Java Data Structures and Algorithms: A Practical Guide to Big Data Analysis
Big data analysis applications of data structures and algorithms in Java Master data structures (arrays, linked lists, stacks, queues, hash tables) and algorithms (sorting, search, hashing, graph theory, and union lookup) for big data Data analysis is crucial. These data structures and algorithms provide mechanisms for efficient storage, management, and processing of massive amounts of data. Practical examples demonstrate the application of these concepts, such as using hash tables to quickly find word frequencies and using graph algorithms to find relevant nodes in social networks.
Java Data Structures and Algorithms: A Practical Guide to Big Data Analysis
Introduction
Master Data Structures and Algorithms Essential for big data analysis. This article will provide a practical guide to introduce key data structures and algorithms in Java, and demonstrate their application in big data analysis through practical cases.
Data structure
- Array: Ordered collection of elements, accessed using index.
- Linked list: A linear structure composed of nodes, each node contains data and a pointer to the next node.
- Stack: Last-In-First-Out (LIFO) data structure supports fast push and pop operations.
- Queue: First-In-First-Out (FIFO) data structure supports fast enqueue and dequeue operations.
- Hash table: A fast lookup structure that uses a hash function to map keys to values.
Algorithm
- Sort: Arrange the data set in a specific order.
- Search: Find specific elements in a data collection.
- Hash: Use a hash function to generate a unique representation of the key.
- Graph theory: Algorithms that study graphs (sets of nodes and edges).
- Union lookup: Maintain a disjoint set of elements.
Practical case
Case 1: Use hash table to quickly find word frequency
import java.util.HashMap; import java.util.StringJoiner; public class WordFrequencyCounter { public static void main(String[] args) { String text = "This is an example text to count word frequencies"; // 使用哈希表存储单词及其频率 HashMap<String, Integer> frequencyMap = new HashMap<>(); // 将文本拆分为单词并将其添加到哈希表中 String[] words = text.split(" "); for (String word : words) { frequencyMap.put(word, frequencyMap.getOrDefault(word, 0) + 1); } // 从哈希表中打印每个单词及其频率 StringJoiner output = new StringJoiner("\n"); for (String word : frequencyMap.keySet()) { output.add(word + ": " + frequencyMap.get(word)); } System.out.println(output); } }
Case 2: Using graph algorithms to find relevant nodes in social networks
import java.util.*; public class SocialNetworkAnalyzer { public static void main(String[] args) { // 创建一个图来表示社交网络 Map<String, Set<String>> graph = new HashMap<>(); // 添加节点和边到图中 graph.put("Alice", new HashSet<>(Arrays.asList("Bob", "Carol"))); graph.put("Bob", new HashSet<>(Collections.singleton("Dave"))); ... // 使用广度优先搜索找到与 Alice 相关的所有节点 Queue<String> queue = new LinkedList<>(); queue.add("Alice"); Set<String> visited = new HashSet<>(); while (!queue.isEmpty()) { String current = queue.remove(); visited.add(current); for (String neighbor : graph.get(current)) { if (!visited.contains(neighbor)) { queue.add(neighbor); } } } // 打印与 Alice 相关的所有节点 System.out.println(visited); } }
Conclusion
By mastering data structures and algorithms, Java programmers can manage efficiently and analyzing big data. This article provides practical examples that demonstrate the practical application of these concepts, enabling programmers to build complex and efficient big data analytics solutions.
The above is the detailed content of Java Data Structures and Algorithms: A Practical Guide to Big Data Analysis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










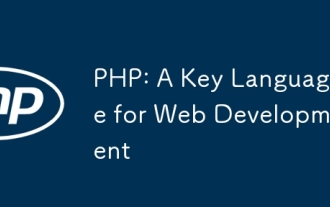
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
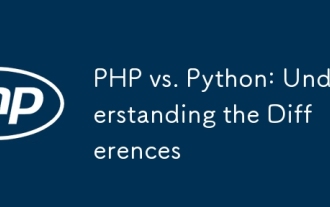
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
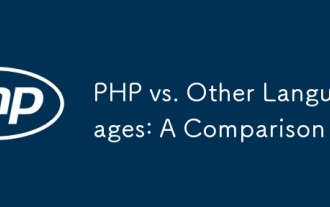
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
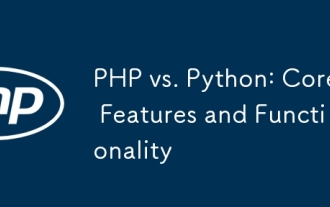
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
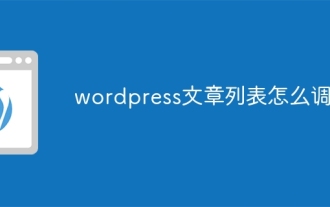
There are four ways to adjust the WordPress article list: use theme options, use plugins (such as Post Types Order, WP Post List, Boxy Stuff), use code (add settings in the functions.php file), or modify the WordPress database directly.
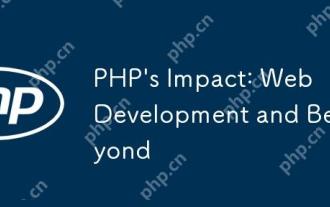
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
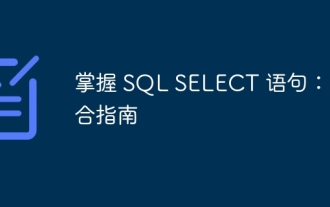
SQLSELECT statement Detailed explanation SELECT statement is the most basic and commonly used command in SQL, used to extract data from database tables. The extracted data is presented as a result set. SELECT statement syntax SELECTcolumn1,column2,...FROMtable_nameWHEREconditionORDERBYcolumn_name[ASC|DESC]; SELECT statement component selection clause (SELECT): Specify the column to be retrieved. Use * to select all columns. For example: SELECTfirst_name,last_nameFROMemployees; Source clause (FR
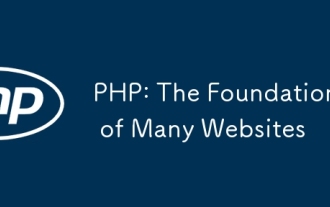
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
