


Disadvantages of Golang functions and limitations in functional programming?
Disadvantages of functions in Go: First-class functions are not supported and functions cannot be passed as parameters or return values. Lack of lazy evaluation, the inability to defer the evaluation of an expression. Limits function composition, making it more difficult to write certain functional programming patterns in Go.
Disadvantages of Go functions: Limitations in functional programming
In Go, functions are both values and types. This means they can be passed around, assigned, and compared like any other value. However, this flexibility also brings limitations to functional programming in Go.
No support for first-class functions
Unlike many other languages that support functional programming, Go does not support first-class functions. This means that functions cannot be used as arguments or return values of other functions. This limits the options available for combining functions.
Code example:
In C#, we can use first-class functions to create an anonymous function and pass it as a parameter to another function:
using System; namespace CSharpExample { class Program { static void Main(string[] args) { Func<int, int> square = x => x * x; PrintSquare(square, 5); } static void PrintSquare(Func<int, int> square, int number) { Console.WriteLine(square(number)); } } }
But in Go, since Go does not support first-class functions, the same code cannot be used:
package main import "fmt" func main() { square := func(x int) int { return x * x } printSquare(square, 5) // 编译错误 } func printSquare(f int) int { return f(25) }
No lazy evaluation
The other side of functional programming An important feature is lazy evaluation, which allows the evaluation of an expression to be postponed until needed. However, Go lacks native support for lazy evaluation.
Code example:
In Haskell, we can use lazy evaluation to define an infinite list:
lazy fibs = 0 : 1 : zipWith (+) fibs (tail fibs)
But in Go, due to its The lack of lazy evaluation prevents the use of the same code:
func fibs() []int { a, b := 0, 1 for { a, b = b, a+b yield(a) } }
limits function composition
The lack of first-class functions and lazy evaluation limits the function as a Combine as a whole. This makes it harder to write some functional programming patterns in Go.
Conclusion
While Go provides flexibility for functions, it also introduces limitations that prevent the full use of functional programming. Ways to work around these limitations are still being explored, but so far the disadvantage of Go functions remains their limitations as a functional programming language.
The above is the detailed content of Disadvantages of Golang functions and limitations in functional programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










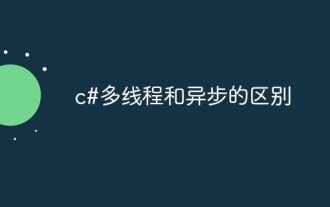
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
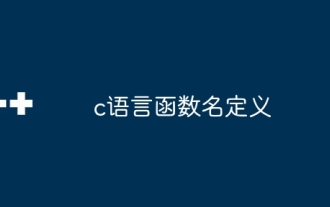
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
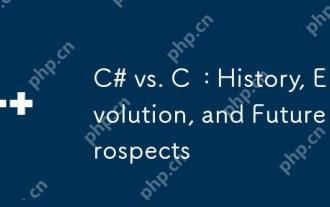
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
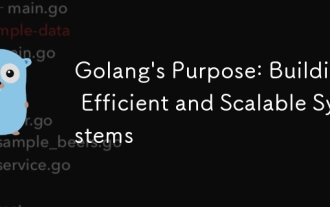
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
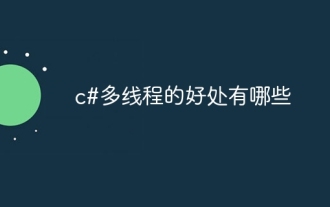
The advantage of multithreading is that it can improve performance and resource utilization, especially for processing large amounts of data or performing time-consuming operations. It allows multiple tasks to be performed simultaneously, improving efficiency. However, too many threads can lead to performance degradation, so you need to carefully select the number of threads based on the number of CPU cores and task characteristics. In addition, multi-threaded programming involves challenges such as deadlock and race conditions, which need to be solved using synchronization mechanisms, and requires solid knowledge of concurrent programming, weighing the pros and cons and using them with caution.
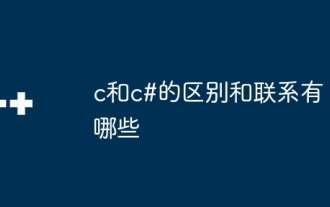
Although C and C# have similarities, they are completely different: C is a process-oriented, manual memory management, and platform-dependent language used for system programming; C# is an object-oriented, garbage collection, and platform-independent language used for desktop, web application and game development.
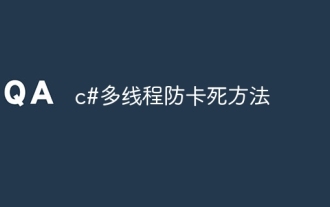
The following ways to avoid "stuck" multithreading in C#: avoid time-consuming operations on UI threads. Use Task and async/await to perform time-consuming operations asynchronously. Update the UI on the UI thread via Application.Current.Dispatcher.Invoke. Use the CancellationToken to control task cancellation. Make rational use of thread pools to avoid excessive creation of threads. Pay attention to code readability and maintainability, making it easy to debug. Logs are recorded in each thread for easy debugging.
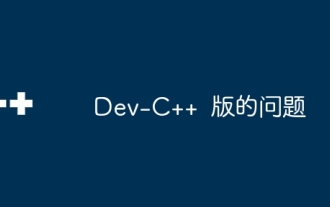
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
