


How does Java database connection use batch processing to improve performance?
Using batch processing technology, you can significantly improve the performance of database operations. The specific steps are as follows: Connect to the database and use Connection.prepareBatch() to create a batch statement. To add statements to be executed to a batch statement, use stmt.addBatch(). Use stmt.executeBatch() to execute statements in batches. Use stmt.close() to close the batch statement.
Java Database Connection: Utilizing Batch Processing to Improve Performance
Batch processing is a database operation technology that allows multiple database statements to be executed at one time. By using batch processing, you can significantly improve the performance of database operations. In this article, we will introduce how to implement batch processing in Java using JDBC.
Connect to the database
First, you need to connect to the database. The following code shows how to connect to a MySQL database using JDBC:
import java.sql.Connection; import java.sql.DriverManager; public class DatabaseConnection { public static void main(String[] args) throws Exception { // 数据库连接信息 String url = "jdbc:mysql://localhost:3306/database"; String user = "username"; String password = "password"; // 加载JDBC驱动 Class.forName("com.mysql.cj.jdbc.Driver"); // 获取数据库连接 Connection conn = DriverManager.getConnection(url, user, password); // ...(在连接上执行其他操作) // 关闭连接 conn.close(); } }
Batch
To create a batch statement, you can use the Connection.prepareBatch()
method. This returns a PreparedStatement
object that you can use to add statements to execute:
import java.sql.PreparedStatement; public class DatabaseBatch { public static void main(String[] args) throws Exception { // ...(数据库连接已建立) // 创建批处理语句 PreparedStatement stmt = conn.prepareBatch(); // 添加要执行的语句 stmt.addBatch("INSERT INTO table (column1, column2) VALUES (?, ?)"); stmt.addBatch("UPDATE table SET column1 = ? WHERE id = ?"); stmt.addBatch("DELETE FROM table WHERE id = ?"); // 执行批处理 stmt.executeBatch(); // 关闭批处理语句 stmt.close(); } }
By using batching, you can group multiple database operations into a single batch statement , and execute them in batches at once. This can greatly reduce the number of interactions with the database, thereby improving performance.
Practical Case
The following is a practical case using batch processing to insert a large amount of data into the database:
import java.sql.Connection; import java.sql.PreparedStatement; public class DatabaseBatchInsert { public static void main(String[] args) throws Exception { // ...(数据库连接已建立) // 创建批处理语句 PreparedStatement stmt = conn.prepareBatch(); // 插入大量数据 for (int i = 0; i < 100000; i++) { stmt.addBatch("INSERT INTO table (column1, column2) VALUES (?, ?)"); stmt.setInt(1, i); stmt.setString(2, "value" + i); } // 执行批处理 stmt.executeBatch(); // 关闭批处理语句 stmt.close(); } }
Using batch processing, the performance of this insertion operation will be better than executing it one by one. Each insert statement is much faster.
The above is the detailed content of How does Java database connection use batch processing to improve performance?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
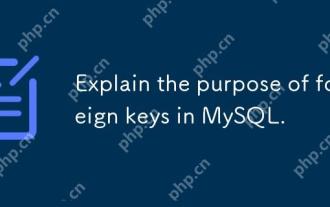
In MySQL, the function of foreign keys is to establish the relationship between tables and ensure the consistency and integrity of the data. Foreign keys maintain the effectiveness of data through reference integrity checks and cascading operations. Pay attention to performance optimization and avoid common errors when using them.
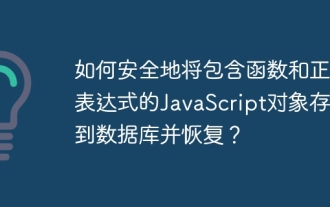
Safely handle functions and regular expressions in JSON In front-end development, JavaScript is often required...
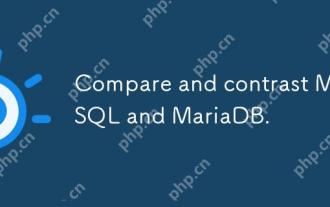
The main difference between MySQL and MariaDB is performance, functionality and license: 1. MySQL is developed by Oracle, and MariaDB is its fork. 2. MariaDB may perform better in high load environments. 3.MariaDB provides more storage engines and functions. 4.MySQL adopts a dual license, and MariaDB is completely open source. The existing infrastructure, performance requirements, functional requirements and license costs should be taken into account when choosing.

SQL is a standard language for managing relational databases, while MySQL is a database management system that uses SQL. SQL defines ways to interact with a database, including CRUD operations, while MySQL implements the SQL standard and provides additional features such as stored procedures and triggers.
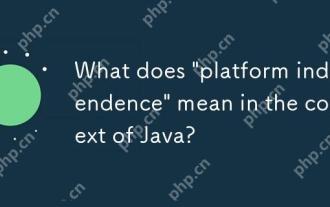
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
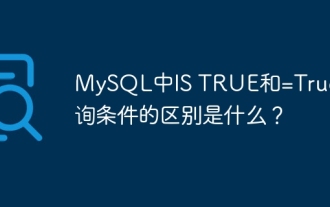
The difference between ISTRUE and =True query conditions in MySQL In MySQL database, when processing Boolean values (Booleans), ISTRUE and =TRUE...

Choosing MySQL or Oracle depends on project requirements: 1. MySQL is suitable for small and medium-sized applications and Internet projects because of its open source, free and ease of use; 2. Oracle is suitable for core business systems of large enterprises because of its powerful, stable and advanced functions, but at a high cost.
