How can Java functions help cloud applications integrate with IoT devices?
Java function provides a serverless way to bridge cloud applications and IoT devices. The specific steps are as follows: implement the BackgroundFunction interface to process MQTT messages, and implement the processMqttMessage method according to the actual situation. Implement the HttpFunction interface to process HTTP requests, and implement the service method according to the actual situation. Java functions are serverless, on-demand, event-driven, easy to integrate, scalable and reliable.
Java functions: A way to bridge cloud applications and IoT devices
Foreword:
As Internet of Things (IoT) devices become more prevalent, cloud applications need to be seamlessly integrated with these devices. Java functions provide an efficient way to achieve this integration, allowing developers to quickly and easily build applications that respond to and perform actions on IoT device events.
Introduction to Java Functions:
Java functions are serverless functions that can run on cloud platforms such as AWS Lambda. They are triggered on demand, eliminating the need to manage servers or virtual machines. Java functions can handle various event sources such as MQTT, HTTP requests, etc.
Practical case:
Use Java functions to process MQTT messages:
import com.google.cloud.functions.BackgroundFunction; import com.google.cloud.functions.Context; import com.google.cloud.pubsub.v1.AckReplyConsumer; import com.google.cloud.pubsub.v1.MessageReceiver; import com.google.cloud.pubsub.v1.Subscriber; import com.google.common.util.concurrent.MoreExecutors; import java.util.logging.Logger; public class MqttMessageFunction implements BackgroundFunction<byte[]> { private static final Logger logger = Logger.getLogger(MqttMessageFunction.class.getName()); @Override public void accept(byte[] data, Context context) { String message = new String(data); logger.info("Received MQTT message: " + message); try { // 模拟业务处理 processMqttMessage(message); } catch (Exception e) { logger.severe("Error processing MQTT message: " + e.getMessage()); } } private void processMqttMessage(String message) { // 在此实现实际设备消息处理逻辑 } }
In this example, MqttMessageFunction
Implements the BackgroundFunction
interface to handle MQTT messages. When a Cloud IoT Core device publishes a message, this function triggers and processes the incoming message.
Use Java functions to handle HTTP requests:
import com.google.cloud.functions.HttpFunction; import com.google.cloud.functions.HttpRequest; import com.google.cloud.functions.HttpResponse; import java.io.BufferedWriter; import java.io.IOException; import java.nio.charset.StandardCharsets; public class HttpFunctionExample implements HttpFunction { @Override public void service(HttpRequest request, HttpResponse response) throws IOException { String name = request.getFirstQueryParameter("name").orElse("world"); BufferedWriter writer = response.getWriter(); writer.write(StandardCharsets.UTF_8.name()); writer.write("Hello, " + name + "!"); } }
In this example, HttpFunctionExample
implements the HttpFunction
interface to handle HTTP request. When a user sends an HTTP request to the application, this function fires and returns the response.
Advantages:
- Serverless and on-demand: Java functions eliminate the hassle of server management and pay per use as needed.
- Event-driven: Java functions react to IoT device events and take appropriate actions.
- Easy to integrate: Java functions can be easily integrated with IoT devices using MQTT, HTTP and other protocols.
- Scalability and Reliability: Java functions automatically scale to handle more requests and provide built-in redundancy against failures.
The above is the detailed content of How can Java functions help cloud applications integrate with IoT devices?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










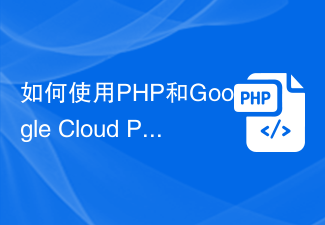
Cloud application development and deployment are an important part of modern software development. Cloud computing provides fast, flexible and scalable solutions that allow developers to develop, deploy and scale cloud applications by using PHP and Google Cloud Platform (GCP). This article will introduce how to use PHP and GCP to implement cloud application development and deployment. 1. Create GCP Projects and Instances Before you start using PHP on GCP for cloud application development, you need to create
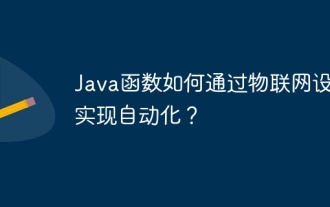
Use Java functions to connect with IoT devices and take automated actions based on their data: Create a CloudFunction project, select an HTTP trigger and a Java8 environment. Paste the provided code inside the function and it will check if the device is registered. Create a device registry that contains the virtual device, and create and download the device's JWT. Verify device identity by sending an HTTPPOST request containing a JWT to the function.
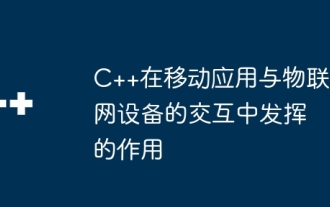
C++ plays a key role in the interaction between mobile applications and IoT devices, helping to achieve efficient and low-latency interactions: Interaction through sensor data: C++ can collect and process sensor data and send it to mobile applications. Control IoT devices: C++ can receive control commands from mobile applications and send them to IoT devices for remote control. Practical case: The home automation system demonstrates the application of C++ in building an IoT ecosystem and remotely controls home devices through mobile applications.
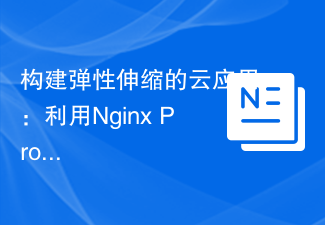
Building elastically scalable cloud applications: Using NginxProxyManager to achieve automatic expansion Introduction: With the development of cloud computing, the elastic scalability of cloud applications has become one of the focuses of enterprises. Traditional application architecture is limited to a single-machine environment and cannot meet the needs of large-scale concurrent access. In order to achieve elastic scaling, we can use NginxProxyManager to manage and automatically expand applications. This article will introduce how to use NginxProxyManager to build elastic expansion
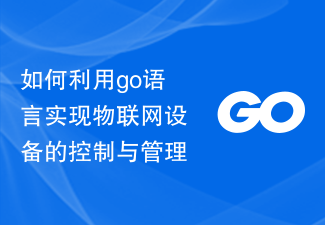
How to use go language to realize the control and management of Internet of Things devices. The Internet of Things (IoT) is a concept that has developed rapidly in recent years. It combines various sensors, smart devices and cloud computing technology to realize the interconnection and management of devices. Data interaction. As an efficient, reliable, and concurrency-friendly programming language, Go language (Golang) has great advantages in realizing the control and management of IoT devices. This article will introduce how to use Go language to control Internet of Things devices.

With the development of cloud computing technology, microservice architecture has become an important architectural model in the field of cloud applications. In the microservice architecture, the application is divided into multiple services, each service can be independently deployed and maintained independently, and can communicate through APIs. The advantage of this architectural pattern is that it improves the scalability, reliability, and maintainability of the system. This article will introduce how the microservices pattern improves the scalability of cloud applications. 1. Overview of Microservice Architecture Microservice architecture is achieved by splitting applications into smaller, more manageable
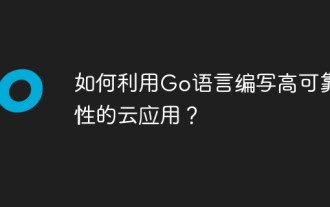
Go language (Golang) is a modern programming language known for its speed, safety and reliability. In cloud applications, high reliability is a very important indicator. Therefore, Go language has become a popular language for writing cloud applications. This article will show you how to use Go language to write high-reliability cloud applications. Using Goroutines and ChannelsGoroutines are lightweight threads in the Go language that allow you to run multiple functions simultaneously. Compared with traditional threads, Gorout
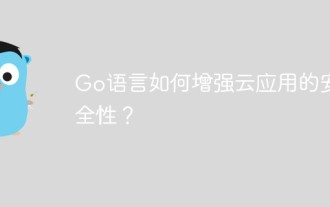
In the current cloud computing application ecosystem, the needs of cloud applications have long exceeded the simple needs of ordinary applications. From the most basic security requirements to data analysis, there are obvious differences between cloud applications and ordinary applications, so the requirements are also completely different. At the same time, as the demand for cloud computing applications grows, the security issue of cloud applications has become a more important issue. As an emerging programming language, Go language's memory management and garbage collection mechanism enable it to have better performance than other languages in the cloud environment. However, in terms of security,
