


What are the common patterns for implementing distributed systems with Golang?
When building a distributed system, it is crucial to follow common patterns: Distributed Consistency: The Raft consensus algorithm is used to ensure node consistency. Load Balancing: Hash rings evenly distribute requests to groups of servers. Message Queuing: Apache Kafka for reliable and scalable event streaming. Distributed lock: Redis distributed lock enables exclusive access across nodes. Distributed transactions: Two-phase commit coordinates multi-participant atomic transaction processing. Distributed cache: Memcached can store high-performance key-value data.
Use Golang to implement common patterns of distributed systems
When building distributed systems, understand and apply common patterns to It's important. Using Golang, we can easily implement these patterns by leveraging its concurrency and parallelism features.
1. Distributed consistency
- Raft consensus algorithm: Ensures that nodes in the cluster reach consensus, even if there is a network partition.
- Example: Using etcd storage system configuration
import ( "github.com/etcd-io/etcd/clientv3" ) func main() { client, err := clientv3.New(clientv3.Config{ Endpoints: []string{"localhost:2379"}, }) if err != nil { // Handle error } defer client.Close() }
2. Load balancing
- Hash ring: Evenly distribute requests among server groups.
- Example: Using consul service discovery and load balancing
import ( "github.com/hashicorp/consul/api" ) func main() { client, err := api.NewClient(api.DefaultConfig()) if err != nil { // Handle error } // ... Register and discover services using the client }
3. Message queue
- Apache Kafka: Distributed messaging platform for reliable and scalable event streaming.
- Example: Use sarama client library to connect to Kafka cluster
import ( "github.com/Shopify/sarama" ) func main() { config := sarama.NewConfig() client, err := sarama.NewClient([]string{"localhost:9092"}, config) if err != nil { // Handle error } defer client.Close() // ... Produce and consume messages using the client }
4. Distributed lock
- Redis distributed lock: Use the atomicity feature of Redis to achieve exclusive access across nodes.
- Example: Use the redisgo library to acquire and release distributed locks
import ( "github.com/go-redis/redis/v8" ) func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", }) defer client.Close() // ... Acquire and release lock using the client }
5. Distributed transactions
- Two-Phase Commit (2PC): Coordinates multiple participants for atomic transaction processing.
- Example: Use go-tx library to implement 2PC
import ( "github.com/guregu/go-tx" ) func main() { db := tx.New(tx.Config{ Driver: "postgres", }) db.AutoCommit = false // ... Execute the two-phase commit }
6. Distributed cache
- Memcached: Distributed memory cache, used to store high-performance key-value data.
- Example: Use the go-memcached library to connect to the Memcached server
import ( "github.com/bradfitz/gomemcache/memcache" ) func main() { client := memcache.New("localhost:11211") // ... Set and get cache values using the client }
The above is the detailed content of What are the common patterns for implementing distributed systems with Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










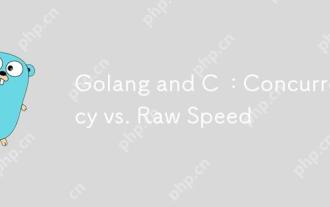
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
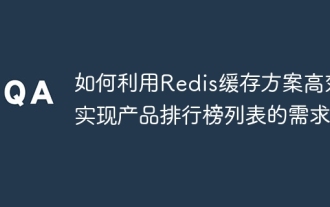
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
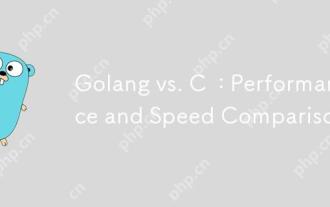
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
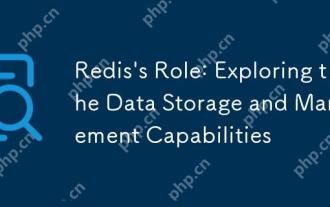
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
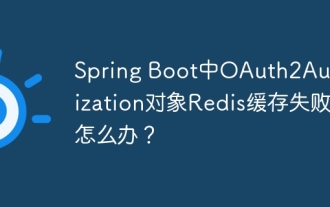
In SpringBoot, use Redis to cache OAuth2Authorization object. In SpringBoot application, use SpringSecurityOAuth2AuthorizationServer...
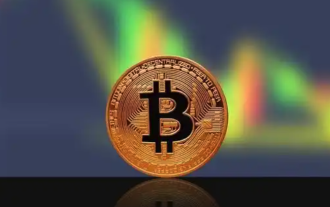
Cryptocurrency data platforms suitable for beginners include CoinMarketCap and non-small trumpet. 1. CoinMarketCap provides global real-time price, market value, and trading volume rankings for novice and basic analysis needs. 2. The non-small quotation provides a Chinese-friendly interface, suitable for Chinese users to quickly screen low-risk potential projects.
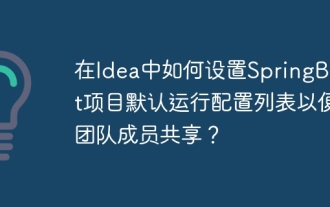
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...
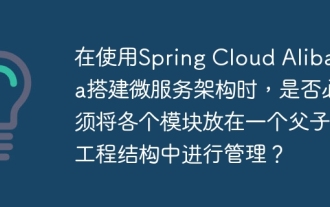
About SpringCloudAlibaba microservices modular development using SpringCloud...
