The art of solving deadlocks with golang functions
Golang provides the following functions to solve the deadlock problem: sync.Mutex: Mutex lock, ensuring that only one thread can access protected resources at the same time. sync.RWMutex: Read-write lock, which allows multiple threads to read resources at the same time, but only allows one thread to write resources.
Golang Function: The Art of Solving Deadlock
Deadlock is often encountered in concurrent programming, that is, two or more processes or threads They compete with each other for resources, causing the program to reach a deadlock. Golang provides some functions to help solve deadlock problems, and this article will introduce the most commonly used functions.
sync.Mutex
sync.Mutex
is a mutex lock, which ensures that only one thread can access protected resources at the same time. The syntax for using sync.Mutex
is as follows:
import "sync" var mu sync.Mutex func main() { mu.Lock() // 访问受保护的资源 mu.Unlock() }
In the above example, the Lock()
method blocks the thread until the lock is unlocked. Unlock()
The method releases the lock, allowing other threads to access the protected resource.
sync.RWMutex
sync.RWMutex
is a read-write lock that allows multiple threads to read resources at the same time, but only allows one thread to write to resources. The syntax for using sync.RWMutex
is as follows:
import "sync" var rwmu sync.RWMutex func main() { rwmu.RLock() // 读取受保护的资源 rwmu.RUnlock() rwmu.Lock() // 写入受保护的资源 rwmu.Unlock() }
In the above example, the RLock()
method allows multiple threads to read resources simultaneously, while The Lock()
method blocks the thread until the lock is unlocked.
Deadlock Example
The following is an example of a deadlock:
import "sync" var mu1 sync.Mutex var mu2 sync.Mutex func f1() { mu1.Lock() mu2.Lock() // ... } func f2() { mu2.Lock() mu1.Lock() // ... }
In this example, the functions f1()
and f2( )
will try to compete for two mutex locks, eventually leading to deadlock.
Prevent deadlock
To prevent deadlock, you can use the following tips:
- Avoid two threads trying to compete for the lock in the opposite order.
- Only contend for necessary locks.
- Use a timer or timeout mechanism to detect and break deadlocks.
- Use
sync.Once
to ensure that the code is executed only once.
Practical case
In a concurrent web application, we can use sync.Mutex
to protect access to the database:
import ( "database/sql" "sync" ) var db *sql.DB var dbLock sync.Mutex func init() { db, _ = sql.Open("mysql", "root:password@localhost:3306/test") } func GetUserData(userID int) (*User, error) { dbLock.Lock() defer dbLock.Unlock() // 从数据库中查询用户数据 }
By using sync.Mutex
, we can ensure that only one thread can access the database connection at the same time, thus avoiding problems such as data inconsistency that may occur when accessing the database concurrently.
The above is the detailed content of The art of solving deadlocks with golang functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
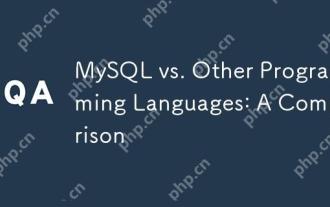
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
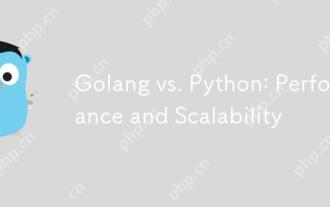
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
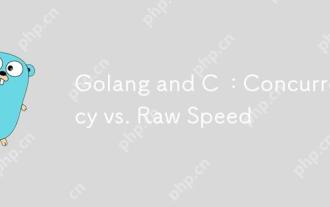
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
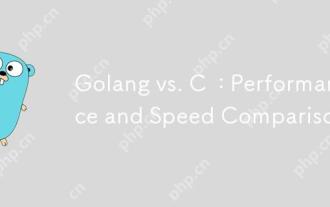
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
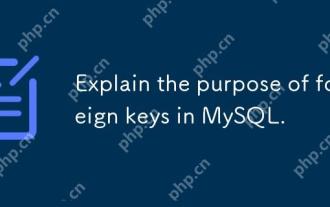
In MySQL, the function of foreign keys is to establish the relationship between tables and ensure the consistency and integrity of the data. Foreign keys maintain the effectiveness of data through reference integrity checks and cascading operations. Pay attention to performance optimization and avoid common errors when using them.
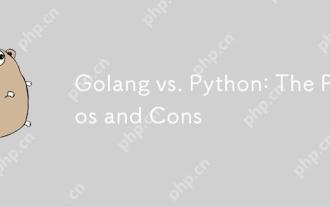
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
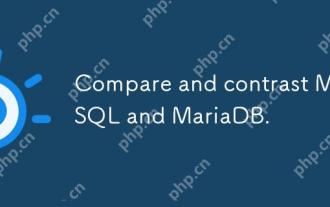
The main difference between MySQL and MariaDB is performance, functionality and license: 1. MySQL is developed by Oracle, and MariaDB is its fork. 2. MariaDB may perform better in high load environments. 3.MariaDB provides more storage engines and functions. 4.MySQL adopts a dual license, and MariaDB is completely open source. The existing infrastructure, performance requirements, functional requirements and license costs should be taken into account when choosing.
