


Are there any security implications to consider without using Java functions?
How to safely use subprocess in Python? Validate user input to prevent injection attacks. Use quotation marks to wrap commands to resist path traversal attacks. Restrict child process directory access to avoid security vulnerabilities. Use shell=False to disable execution of any shell commands.
How to use subprocess safely in Python
Introduction
In Python , the subprocess
module provides powerful functions for interacting with other processes. However, caution is required when using subprocess
to avoid security risks. This article will guide you in using subprocess
safely and provide a practical case.
Security Hazards
The main security risks of using subprocess
include:
- Injection attacks: Unvalidated user input may be injected into the command, thereby executing malicious code.
-
Directory Traversal Attack: The path passed to
subprocess
can be manipulated to access sensitive files or directories.
Security Practices
To mitigate these security risks, follow these best practices:
-
Validate input :Always validate user input to ensure it does not contain malicious characters. You can use Python's built-in functions, such as
str.isalnum()
. - Use quotes: Use quotes to wrap commands to prevent path traversal attacks.
-
Restrict path access: By setting the
cwd
parameter, limit the directories that child processes can access. -
Use shell=False: Avoid using
shell=True
as it allows arbitrary shell commands to be executed.
Practical case
Suppose you want to safely use subprocess
to execute a Linux command, such as ls -l
. Here is a sample code:
import subprocess # 验证输入 input_dir = input("请输入要列出的目录:") if not input_dir.isalnum(): print("无效目录名") exit(1) # 使用引号和限制路径访问 command = f"ls -l '{input_dir}' --color=auto" result = subprocess.run(command, shell=False, stdout=subprocess.PIPE) # 处理结果 if result.returncode == 0: print(result.stdout.decode()) else: print(f"错误:{result.stderr.decode()}")
In this example, the user input is validated before using the isalnum()
function. The command is wrapped in quotes, and cwd
is set to the current working directory to restrict file access by the child process.
Conclusion
By following these best practices, you can safely use the subprocess
module in Python. Always keep potential safety hazards in mind and take steps to mitigate them.
The above is the detailed content of Are there any security implications to consider without using Java functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











Docker is important on Linux because Linux is its native platform that provides rich tools and community support. 1. Install Docker: Use sudoapt-getupdate and sudoapt-getinstalldocker-cedocker-ce-clicotainerd.io. 2. Create and manage containers: Use dockerrun commands, such as dockerrun-d--namemynginx-p80:80nginx. 3. Write Dockerfile: Optimize the image size and use multi-stage construction. 4. Optimization and debugging: Use dockerlogs and dockerex

IIS and PHP are compatible and are implemented through FastCGI. 1.IIS forwards the .php file request to the FastCGI module through the configuration file. 2. The FastCGI module starts the PHP process to process requests to improve performance and stability. 3. In actual applications, you need to pay attention to configuration details, error debugging and performance optimization.
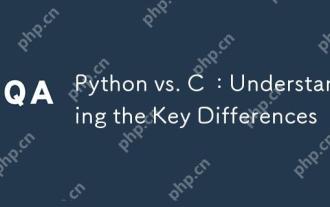
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
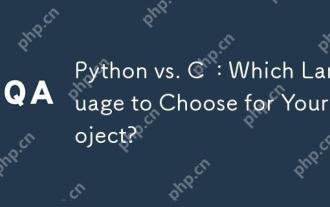
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
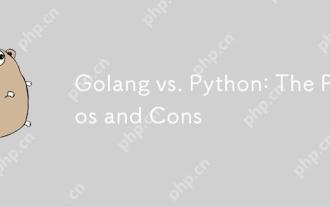
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
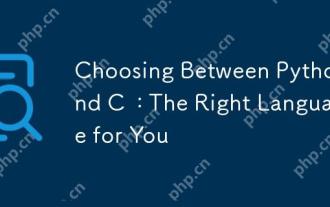
Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.

Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.
