Best practices and design patterns for Golang function libraries
Go function library design best practices include: modularization, clear interfaces, clear documentation, error handling, and careful use of type assertions. Commonly used design patterns include: singleton pattern (ensuring a single instance), factory pattern (creating object variants), and dependency injection (decoupling components). Following these principles can create a library that is modular, maintainable, extensible, and less error-prone.
Best practices and design patterns for Golang function libraries
In Go programming, a function library is a way to group related functions and provide a consistent interface Powerful tool. Use best practices and design patterns to create libraries that are modular, maintainable, and extensible.
Best Practices:
- Keep modular: Organize related functions into subpackages to improve code readability and manageability.
- Define clear interfaces: Use interface types to define the public API of the function library, allowing users to rely on the function library without knowing the implementation details.
-
Provide documentation: Use
//
comments or Go docstrings (///
) to provide documentation to help developers understand the functions Purpose, parameters and return values. - Handling errors: When possible, return an explicit error object with a detailed error message.
- Use type assertions: Use type assertions with caution as they may cause runtime errors.
Design pattern:
Singleton pattern:
- Ensure that there is only one instance of a specific object in the function library.
- Avoid resource waste and object conflicts.
-
Example:
package main import "sync" type Single struct { sync.Mutex instance *Single } func (s *Single) Instance() *Single { s.Lock() defer s.Unlock() if s.instance == nil { s.instance = &Single{} } return s.instance } func main() { instance1 := Single{}.Instance() instance2 := Single{}.Instance() fmt.Println(instance1 == instance2) // true }
Copy after login
Factory pattern:
- Create objects Multiple variants without exposing the creation logic to the client.
- Improve code flexibility, allowing new variants to be added without changing client code.
Example:
package main type Animal interface { GetName() string } type Cat struct { name string } func (c *Cat) GetName() string { return c.name } type Dog struct { name string } func (d *Dog) GetName() string { return d.name } type AnimalFactory struct {} func (f *AnimalFactory) CreateAnimal(animalType string, name string) Animal { switch animalType { case "cat": return &Cat{name} case "dog": return &Dog{name} default: return nil } } func main() { factory := &AnimalFactory{} cat := factory.CreateAnimal("cat", "Meow") dog := factory.CreateAnimal("dog", "Buddy") fmt.Println(cat.GetName()) // Meow fmt.Println(dog.GetName()) // Buddy }
Copy after login
Dependency Injection:
- Through functions Constructors or interfaces provide dependencies to objects.
- Decouple components to improve testability and maintainability.
Example:
package main import "fmt" type Logger interface { Log(msg string) } type Database interface { Get(id int) interface{} } type Service struct { Logger Database } func NewService(logger Logger, db Database) *Service { return &Service{logger, db} } func main() { logger := ConsoleLogger{} db := MockDatabase{} service := NewService(logger, db) service.Log("Hello world") fmt.Println(service.Get(1)) } type ConsoleLogger struct {} func (ConsoleLogger) Log(msg string) { fmt.Println(msg) } type MockDatabase struct {} func (MockDatabase) Get(id int) interface{} { return id }
Copy after login
By following these best practices and adopting design patterns, you can create powerful, scalable Golang function library makes code easier to maintain, more flexible, and reduces errors.
The above is the detailed content of Best practices and design patterns for Golang function libraries. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










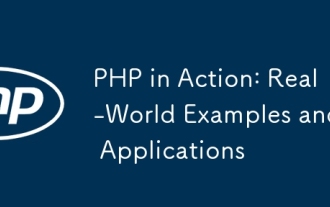
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
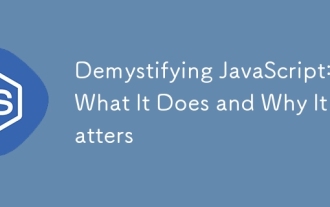
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
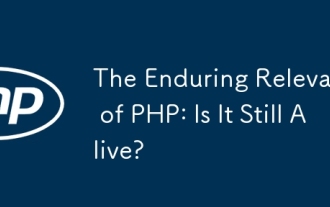
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
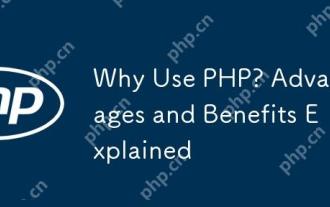
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
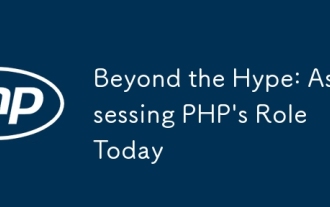
PHP remains a powerful and widely used tool in modern programming, especially in the field of web development. 1) PHP is easy to use and seamlessly integrated with databases, and is the first choice for many developers. 2) It supports dynamic content generation and object-oriented programming, suitable for quickly creating and maintaining websites. 3) PHP's performance can be improved by caching and optimizing database queries, and its extensive community and rich ecosystem make it still important in today's technology stack.
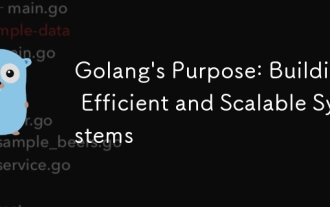
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
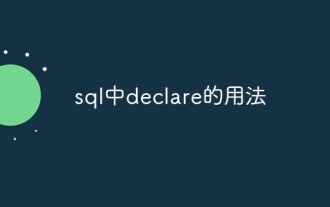
The DECLARE statement in SQL is used to declare variables, that is, placeholders that store variable values. The syntax is: DECLARE <Variable name> <Data type> [DEFAULT <Default value>]; where <Variable name> is the variable name, <Data type> is its data type (such as VARCHAR or INTEGER), and [DEFAULT <Default value>] is an optional initial value. DECLARE statements can be used to store intermediates
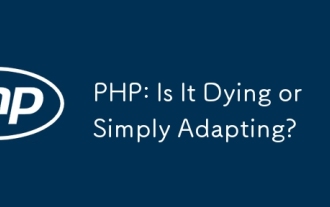
PHP is not dying, but constantly adapting and evolving. 1) PHP has undergone multiple version iterations since 1994 to adapt to new technology trends. 2) It is currently widely used in e-commerce, content management systems and other fields. 3) PHP8 introduces JIT compiler and other functions to improve performance and modernization. 4) Use OPcache and follow PSR-12 standards to optimize performance and code quality.
