


A brief analysis of implicit type conversion in JavaScript_javascript skills
If you explicitly convert a certain type to another type through a function or method call, it is called explicit conversion, and the opposite is called implicit type conversion. The words "display type conversion" and "implicit type conversion" are not found in Google and Wikipedia. Let’s call it that.
1. Implicit type conversions in operations
1, " " operator
var a = 11, b = '22';
var c = a b;
Here the engine will first turn a into the string "11" and then connect it with b , becomes "1122". Some people may wonder, why not turn b into the number 22 and then perform arithmetic addition, in which case c will be 33. There is no reason why, when one on both sides of the operator " " is a numeric type and the other is a string type, the js engine stipulates that the string concatenation operation be performed instead of the arithmetic addition operation. Using the feature of operator " ", Number can be easily converted into String. For example,
var a = 11;
alert(typeof a); //-->number
a = a '';
alert(typeof a); //-->string
2," -" operator
"-" can be a unary operator (negative) or a binary operator (subtraction operation). For example,
var a = 11, b = '5';
var c = a - b;
alert(typeof c); //--> number
This is contrary to the above " ", and the string b will be implicit is converted into the number 5 and then arithmetic subtraction is performed. Using this feature, you can easily convert String into Number
var a = '11';
a = a - '';
alert(typeof a);// -->number
two , implicit type conversions in statements
1, if
var obj = {name:'jack'}
if(obj){
//do more
}
Here obj will be implicitly converted to Boolean type
2, while
var obj = {name:'jack'}
while(obj){
//do more
}
Same as if
3, type conversion when for in
Implicit conversion from identifier to string occurs when defining an object literal.
var person = {'name':'jack', "age":20,school:'PKU'};
for(var a in person){
alert(a ": " typeof a);
}
Here, single/double quotes are added to name and age respectively to emphasize that they are of String type, while single/double quotes are not added to school. We traverse the properties of the object to see its type. It is found that school is also implicitly converted to String type.
The index of the array is actually a string type. It's amazing, but it's true. For example,
var ary = [1,3,5,7 ];
for(var a in ary){
alert(a ": " typeof a);
}
3. Exist during alert Implicit type conversion of
String .prototype.fn = function(){return this};
var a = 'hello';
alert(typeof a.fn()); //-->object
alert(a. fn()); //-->hello
Add a fn method to the String prototype, which returns this. We know that this can be understood as an instance object of the current class. Since it is an object, typeof a.fn() naturally returns object.
The key is the last alert(a.fn()). What a.fn() returns is obviously an object, but it is implicitly converted into a string "hello" for display.
The same situation happens with numeric types, such as
Number.prototype.fn = function(){return this};
var a = 10;
alert(typeof a.fn());//-->object
alert(a. fn()); //-->10
a.fn() returns the object type, but it will be converted implicitly when alert(a.fn()) into numbers.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
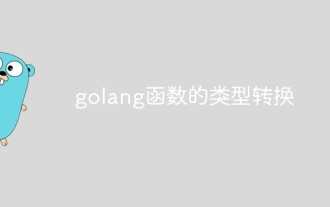
In-function type conversion allows data of one type to be converted to another type, thereby extending the functionality of the function. Use syntax: type_name:=variable.(type). For example, you can use the strconv.Atoi function to convert a string to a number and handle errors if the conversion fails.
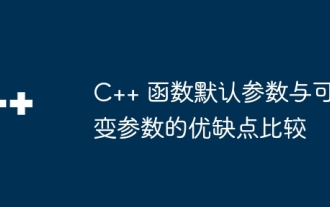
The advantages of default parameters in C++ functions include simplifying calls, enhancing readability, and avoiding errors. The disadvantages are limited flexibility and naming restrictions. Advantages of variadic parameters include unlimited flexibility and dynamic binding. Disadvantages include greater complexity, implicit type conversions, and difficulty in debugging.
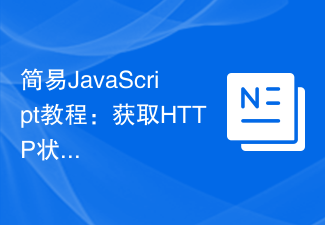
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
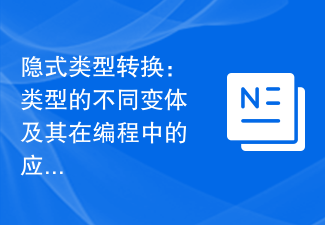
Explore the different types of implicit type conversions and their role in programming Introduction: In programming, we often need to deal with different types of data. Sometimes, we need to convert one data type to another type in order to perform a specific operation or meet specific requirements. In this process, implicit type conversion is a very important concept. Implicit type conversion refers to the process in which the programming language automatically performs data type conversion without explicitly specifying the conversion type. This article will explore the different types of implicit type conversions and their role in programming,
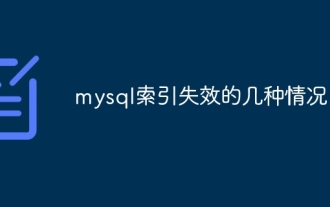
Common situations: 1. Use functions or operations; 2. Implicit type conversion; 3. Use not equal to (!= or <>); 4. Use the LIKE operator and start with a wildcard; 5. OR conditions; 6. NULL Value; 7. Low index selectivity; 8. Leftmost prefix principle of composite index; 9. Optimizer decision; 10. FORCE INDEX and IGNORE INDEX.
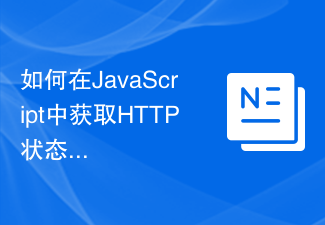
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
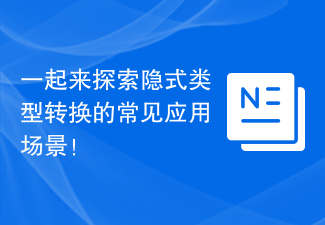
Let’s explore common application scenarios of implicit type conversion! Introduction: In programming languages, implicit type conversion is an automatically performed data type conversion process. In some programming languages, this conversion is performed implicitly, without the need to explicitly tell the compiler or interpreter to perform the conversion. Implicit type conversion has a wide range of application scenarios in programming. This article will discuss some of the common application scenarios. Implicit type conversion in numerical calculations In numerical calculations, operations between different types of data are often required. When different types of data
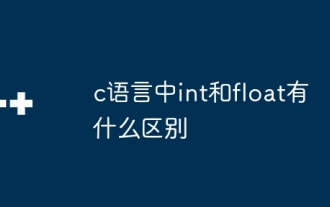
The difference between int and float variables in C language is that they have different types: int is used to store integers, while float is used to store decimals. Storage size: int usually takes 4 bytes, and float also takes 4 bytes. Precision: int represents an exact integer, while float has limited precision. Range: int typically ranges from -2^31 to 2^31-1, while float has a wider range. Arithmetic operations: int and float can perform arithmetic operations and comparisons, but the results may be affected by precision limitations. Type conversion: Explicit or implicit type conversion can be performed between int and float.
