HTML paragraphs are automatically indented by two spaces
The method to use Python and BeautifulSoup to parse HTML documents is as follows: load the HTML document and create a BeautifulSoup object. Use BeautifulSoup objects to find and process tag elements, such as: Find a specific tag: soup.find(tag_name) Find all specific tags: soup.find_all(tag_name) Find tags with specific attributes: soup.find(tag_name, {'attribute': 'value'}) extracts the text content or attribute value of the label. Adjust the code as needed to obtain specific information.
Parsing HTML documents using Python and BeautifulSoup
Objective:
Learn how to parse HTML documents using Python and the BeautifulSoup library.
Required knowledge:
- Python basics
- HTML and XML knowledge
##Code :
from bs4 import BeautifulSoup # 加载 HTML 文档 html_doc = """ <html> <head> <title>HTML 文档</title> </head> <body> <h1>标题</h1> <p>段落</p> </body> </html> """ # 创建 BeautifulSoup 对象 soup = BeautifulSoup(html_doc, 'html.parser') # 获取标题标签 title_tag = soup.find('title') print(title_tag.text) # 输出:HTML 文档 # 获取所有段落标签 paragraph_tags = soup.find_all('p') for paragraph in paragraph_tags: print(paragraph.text) # 输出:段落 # 获取特定属性的值 link_tag = soup.find('link', {'rel': 'stylesheet'}) print(link_tag['href']) # 输出:样式表链接
Practical case: A simple practical case is a crawler that uses BeautifulSoup to extract specified information from a web page. For example, you can use the following code to pull questions and answers from Stack Overflow:
import requests from bs4 import BeautifulSoup url = 'https://stackoverflow.com/questions/31207139/using-beautifulsoup-to-extract-specific-attribute' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') questions = soup.find_all('div', {'class': 'question-summary'}) for question in questions: question_title = question.find('a', {'class': 'question-hyperlink'}).text question_body = question.find('div', {'class': 'question-snippet'}).text print(f'问题标题:{question_title}') print(f'问题内容:{question_body}') print('---')
The above is the detailed content of HTML paragraphs are automatically indented by two spaces. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











IIS and PHP are compatible and are implemented through FastCGI. 1.IIS forwards the .php file request to the FastCGI module through the configuration file. 2. The FastCGI module starts the PHP process to process requests to improve performance and stability. 3. In actual applications, you need to pay attention to configuration details, error debugging and performance optimization.
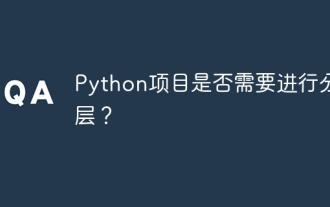
Discussion on Hierarchical Structure in Python Projects In the process of learning Python, many beginners will come into contact with some open source projects, especially projects using the Django framework...
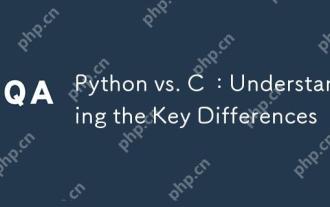
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.
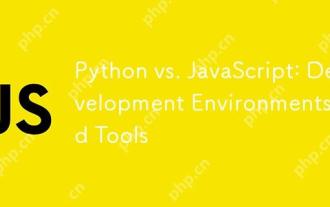
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
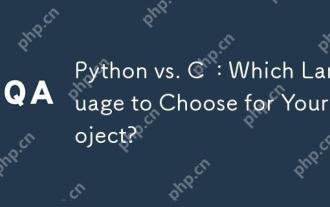
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
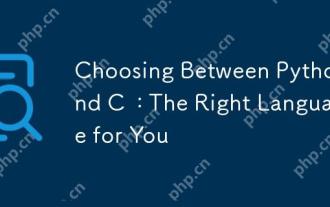
Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
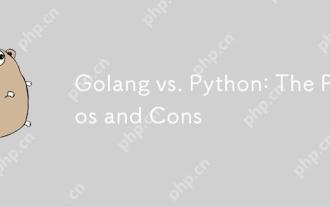
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
