Detailed explanation of Constructor Prototype Pattern in PHP
The prototype mode is a creator mode, which is characterized by "copying" an existing instance to return a new instance instead of creating a new instance. This article will provide a detailed explanation of the prototype mode with examples. I hope it will be helpful to everyone.
Main roles in the prototype pattern
Abstract prototype (Prototype) role: declare an interface that clones itself
Concrete Prototype (Concrete Prototype) role: Implement an operation of cloning yourself
When most of a class is the same and only some parts are different, if a large number of objects of this class are needed, it will be very expensive to instantiate the same parts repeatedly every time. , and if you create the same parts of the object before cloning, you can save overhead.
One implementation method for PHP is that the __construct() and initialize functions handle the initialization of this class separately. The prototype is placed in construct, which is the public part, and the special part of each object is placed in initialize. In this way, we first create a class without initializing it, and then clone this class and then initialize it every time.
This is mentioned in the zend framework official manual http://framework.zend.com/manual/2.0/en/user-guide/database-and-models.html, but it is not explained in detail. I will explain it below. Let’s analyze it
1. Introduction
There is an albumTable class in the zf2 model, which is equivalent to an assistant class for operating database actions, and tablegateway is used in it.
In order to initialize the albumtable with the same class every time, the initialization work is placed in getServiceConfig() of the module.php file in the root directory. The factory mode is used, and through the callback function, each time the ServiceManager ($sm) When an object needs to be instantiated, it will be automatically called to create an alumniTable. We can see from the code below that creating an albumTable also requires creating an AlbumTableGateWay in the same way. This class uses the prototype pattern we are going to talk about.
2. Detailed code explanation
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
Note that TableGateWay does not use the prototype mode. It is the ResultSet class that is used. Whenever the tablegateway calls methods such as select() or insert(), a ResultSet will be created to represent the result. The common parts of these ResultSets are cloned, and unique partial classes such as data will be initialized.
3. More code examples
In order to understand this prototype more clearly, let’s put aside the big framework of zend and look at a complete code example. Example from
PHP Constructor Best Practices And The Prototype Pattern
The first half of this article about prototype pattern is actually a mixture of how to use inheritance in constructors to improve scalability. The two patterns may not seem very similar. It’s easy to understand. Let’s look directly at the prototype pattern part of the final code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
|
These classes actually correspond to the classes in the zend code above
Dbadapter -- adpater
RowGateWay -- ResultSet
UserRepository - TableGateWay
See the comments in the code for details.
The RowGateWay here can clearly see that a large number of instantiations are required in getusers, so the prototype mode is very necessary.
The following is the code to use this class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
##Related recommendations:
Detailed Explanation of the Adapter Pattern of PHP Design Pattern
Detailed Explanation of the Iterator Pattern of PHP Design Pattern
Detailed explanation of the observer pattern of php design pattern
The above is the detailed content of Detailed explanation of Constructor Prototype Pattern in PHP. For more information, please follow other related articles on the PHP Chinese website!
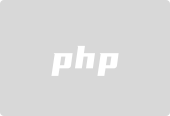
Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!
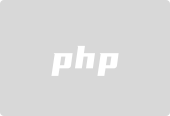
Hot Article
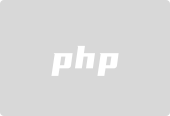
Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)
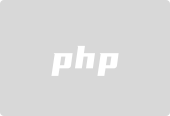
Hot Topics










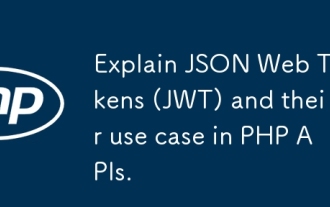
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
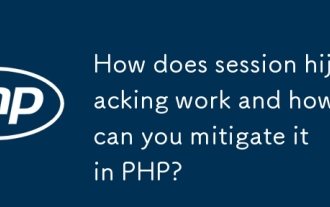
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
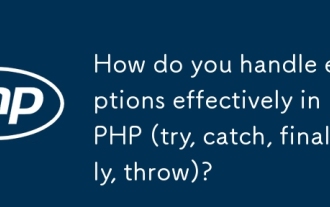
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
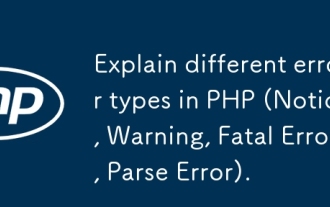
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
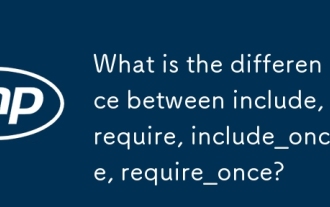
In PHP, the difference between include, require, include_once, require_once is: 1) include generates a warning and continues to execute, 2) require generates a fatal error and stops execution, 3) include_once and require_once prevent repeated inclusions. The choice of these functions depends on the importance of the file and whether it is necessary to prevent duplicate inclusion. Rational use can improve the readability and maintainability of the code.
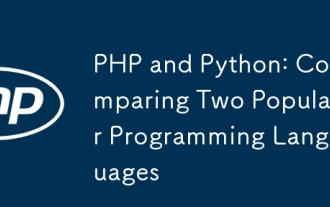
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
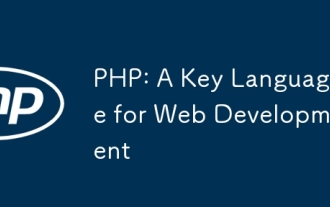
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
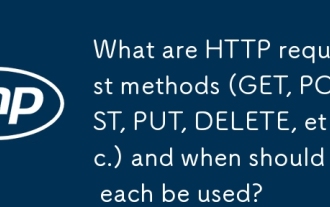
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
