What are the life cycle functions of React components?
Each component has some life cycle functions.
When a component instance is created and inserted into the DOM, the following functions will be called
constructor componentWillMount render componentDidMount
to change the component's state or props will cause updates. When the component is re-rendered, the following methods will be called.
componentWillReceiveProps shouldComponentUpdate componentWillUpdate render componentDidUpdate
When the component is removed from DOM, the following methods will be called.
componentWillUnmount
一.render()
render method is required. The return value of render is of the following type:
( 1) react element: either a custom component or a native DOM component
(2)String or number: will Rendered into text nodes in DOM
(3)Portals:Created by ReactDOM.createPortal
(4)null: Nothing will be rendered
(5)Boolean:Nothing will be rendered
(6)Array containing multiple elements
render(){ return [ <li key='1'>1</li>, <li key='2'>2</li> ] }
The render method should be simple. The state of the component cannot be modified in render. Each time render is called, a new result will be returned. And you cannot interact with the browser in render. If you need to interact with the browser, execute it in componentDidMount or other life cycle functions.
##2.constructor(props)
reactComponent The constructor is called before the component is loaded. If constructor is not explicitly defined, the default constructor will be called when instantiating the component. If constructor is explicitly defined in a subclass of React.Component, then It is necessary to call super(props) first in constructor. react state is legal, but there is a problem: when props are updated, state will not be updated. The solution is: update state with new props in the component's componentWillReceiveProps(nextProps). Although this can solve the problem, it is not recommended. It is recommended to upgrade the state to the nearest public parent component render. Modifying state in componentWillMount will not cause the component to be re-rendered. This method will only be called for server-side rendering. It is recommended to replace this method with constructor. #componentDidMount will be triggered immediately when the component is loaded. Modifying the state in this function will cause the component to be re-rendered. . The DOM cannot be manipulated until the component is loaded. If you need to load remote data, it is a good idea to send network requests here. componentWillReceiveProps will be triggered when the component that has been reproduced accepts new props. If you need to update state to respond to the update of props, you can update state through the setState method here. When the component receives props for the first time, this method will not be called. Note: props may not be changed but this method may be called, so the current shouldComponentUpdate will be called before rendering. This method returns true by default. The initial rendering and Using forceUpdate, this method will not be called. If shouldComponentUpdate returns false, subsequent componentWillUpdate, render and componentDidMount will not be called , and the component and its subcomponents will not be re-rendered. #7.componentWillUpdate(nextProps, nextState) ##8.componentDidUpdate(prevProps, prevState) ##9.componentWillUnmount()This method will be called immediately before the component is destroyed. In this method, some necessary cleanup can be done. The above is the detailed content of What are the life cycle functions of React components?. For more information, please follow other related articles on the PHP Chinese website!
It is a good choice to instantiate state in the constructor. Here is an example of a piece of code##constructor(props) {
super(props);
this.state = {
color: props.initialColor
};
}
componentWillMount, componentWillMount Will be called before calling
It will be called immediately before loading occursIV.componentDidMount()
props will be # removed in this method ##next props comparison is necessary. #6.shouldComponentUpdate(nextState,nextProps)
When new props or
state are accepted, When new props or state are received, this method will be called immediately before re-rendering. You cannot
this.setState() in this method. This method will not be called for the first renderingDOM here. When you find that the current props are different from the previous props, it is a good idea to send a network request here

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
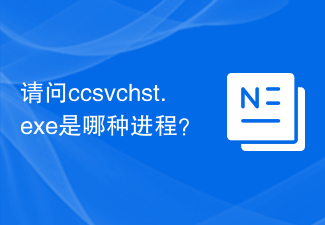
ccsvchst.exe is a common process file that is part of the Symantec Endpoint Protection (SEP) software, and SEP is an endpoint protection solution developed by the well-known network security company Symantec. As part of the software, ccsvchst.exe is responsible for managing and monitoring SEP-related processes. First, let’s take a look at SymantecEndpointProtection(
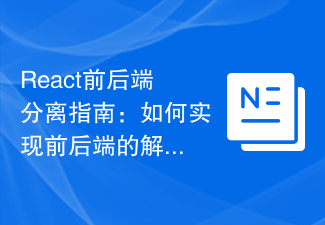
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
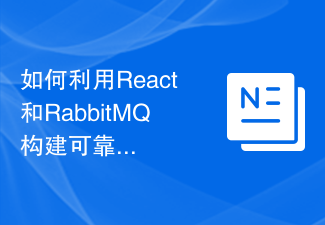
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
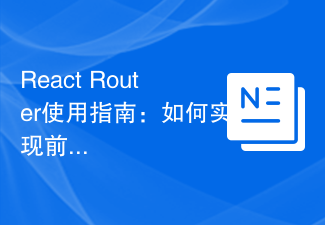
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need

How to use React and Apache Kafka to build real-time data processing applications Introduction: With the rise of big data and real-time data processing, building real-time data processing applications has become the pursuit of many developers. The combination of React, a popular front-end framework, and Apache Kafka, a high-performance distributed messaging system, can help us build real-time data processing applications. This article will introduce how to use React and Apache Kafka to build real-time data processing applications, and
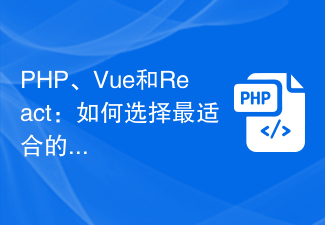
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
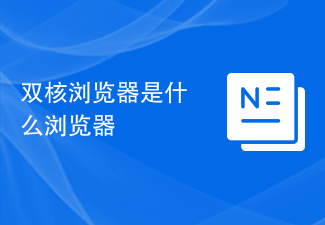
Dual-core browser is a browser software that integrates two different browser cores. The kernel is the core part of the browser, responsible for rendering web content and executing web scripts and other functions. Traditional browsers generally use only a single kernel, such as IE browser using Trident kernel, Chrome browser using WebKit/Blink kernel, Firefox browser using Gecko kernel, etc. The dual-core browser integrates two different cores into one browser, and users can freely switch between them as needed. The emergence of dual-core browsers
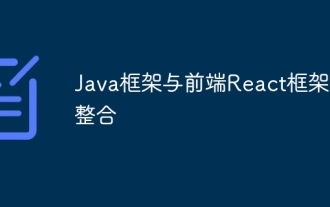
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
