis of custom objects in js, which has certain reference value. Now I share it with everyone. Friends in need can refer to it
1. Objects
1. Literal created object
var person = { name: "sun", age: 18, work: function () { console.log(this.name + "is working..."); }, address: { home: "大屯里xxx路xxx小区xx单元xxx室", phone: "123456789", } }; person.work(); console.log(person.address.home);
2.Data description and access description setting
var person = { age: 18, address: { home: "大屯里xxx路xxx小区xx单元xxx室", phone: "123456789", } }; Object.defineProperties(person, { name: { value: "sun", // 该属性的值,可被读取 writable: true, // 表示能否修改属性的值,默认值为true configurable: true, // 表示能否delete该属性并重新定义,直接在对象上定义的属性默认值为true enumerable: true // 表示能否通过for-in枚举,直接在对象上定义的属性默认值为true }, work: { value: function(){ console.log(this.name + "is working..."); }, // 通过Object.defineProperty和Object.defineProperties定义属性, // 如果没有声明writable、configurable、enumerable,它们的默认值都是false } }); person.work(); console.log(person.address.home);
3.get and set
var circle = { value: 10, get girth(){ return 2 * 3.14 * this.R }, get area(){ return 3.4 * this.R * this.R }, }; Object.defineProperty(circle, "R", { get : function () { return this.value; }, set : function (val) { console.log("半径被修改了!"); this.value = val; } }); circle.R = 100; console.log("girth: " + circle.girth + "area: " + circle.area);
4.Data description and access description Check
var circle = { R: 10, // __proto__: null, get area(){ return 3.4 * this.R * this.R }, }; Object.defineProperty(circle, "site", { value: [0, 2.2, 4.1], // enumerable: true, // 是否可配置(读取),不设置为true时,Object.keys(circle))和Object.values(circle))将获取不到该键值对 }); console.log("R" in circle); // 检查属性 console.log(circle.hasOwnProperty("R")); // 检查自有的属性 console.log(circle.propertyIsEnumerable("R")); // 检查属性是否是可枚举的 // Object对象的方法 console.log(Object.keys(circle)); console.log(Object.values(circle)); console.log(Object.getOwnPropertyNames(circle)); // 检查对象自身所有属性 console.log(Object.getOwnPropertyDescriptor(circle, "R")); // 得到circle对象关于R属性的描述
2. prototype
1.prototype definition
- 每一次创建函数,解析器都会向函数中添加一个属性:prototype - 如果函数作为普通函数调用prototype,没有任何作用 - 当该函数以构造函数的形式调用时,它会有一个隐含的属性__proto__指向其原型对象 - 每个实例有各自的__proto__指向原型对象的prototype, 也就是原型对象中的属性和方法被调用函数"共享" - 当类的原型对象prototype指向的内存地址发生改变时,已创建实例的__proto__ !== prototype,也就是不会被覆盖。而新创建的实例仍然是__proto__ === prototyp
function Person(name, age) { this.name = name; this.age = age; } // Person.prototype.gender = "male"; // Person.prototype.sayHello = function () { // return this.name + ", " + this.age + "years old." // }; Person.prototype = { gender: "male", sayHello: function () { return this.name + ", " + this.age + "years old." } }; var p1 = new Person("孙悟空", 2000); p1.sayHello(); console.log(Person.prototype); console.log(Person.prototype.constructor === Person);
2.prototype and __proto__
function Person() {} var obj1 = { gender: "male"}; // 创建两个内存地址 var obj2 = { age: 200 }; Person.prototype = obj1; var p1 = new Person(); console.log(p1.__proto__ === Person.prototype); console.log(p1.__proto__.gender); console.log(Person.prototype); Person.prototype = obj2; var p2 = new Person(); console.log(p2.__proto__.age); console.log(Person.prototype); console.log(p1.__proto__.age); // undefined console.log(p2.__proto__.gender); // undefined console.log(p1.__proto__ === Person.prototype); // false,表示当prototype指向的内存地址改变时,已经创建的实例对象的__proto__仍指向原来的内存地址 console.log(p2.__proto__ === Person.prototype);
function Person() {} Person.prototype = {name: "xxx", age: 100,}; var p1 = new Person(); console.log(p1.__proto__.name); Person.prototype = { price: 998,}; var p2 = new Person(); console.log(p2.__proto__.price); console.log(p1.__proto__.price); // undefined console.log(p2.__proto__.name); // undefiend console.log(p1.__proto__ === Person.prototype); // false, 原型对象的内存地址引用已发生改变 console.log(p1.__proto__.age); // __proto__指向的内存地址被保留 console.log(p2.__proto__ === Person.prototype); // true
function Person() {} Person.prototype = { price: 60 }; var p1 = new Person(); Person.prototype = { price: 998}; var p2 = new Person(); console.log(p1.__proto__ === Person.prototype); // 依然是false console.log(p2.__proto__ === Person.prototype); // true
3. Sharability of prototype
// prototype非常类似python中的静态属性和静态方法。每个实例都可以访问同一块内存空间。 function Person() {} Person.prototype = {price: 60}; var p1 = new Person(); var p2 = new Person(); console.log(p1.__proto__.price); console.log(p2.__proto__.price); console.log(Person.prototype.price);
4. Inheritance of prototype
// 当访问实例对象的一个属性或方法时,它会先在对象自身中查找,如果有则直接使用;如果没有则在原型对象中继续查找,如果有则直接使用 function Person() {} Person.prototype = {price: 60}; var p1 = new Person(); var p2 = new Person(); console.log(p1.price); console.log(p2.price); console.log(Person.prototype.price);
3. Class
1. Class encapsulation
// 字面量方法(工厂方法) -- 直接在var obj = {}内部写代码,缺点是只实例化一次 // 构造函数方法 -- 只用构造函数声明this,缺点是可扩展性差,数据重复 // 原型方法 -- 只用prototype声明共有的属性和方法,缺点是实例的数据相同,不满足多态
1. Mixed constructor/prototype method
// 最广泛的使用方法 function Person(name, age) { this.name = name; this.age = age; } // prototype写在外面是为了保证其动态增加公共属性和方法 Person.prototype.sayHello = function () { console.log(this.name + ", " + this.age + " years old."); // 把共有的属性和方法封装到prototype中 }; var p = new Person("孙悟空", 2000); p.sayHello();
// 我把它写给Person的属性,让父类也能够访问 function Person(name, age) { Person.group = Person.prototype.group = "西天取经组"; Person.toString = Person.prototype.toString = function (){ console.log("Person: " + Person.group) }; this.name = name; this.age = age; this.sayHello = function () { console.log(this.name + ", " + this.age + "years old.") }; } var person = new Person("孙悟空", 2000); console.log(person.constructor); // 检查构造器函数 console.log(person instanceof Person); // 检查是否为其原型类 person.sayHello(); Person.toString();
2. Dynamic prototype method
// 也是常用的方法 function Person(name, age) { this.name = name; this.age = age; if (typeof Person._initialized === "undefined"){ Person.prototype.sayHello = function () { console.log(this.name + ", " + this.age + " years old."); }; Person._initialized = true; } } var p = new Person("孙悟空", 2000); p.sayHello();
3. Mixed factory Method
// 混合工厂方法 -- 存在与工厂方法类似的问题,不建议使用 function Person(name, age) { var obj = {}; obj.name = name; obj.age = age; obj.sayHello = function () { console.log(this.name + ", " + this.age + " years old."); }; return obj } var p = new Person("孙悟空", 2000); p.sayHello();
4. Discuss the class structure again
function Person(name, age) { // 静态属性 Person.group = "西天取经四人组,暗合金木水火土"; // 静态方法 Person.introduce = function () { console.log("贫僧自东土大唐而来") }; // 实例属性 this.name = name; this.age = age; // 实例方法,应该写在prototype中 this.say = function () { console.log("hello, i'm " + this.name); }; Person.prototype.introduce = Person.introduce; // 此时Person类和其实例都可以使用introduce方法 // 父类使用实例方法 Person.example = Person.prototype.example = function (self) { self = self || this; console.log(self.name + " " + self.age); } } // 在python中,实例可以访问父类的属性和方法,父类也可以使用实例方法 // 在java和js中,实例不能调用父类的静态属性和静态方法,父类不能使用实例方法 // 如果想让实例和父类共享一个属性或者方法,就只能放到方法区并创建引用 var sun = new Person("孙悟空", 2000); Person.introduce(); // 父类调用静态方法 sun.say(); sun.introduce(); // 实例调用静态方法 Person.example(sun); // 父类调用实例方法 sun.example(); // 子类调用实例方法 // 可见,prototype是父类和实例的沟通桥梁
2. Custom class
function Person(name, age) { this.name = name; this.age = age; this.sayHello = function () { console.log(this.name + ", " + this.age + "years old.") }; } function New(Person) { return function () { var obj = {"__proto__": Person.prototype}; // 必须写在这里 Person.apply(obj, arguments); // arguments同this一样,是默认自带的关键字,用于存储传入的参数 return obj } } var temp = New(Person); var p1 = temp("孙悟空", 2000); var p2 = temp("猪八戒", 1); p1.sayHello(); p2.sayHello();
3. Class inheritance
1.Copy inheritance literal Object (instance)
var person = { name: "Li", age: 16, address: { home: "none", city: "none", }, say: function(){ console.log("hello, guy.") } }; var child = {gender:"female",}; function extendDeeply (p, c){ var c = c || {}; for (var prop in p) { if (typeof p[prop] === "object") { c[prop] = (p[prop].constructor === Array) ? [] : {}; extendDeeply(p[prop], c[prop]); } else { c[prop] = p[prop]; } } } extendDeeply(person, child); console.log(child); child.say();
2.call and apply implement object inheritance
function Person(name, age) { this.name = name; this.age = age; this.address = { home: "none", city: "none", } } Person.prototype.say = function () { console.log("hello, guy.") }; // 它继承的只是实例对象this,无法继承父类原型prototyp function Child(name, age) { Person.call(this, name, age); this.gender = "female"; } var child = new Child("Li", 16); console.log(child); // child.say(); 报错: child.say is not a function.
对象继承的缺点:只继承了实例对象的可访问的属性和方法,没有继承原型
3.Prototype chain inheritance
// 原型链继承 function Person() {} Person.prototype.name = "Person"; Person.prototype.toString = function () { console.log(this.name); }; function Child(name, age) { this.age = age; this.name = name; } Child.prototype = Person.prototype; Child.prototype.constructor = Child; var child = new Child("Li", 16); console.log(child.name + " " + child.age); child.toString(); // 其缺点是之继承了原型,没有继承实例
4.create implements class inheritance
function Person(name, age) { this.name = name; this.age = age; this.address = { home: "none", city: "none", } } Person.prototype.say = function () { console.log("hello, guy.") }; function Child(P, name, age) { function F() {} F.prototype = new P(name, age); var c = new F(); return c; } Child.prototype.constructor = Child; // 无法修正 var child = new Child(Person, "Li", 16); console.log(child); console.log(child.name); child.say(); console.log(child.constructor); // 结果为[Function: Person],构造器指向无法修正 console.log(child instanceof Child); // false console.log(child instanceof Person); // true
5. Object.create implements class inheritance – the recommended way
// Object.create继承,实现原理和上面的create类似 // 1.创建父类 function Person() {} Person.prototype.sayPerson = function () { console.log("hello, Person.") }; // 2.创建子类 function Child(gender) {this.gender = gender;} // 3.create继承 // Object.create的第二个参数是属性描述 Child.prototype = Object.create(Person.prototype, { name: { value: "Li", writable: true, enumerable: true, configurable: true, }, age: { value: 16, writable:true, configurable:true, enumerable:true, }, }); // 重写子类prototype Child.prototype.constructor = Child; // constructor 修正 // 4.在create之后写子类的prototype Child.prototype.sayChild = function () { console.log("hello, Child.") }; var child = new Child("female"); console.log(child); console.log(child.name + " " + child.age); child.sayChild(); child.sayPerson();
5. Combination inheritance – the recommended way
function Person(name, age) { this.name =name; this.age = age; } Person.prototype.toString = function () { console.log(this.name + " " + this.age); }; function Child(name, age, gender) { Person.call(this, name, age); this.gender = gender; } Child.prototype = new Person(); // new时不传参数,是为了只继承原型,即Child.prototype = Person.prototype // Child.prototype = Person.prototype; // 两者等价 Child.prototype.constructor = Child; var child = new Child("Li", 16, "female"); console.log(child); child.toString(); console.log(child instanceof Child); // true console.log(child instanceof Person); // true
6. Inheritance summary
js继承需要继承两部分内容: - 一部分是父类构造函数中的this定义属性和方法,相当于继承初始化的数据 - 另一部分是父类的prototype,相当于继承实例方法- 要实现this的继承,可以用call(apply);要实现prtotype的继承,可以用原型链 - 要实现两者的继承,可以用this+prototype的组合方式,Object.create本质上也是这种思路
7. The relationship between prototype, constructor and __proto__ in inheritance
The above is the entire content of this article. I hope it will be helpful to everyone's learning. For more related content, please pay attention to PHP Chinese website!
Related recommendations:
Analysis of event models in js
How to pass js variable values to php
The above is the detailed content of Parsing of custom objects in js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
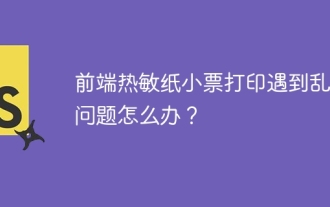
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
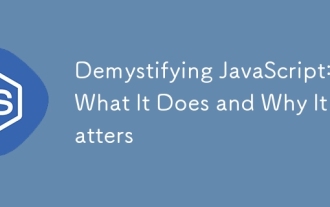
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
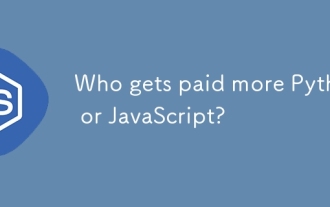
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
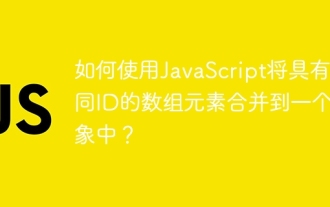
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
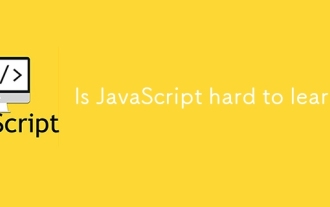
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
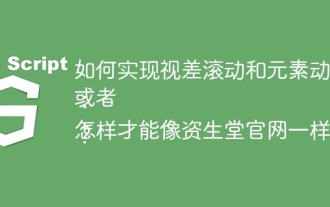
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
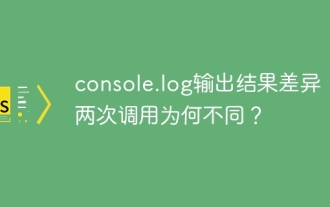
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
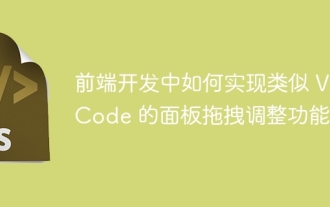
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
