Common mistakes and pitfalls of golang functional programming
Five common mistakes and pitfalls to be aware of when using functional programming in Go: Avoid accidental modification of references and ensure that newly created variables are returned. To resolve concurrency issues, use synchronization mechanisms or avoid capturing external mutable state. Use partial functionalization sparingly to improve code readability and maintainability. Always handle errors in functions to ensure the robustness of your application. Consider the performance impact and optimize your code using inline functions, flattened data structures, and batching of operations.
Common mistakes and pitfalls of functional programming in Go language
Functional programming provides a powerful toolset in the Go language , but with that comes some potential errors and pitfalls. Understanding these pitfalls and taking appropriate precautions is critical to writing robust and efficient code.
1. References are accidentally modified
In functional programming, variables within the function scope are usually regarded as immutable, but references can be modified accidentally, resulting in Unpredictable behavior. For example:
func f() []int { x := []int{1, 2, 3} return x[:2] // 返回 x 的引用 } func main() { a := f() a[1] = 10 // 修改 x 的元素,这将影响 f 中的变量 }
To avoid this, make sure the function returns a newly created variable rather than a reference to an existing variable.
2. Concurrency Issues
Functional programming often involves creating and passing closures that capture variables in outer scopes. In a concurrent context, this can lead to data races. For example:
func createCounter(n int) func() int { return func() int { n++ // 捕获外部变量 n return n } } func main() { counter := createCounter(0) // 并发调用计数器可能会导致不一致的结果 go func() { fmt.Println(counter()) }() go func() { fmt.Println(counter()) }() }
To resolve this issue, ensure that the closure does not capture or modify external mutable state, or use an appropriate synchronization mechanism when accessed in parallel.
3. Overuse of partial functionalization
Although partial functionalization can improve code reusability, overuse of it may make the code difficult to read and maintain. For example:
// 偏函数化的 add 函数 func add(x int) func(int) int { return func(y int) int { return x + y } } func main() { // 嵌套的函数调用变得难以理解 fmt.Println(add(1)(2)) fmt.Println(add(1)(3)) }
Consider using dot functions or explicit function signatures to improve code readability.
4. Forget error handling
Exception handling in functional programming is similar to imperative programming and requires careful consideration. For example:
func getLength(s string) (int, error) { if s == "" { return 0, errors.New("string is empty") } return len(s), nil } func main() { // 未处理错误,应用程序崩溃 length, _ := getLength("") fmt.Println(length) }
Always handle errors returned in functions to ensure that the application recovers gracefully even in the event of an exception.
5. Performance considerations
Functional programming operations such as map, filter, and reduce can have a significant impact on performance. To avoid unnecessary overhead, consider:
- Use inline functions or explicit loops instead of closures.
- Flatten data structures to optimize memory access.
- Batch operations to reduce the number of function calls.
By following these guidelines, you can reduce the risk of common mistakes and pitfalls in functional Go code and write more robust and efficient programs.
The above is the detailed content of Common mistakes and pitfalls of golang functional programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
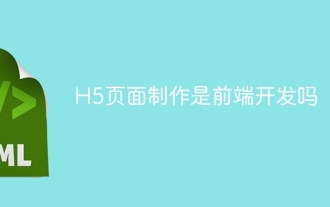
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
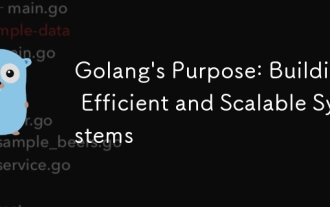
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
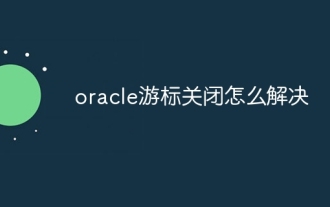
The method to solve the Oracle cursor closure problem includes: explicitly closing the cursor using the CLOSE statement. Declare the cursor in the FOR UPDATE clause so that it automatically closes after the scope is ended. Declare the cursor in the USING clause so that it automatically closes when the associated PL/SQL variable is closed. Use exception handling to ensure that the cursor is closed in any exception situation. Use the connection pool to automatically close the cursor. Disable automatic submission and delay cursor closing.
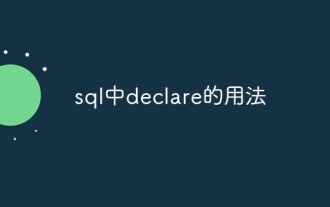
The DECLARE statement in SQL is used to declare variables, that is, placeholders that store variable values. The syntax is: DECLARE <Variable name> <Data type> [DEFAULT <Default value>]; where <Variable name> is the variable name, <Data type> is its data type (such as VARCHAR or INTEGER), and [DEFAULT <Default value>] is an optional initial value. DECLARE statements can be used to store intermediates
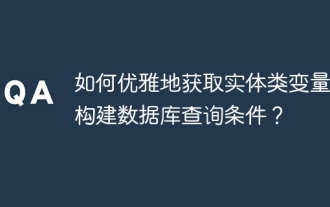
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
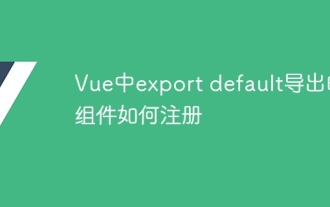
Question: How to register a Vue component exported through export default? Answer: There are three registration methods: Global registration: Use the Vue.component() method to register as a global component. Local Registration: Register in the components option, available only in the current component and its subcomponents. Dynamic registration: Use the Vue.component() method to register after the component is loaded.
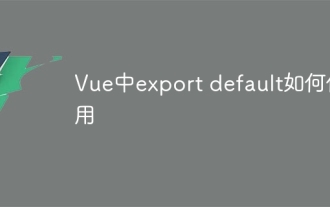
Export default in Vue reveals: Default export, import the entire module at one time, without specifying a name. Components are converted into modules at compile time, and available modules are packaged through the build tool. It can be combined with named exports and export other content, such as constants or functions. Frequently asked questions include circular dependencies, path errors, and build errors, requiring careful examination of the code and import statements. Best practices include code segmentation, readability, and component reuse.
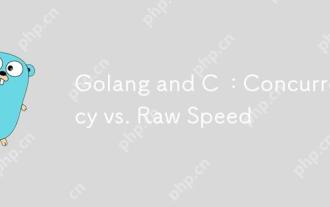
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
