Counterexamples that violate golang function best practices
It is important to follow functional best practices. Avoid the following counterexamples: Functions that are too long Functions without docstrings Output parameters Functions with too many nested functions Return error codes instead of error values
Violation of Go language functions 5 Counterexamples to Best Practices
When writing high-quality code in Go, it is crucial to follow functional best practices. Avoiding the following counterexamples can help you write maintainable, readable, and efficient functions.
1. Overly long functions
func DoEverything(a, b int, c string, d bool) (int, error) { if c == "" { return 0, errors.New("c cannot be empty") } if !d { return 1, errors.New("d must be true") } return a + b, nil }
Best practice: Break functions into smaller, reusable functions.
2. Functions without documentation string
func DoSomething(x int) int { return x * x }
Best practice: Add a documentation string to each function to explain its purpose and parameters and return value.
3. Output Parameters
func Swap(a, b *int) { tmp := *a *a = *b *b = tmp }
Best Practice: Avoid using output parameters as it can make the code difficult to understand and debug.
4. Too many nested functions
func Nested(x int) int { if x > 10 { func inner(y int) int { return y + 1 } return inner(x) } return x }
Best practice: Move nested functions out of the main function, or use closures.
5. Functions return error codes instead of error values
func OpenFile(name string) int { f, err := os.Open(name) if err != nil { return -1 } return f.Fd() }
Best practice: According to Go language conventions, functions should return error values, instead of an error code.
Practical case
Consider the following function that needs to convert a list into a dictionary:
// 不遵循最佳实践的示例 func ConvertListToDict(list []string) map[string]bool { dict := make(map[string]bool) for _, v := range list { dict[v] = true } if len(dict) != len(list) { return nil } return dict }
This function has the following problems:
- The return value is
nil
, but the docstring does not describe this situation. - The function does not handle duplicate elements, and there is also ambiguity in returning
nil
.
Example of following best practices
// 遵循最佳实践的示例 func ConvertListToDict(list []string) (map[string]bool, error) { dict := make(map[string]bool) for _, v := range list { if _, ok := dict[v]; ok { return nil, errors.New("duplicate element in list") } dict[v] = true } return dict, nil }
This function solves the above problem and returns an error value for duplicate elements.
The above is the detailed content of Counterexamples that violate golang function best practices. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










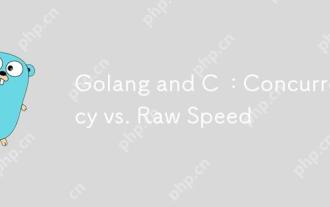
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
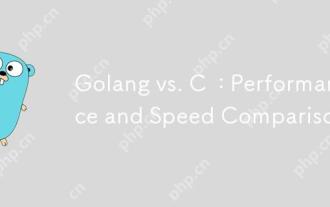
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
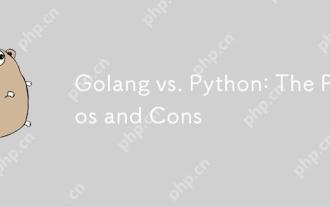
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t

IIS and PHP are compatible and are implemented through FastCGI. 1.IIS forwards the .php file request to the FastCGI module through the configuration file. 2. The FastCGI module starts the PHP process to process requests to improve performance and stability. 3. In actual applications, you need to pay attention to configuration details, error debugging and performance optimization.
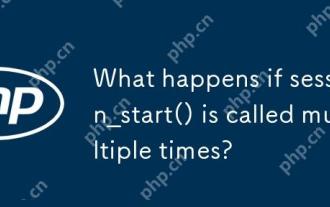
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.

AI can help optimize the use of Composer. Specific methods include: 1. Dependency management optimization: AI analyzes dependencies, recommends the best version combination, and reduces conflicts. 2. Automated code generation: AI generates composer.json files that conform to best practices. 3. Improve code quality: AI detects potential problems, provides optimization suggestions, and improves code quality. These methods are implemented through machine learning and natural language processing technologies to help developers improve efficiency and code quality.
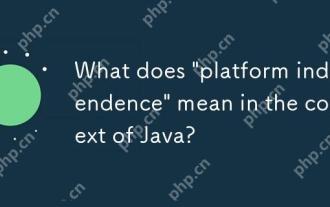
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
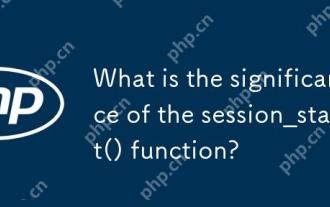
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
