Application of PHP functions in web development
PHP functions are indispensable in web development, providing convenient methods for common tasks: Basic functions: Manipulate strings, numbers, and arrays. File handling: reading, writing and deleting files. Database operations: connecting, querying and retrieving data. For example, in the scenario of getting MySQL user data and displaying it on a web page, PHP functions are used to connect to the database, execute the query, get the results and output them into an HTML list.
Application of PHP functions in Web development
Introduction
PHP ( Hypertext Preprocessor) is a server-side programming language widely used in Web development. It provides a rich library of functions that simplify common web development tasks.
Basic functions
- echo(), print(): Output data
- strlen( ), substr(): Processing string length and substring
- number_format(), round(): Formatting numbers
- array_merge() , array_keys(): Operation array
File processing
- file_get_contents(): Read file contents
- file_put_contents(): Write file contents
- unlink(): Delete file
Database operations
- mysqli_connect(): Connect to MySQL database
- mysqli_query(): Execute SQL query
- mysqli_fetch_assoc(): Get the associative array of query results
Practical case
Consider the following scenario: There is a saved user MySQL table of data. We wrote a PHP script to get all the records in the table and print them on a web page.
<?php // 连接到数据库 $servername = "localhost"; $username = "root"; $password = ""; $dbname = "users"; // 创建数据库连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败:" . $conn->connect_error); } // 执行 SQL 查询 $sql = "SELECT * FROM users"; $result = $conn->query($sql); // 获取查询结果 $users = array(); while ($row = $result->fetch_assoc()) { array_push($users, $row); } // 关闭连接 $conn->close(); // 输出查询结果 echo "<ul>"; foreach ($users as $user) { echo "<li>" . $user['name'] . " (" . $user['email'] . ")</li>"; } echo "</ul>"; ?>
Conclusion
PHP functions are crucial for web development as it provides various convenient methods to perform common tasks. From basic string processing to database operations, PHP functions help simplify complex processes and improve development efficiency.
The above is the detailed content of Application of PHP functions in web development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











MySQL and phpMyAdmin can be effectively managed through the following steps: 1. Create and delete database: Just click in phpMyAdmin to complete. 2. Manage tables: You can create tables, modify structures, and add indexes. 3. Data operation: Supports inserting, updating, deleting data and executing SQL queries. 4. Import and export data: Supports SQL, CSV, XML and other formats. 5. Optimization and monitoring: Use the OPTIMIZETABLE command to optimize tables and use query analyzers and monitoring tools to solve performance problems.

AI can help optimize the use of Composer. Specific methods include: 1. Dependency management optimization: AI analyzes dependencies, recommends the best version combination, and reduces conflicts. 2. Automated code generation: AI generates composer.json files that conform to best practices. 3. Improve code quality: AI detects potential problems, provides optimization suggestions, and improves code quality. These methods are implemented through machine learning and natural language processing technologies to help developers improve efficiency and code quality.
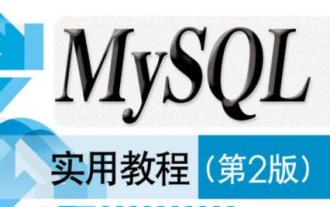
In MySQL, add fields using ALTERTABLEtable_nameADDCOLUMNnew_columnVARCHAR(255)AFTERexisting_column, delete fields using ALTERTABLEtable_nameDROPCOLUMNcolumn_to_drop. When adding fields, you need to specify a location to optimize query performance and data structure; before deleting fields, you need to confirm that the operation is irreversible; modifying table structure using online DDL, backup data, test environment, and low-load time periods is performance optimization and best practice.
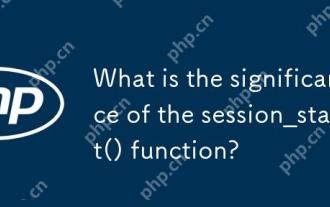
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
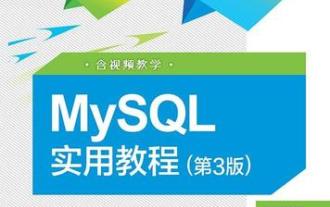
To safely and thoroughly uninstall MySQL and clean all residual files, follow the following steps: 1. Stop MySQL service; 2. Uninstall MySQL packages; 3. Clean configuration files and data directories; 4. Verify that the uninstallation is thorough.

H5 brings a number of new functions and capabilities, greatly improving the interactivity and development efficiency of web pages. 1. Semantic tags such as enhance SEO. 2. Multimedia support simplifies audio and video playback through and tags. 3. Canvas drawing provides dynamic graphics drawing tools. 4. Local storage simplifies data storage through localStorage and sessionStorage. 5. The geolocation API facilitates the development of location-based services.
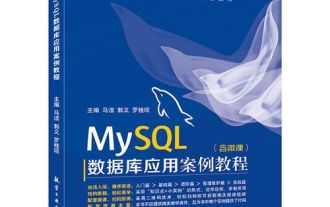
Efficient methods for batch inserting data in MySQL include: 1. Using INSERTINTO...VALUES syntax, 2. Using LOADDATAINFILE command, 3. Using transaction processing, 4. Adjust batch size, 5. Disable indexing, 6. Using INSERTIGNORE or INSERT...ONDUPLICATEKEYUPDATE, these methods can significantly improve database operation efficiency.
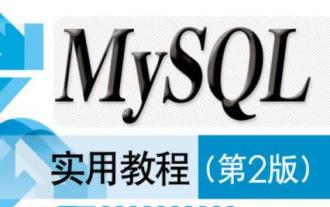
MySQL functions can be used for data processing and calculation. 1. Basic usage includes string processing, date calculation and mathematical operations. 2. Advanced usage involves combining multiple functions to implement complex operations. 3. Performance optimization requires avoiding the use of functions in the WHERE clause and using GROUPBY and temporary tables.
