


Example sharing of the nine-square puzzle game implemented by jQuery+vue.js
This article mainly introduces the nine-square puzzle game implemented by jQuery+vue.js, and analyzes the related operation skills of jQuery combined with vue.js for pictures in the form of a complete example. Friends who need it can refer to it. I hope it can help everyone.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <style> * { margin: 0; padding: 0; } /*#piclist { width: 600px; height: 600px; background-color: green; }*/ .nitem { /*width: 200px;*/ /*height: 200px;*/ float: left; background: url(img/meinv.jpg) 0px 0px no-repeat; -webkit-background-size: 600px 600px; background-size: 600px 600px; font-size: 33px; color: red; font-weight: bold; cursor: pointer; } /*.nitem:nth-child(2){ background-position: -200px 0; } .nitem:nth-child(3){ background-position: -400px 0; } .nitem:nth-child(4){ background-position: 0px -200px; } .nitem:nth-child(5){ background-position: -200px -200px; } .nitem:nth-child(6){ background-position: -400px -200px; } .nitem:nth-child(7){ background-position: 0px -400px; } .nitem:nth-child(8){ background-position: -200px -400px; } .nitem:nth-child(9){ background-position: -400px -400px; }*/ .fn-clear { clear: both; height: 0; line-height: 0px; font-size: 0px; } </style> </head> <body> <p id="appbox" :style="{ width:width+'px',height:height+'px' }"> <p id="piclist"> <p class="nitem" v-for="(item,index) in itemlist" :class="{remove : index === 0}" :index="index " v-bind:style="{ 'backgroundPosition':-px(index)+'px -' + py(index) + 'px', width : width / rows + 'px', height : height / cols + 'px'}">{{index}} </p> </p> </p> </body> <script src=js/jquery-1.9.1.min.js></script> <script src=js/vue.min.js></script> <script> var olen = 0, oi = 0, cindex = 0, oa = new Array(), oindex = 0, listarray = new Array(); var vm = new Vue({ el: "#appbox", data: { itemlist: [], rows: 3, cols: 3, width: 600, height: 600, }, methods: { px(index){ return (index % this.rows) * (this.width / this.rows) }, py (index){ return parseInt((index / this.cols)) * (this.height / this.cols); } } }); for (var i = 0; i < vm.rows * vm.cols; i++) { vm.itemlist.push(i); } function getrow(index) { return parseInt(index / vm.cols); } function getcols(index) { return index % vm.rows; } function getBound(index) { var left = index - 1; var right = index + 1; var top = index - vm.rows; var bottom = index + vm.rows; var len = vm.itemlist.length; //总长度 var currentRow = getrow(index); var currentCol = getcols(index); var roundArr = new Array(); if (left >= 0 && left < len && getrow(left) == currentRow) { roundArr.push(left); } if (right >= 0 && right < len && getrow(right) == currentRow) { roundArr.push(right); } if (top >= 0 && top < len && getcols(top) == currentCol) { roundArr.push(top); } if (bottom >= 0 && bottom < len && getcols(bottom) == currentCol) { roundArr.push(bottom); } return roundArr; } function box_switch(i, j) { var iobj = $('#piclist .nitem').eq(i); var jobj = $('#piclist .nitem').eq(j); var tobj = iobj.clone(); jobj.after(tobj); iobj.replaceWith(jobj); } vm.$nextTick(function () { $('.remove').css({ background: 'none', backgroundColor: 'green' }); }); function box_rand(times) { for (var i = 0; i < times; i++) { oindex = $('.remove').index(); oa = getBound(oindex); olen = oa.length; oi = Math.floor(Math.random() * olen); cindex = oa[oi]; box_switch(cindex, oindex); } listarray.length = 0; $.each($('.nitem'), function (i, item) { listarray.push($(item).attr('index')); }); if (listarray.join(',') == vm.itemlist.join(',')) { box_rand(times); } } $(function () { //打乱图片 box_rand(20); $('.nitem').on('click ', function () { var cindex = $(this).index(); var oindex = $('.remove').index(); var oRound = getBound(oindex); //空盒子四周的索引 if ($.inArray(cindex, oRound) < 0) { //不在 return false; } else { box_switch(oindex, cindex); var listArray = new Array(); $.each($('.nitem'), function (i, item) { listArray.push($(item).attr('index')); }) if (listArray.join(',') == vm.itemlist.join(',')) { alert('success') } else { console.log('失败') } } }); }) </script> </html>
Related recommendations:
Detailed example of implementing a guessing game based on vue components
html5 tutorial on making mooncake-eating games
How to use js to develop and implement a simple snake game
The above is the detailed content of Example sharing of the nine-square puzzle game implemented by jQuery+vue.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
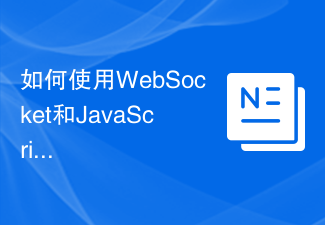
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
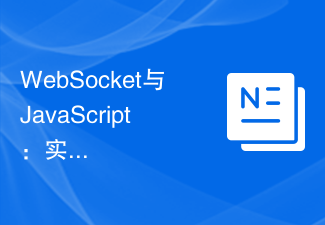
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
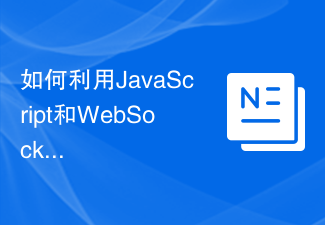
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
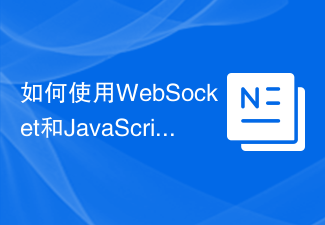
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
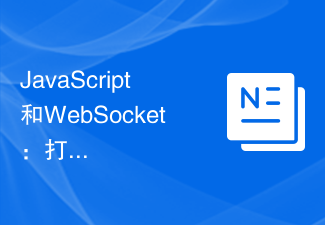
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
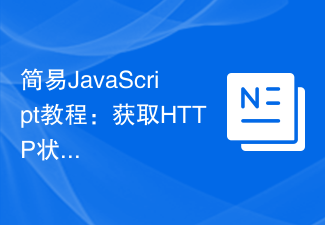
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
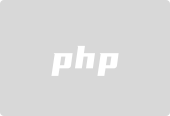
Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
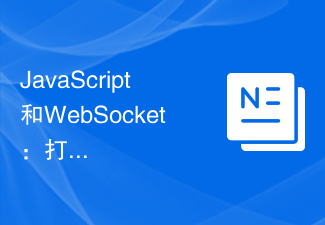
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
