Synergy between HTML and database queries
HTML complements database queries, enabling the construction of interactive and data-driven web applications: HTML form processing: collects user input and retrieves data from the database, responding to user actions. AJAX data request: Send database query asynchronously, update data without refreshing the page. Database-driven search functionality: The user enters a query and the application uses SQL to query the database to return relevant results.
The synergy of HTML and database queries
Introduction
HTML and databases Queries complement each other and help us build interactive and data-driven web applications. This article explores how to combine HTML with database queries and provides some practical examples.
1. HTML form processing
HTML forms can be used to collect user input. We can use backend languages like PHP, Python or Node.js to process these forms and retrieve the required data from the database in response.
Code Example:
<!-- HTML 表单 --> <form action="process.php" method="post"> <input type="text" name="name" placeholder="姓名"> <input type="email" name="email" placeholder="电子邮箱"> <input type="submit" value="提交"> </form> <!-- PHP 表单处理 --> <?php // 从表单中获取数据 $name = $_POST['name']; $email = $_POST['email']; // 连接数据库 $conn = new mysqli('localhost', 'root', '', 'mydb'); // 准备 SQL 查询 $stmt = $conn->prepare("SELECT * FROM users WHERE name=?"); $stmt->bind_param('s', $name); // 执行查询并获取结果 $stmt->execute(); $result = $stmt->get_result(); // 遍历结果并显示用户数据 while ($row = $result->fetch_assoc()) { echo "姓名:" . $row['name'] . "<br>"; echo "电子邮箱:" . $row['email'] . "<br>"; } ?>
2. AJAX Data Request
AJAX (Asynchronous JavaScript and XML) can be used to request data to the database Send a query without refreshing the entire page. This allows us to update data without disrupting the user experience.
Code example:
<!-- HTML 页面 --> <div id="data"></div> <!-- JavaScript AJAX 调用 --> <script> // 创建 XMLHttpRequest 对象 var xhr = new XMLHttpRequest(); // 设置请求方法和 URL xhr.open('GET', 'get_data.php'); // 发送请求 xhr.send(); // 处理响应 xhr.onload = function() { if (xhr.status == 200) { var data = JSON.parse(xhr.responseText); // 使用接收到的数据更新 HTML 元素 document.getElementById('data').innerHTML = data.message; } }; </script> <!-- PHP 获取数据 --> <?php // 连接数据库 $conn = new mysqli('localhost', 'root', '', 'mydb'); // 准备 SQL 查询 $stmt = $conn->prepare("SELECT * FROM messages"); // 执行查询并获取结果 $stmt->execute(); $result = $stmt->get_result(); // 将结果编码为 JSON 并返回 $messages = []; while ($row = $result->fetch_assoc()) { $messages[] = $row; } echo json_encode(['message' => $messages]); ?>
3. Database-driven search function
We can combine HTML and database queries to build a database Driver search function. The user enters a query and the application uses SQL to query the database and return relevant results.
Code Example:
<!-- HTML 搜索栏 --> <input type="text" id="search"> <!-- JavaScript 搜索处理 --> <script> // 获取搜索栏输入 var searchTerm = document.getElementById('search').value; // 使用 AJAX 发送查询 var xhr = new XMLHttpRequest(); xhr.open('GET', 'search.php?q=' + searchTerm); xhr.send(); // 处理响应 xhr.onload = function() { if (xhr.status == 200) { var results = JSON.parse(xhr.responseText); // 使用接收到的结果更新 HTML 元素 // ... } }; </script> <!-- PHP 搜索 --> <?php // 获取搜索查询 $q = $_GET['q']; // 连接数据库 $conn = new mysqli('localhost', 'root', '', 'mydb'); // 准备 SQL 查询 $stmt = $conn->prepare("SELECT * FROM products WHERE name LIKE ?"); $stmt->bind_param('s', $q); // 执行查询并获取结果 $stmt->execute(); $result = $stmt->get_result(); // 将结果编码为 JSON 并返回 $products = []; while ($row = $result->fetch_assoc()) { $products[] = $row; } echo json_encode(['results' => $products]); ?>
Conclusion
HTML works with database queries to provide the ability to build interactive and data-driven Powerful tools for web applications. By integrating these technologies, we can create dynamic and interactive pages that can fetch, process and display data from the database.
The above is the detailed content of Synergy between HTML and database queries. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.

HTML, CSS and JavaScript are the core technologies for building modern web pages: 1. HTML defines the web page structure, 2. CSS is responsible for the appearance of the web page, 3. JavaScript provides web page dynamics and interactivity, and they work together to create a website with a good user experience.
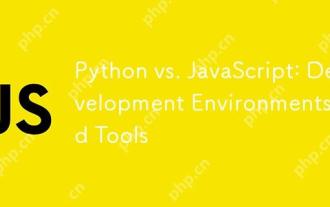
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
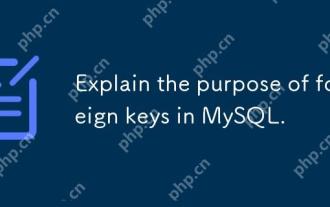
In MySQL, the function of foreign keys is to establish the relationship between tables and ensure the consistency and integrity of the data. Foreign keys maintain the effectiveness of data through reference integrity checks and cascading operations. Pay attention to performance optimization and avoid common errors when using them.
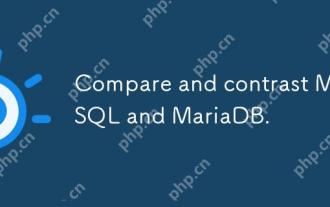
The main difference between MySQL and MariaDB is performance, functionality and license: 1. MySQL is developed by Oracle, and MariaDB is its fork. 2. MariaDB may perform better in high load environments. 3.MariaDB provides more storage engines and functions. 4.MySQL adopts a dual license, and MariaDB is completely open source. The existing infrastructure, performance requirements, functional requirements and license costs should be taken into account when choosing.

SQL is a standard language for managing relational databases, while MySQL is a database management system that uses SQL. SQL defines ways to interact with a database, including CRUD operations, while MySQL implements the SQL standard and provides additional features such as stored procedures and triggers.

MySQL and phpMyAdmin can be effectively managed through the following steps: 1. Create and delete database: Just click in phpMyAdmin to complete. 2. Manage tables: You can create tables, modify structures, and add indexes. 3. Data operation: Supports inserting, updating, deleting data and executing SQL queries. 4. Import and export data: Supports SQL, CSV, XML and other formats. 5. Optimization and monitoring: Use the OPTIMIZETABLE command to optimize tables and use query analyzers and monitoring tools to solve performance problems.
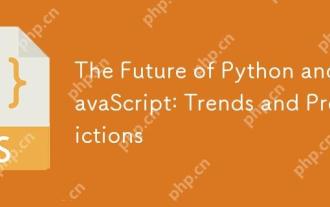
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
