How to write components in vue
This time I will show you how to write components in Vue, and what are the precautions when writing components in Vue. The following is a practical case, let's take a look.
Write a vue component
What I write below is how to write a single file component ending with .vue, which is a project built based on webpack . If you still don’t know how to use webpack to build a vue project, you can move to vue-cli.
A complete vue component will include the following three parts:
template: Template
js: Logic
css: Style
Each component has its own template, js and style. If a page is compared to a house, the components are the living room, bedroom, kitchen, and toilet in the house. If the kitchen is taken out separately, the components can be knives, range hoods...etc. That is to say, pages are made up of components, and components can also be made up of components. This makes it very flexible and has very low coupling.
Let’s first look at how a component is written in a .vue file:
Vue.component('simple-counter', { template: '<p id="inputBox"><input type="text"></p>', data () { // 数据 return { counter: 0 } }, methods: { // 写点方法 }, created () { // 生命钩子 }, computed: { // 计算属性 } })
What is template used for?
<template> <p id="inputBox"> <input type="text"> </p> </template> <!-- template就是这个组件的html,也就是下面部分(vue-loader会将template标签下的内容解析出来): --> <p id="inputBox"> <input type="text"> </p> <!-- 对应原生写法的话,就是template内的dom字符串 -->
js part
export default { data () { return { counter: 0 } }, methods: { // 方法 }, created () { // 生命钩子 }, computed: { // 计算属性 } } // 在这里很明显js部分就是对应的原生写法内的非template部分了。 // export default这个是es6的模块写法,不懂的可以先去了解es6的模块化
css part
<style lang="scss" scoped> ...样式 </style>
Introduction
How to reference this component in other components?
Component one (button.vue)
<template> <p class="button"> <button @click="onClick">{{text}}</button> </p> </template> <script> export default { props: ['text'], // 获取父组件的传值 data () { return { } }, methods: { onClick () { console.log('点击了子组件') } } } </script> <style lang="scss" scoped> .button { button { width: 100px; } } </style>
Component two (box.vue)
<template> <p class="box"> <v-button :text="text"></v-button> <!--使用组件并传值(text)--> </p> </template> <script> import Button from './button.vue' // 引入子组件 export default { components: { 'v-button': Button }, data () { return { text: '按键的name' } }, methods: { } } </script>
Detailed explanation of the use of webpack hot refresh and hot loading
code spliting optimization Vue packaging steps detailed explanation
The above is the detailed content of How to write components in vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
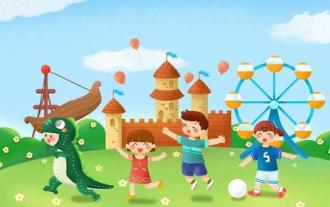
How to set up keyboard startup on Gigabyte's motherboard. First, if it needs to support keyboard startup, it must be a PS2 keyboard! ! The setting steps are as follows: Step 1: Press Del or F2 to enter the BIOS after booting, and go to the Advanced (Advanced) mode of the BIOS. Ordinary motherboards enter the EZ (Easy) mode of the motherboard by default. You need to press F7 to switch to the Advanced mode. ROG series motherboards enter the BIOS by default. Advanced mode (we use Simplified Chinese to demonstrate) Step 2: Select to - [Advanced] - [Advanced Power Management (APM)] Step 3: Find the option [Wake up by PS2 keyboard] Step 4: This option The default is Disabled. After pulling down, you can see three different setting options, namely press [space bar] to turn on the computer, press group
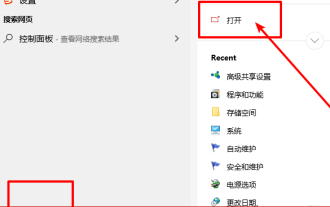
Many users always encounter some problems when playing some games on win10, such as screen freezes and blurred screens. At this time, we can solve the problem by turning on the directplay function, and the operation method of the function is also Very simple. How to install directplay, the old component of win10 1. Enter "Control Panel" in the search box and open it 2. Select large icons as the viewing method 3. Find "Programs and Features" 4. Click on the left to enable or turn off win functions 5. Select the old version here Just check the box
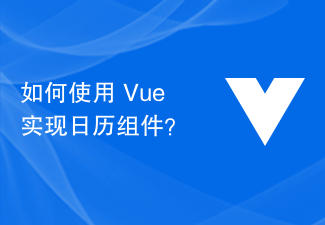
Vue is a very popular front-end framework. It provides many tools and functions, such as componentization, data binding, event handling, etc., which can help developers build efficient, flexible and easy-to-maintain Web applications. In this article, I will introduce how to implement a calendar component using Vue. 1. Requirements analysis First, we need to analyze the requirements of this calendar component. A basic calendar should have the following functions: display the calendar page of the current month; support switching to the previous month or next month; support clicking on a certain day,
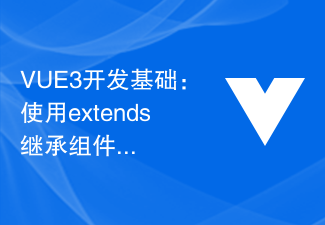
Vue is one of the most popular front-end frameworks currently, and VUE3 is the latest version of the Vue framework. Compared with VUE2, VUE3 has higher performance and a better development experience, and has become the first choice of many developers. In VUE3, using extends to inherit components is a very practical development method. This article will introduce how to use extends to inherit components. What is extends? In Vue, extends is a very practical attribute, which can be used for child components to inherit from their parents.
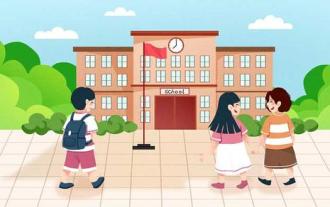
How to enable the direct connection of the independent graphics card of the Shenzhou Xuanlong m7. To enable the direct connection function of the independent graphics card of the Shenzhou Xuanlong m7, you can follow the following steps: 1. First, make sure that you have installed the driver of the independent graphics card. You can go to the official Shenzhou website or the official website of the independent graphics card manufacturer to download and install the latest driver suitable for your graphics card model. 2. On the computer desktop, right-click a blank space and select "NVIDIA Control Panel" in the pop-up menu (if it is an AMD graphics card, select "AMDRadeon Settings"). 3. In the control panel, find "3D Settings" or a similarly named option and click to enter. 4. In "3D Settings" you need to find "Global Settings" or a similarly named option. Here you can specify the use of a unique
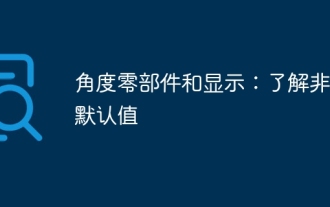
The default display behavior for components in the Angular framework is not for block-level elements. This design choice promotes encapsulation of component styles and encourages developers to consciously define how each component is displayed. By explicitly setting the CSS property display, the display of Angular components can be fully controlled to achieve the desired layout and responsiveness.
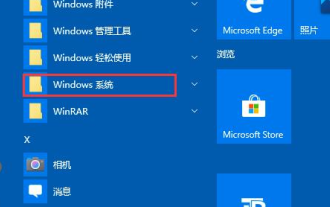
Win10 old version components need to be turned on by users themselves in the settings, because many components are usually closed by default. First we need to enter the settings. The operation is very simple. Just follow the steps below. Where are the win10 old version components? Open 1. Click Start, then click "Win System" 2. Click to enter the Control Panel 3. Then click the program below 4. Click "Enable or turn off Win functions" 5. Here you can choose what you want to open

Vue component development: Progress bar component implementation method Preface: In Web development, the progress bar is a common UI component, often used to display the progress of operations in scenarios such as data requests, file uploads, and form submissions. In Vue.js, we can easily implement a progress bar component by customizing components. This article will introduce an implementation method and provide specific code examples. I hope it will be helpful to Vue.js beginners. Component structure and style First, we need to define the basic structure and style of the progress bar component.
