Mastering Go: Anatomy of a POST Request
In the Go language, parsing a POST request is divided into the following steps: Use ParseForm() to parse form data. Use FormValue() to get the value of a specific field. Use the encoding/json package to parse JSON data. Use json.Unmarshal() to parse JSON data into Go structures.
Proficient in the Go language: Parsing POST requests
The POST request is an HTTP method used to submit data to the server. In the Go language, the process of parsing a POST request is simple.
Parsing form data
The most common type of POST request is form data. Here's how to parse form data:
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { if r.Method == "POST" { r.ParseForm() name := r.FormValue("name") email := r.FormValue("email") fmt.Fprintf(w, "Name: %s\nEmail: %s", name, email) } }) http.ListenAndServe(":8080", nil) }
In the above example, we use the ParseForm()
function to parse the form data. We can then use the FormValue()
function to get the value of a specific field.
Parsing JSON data
Another common POST request type is JSON data. Here's how to parse JSON data:
package main import ( "encoding/json" "fmt" "io/ioutil" "net/http" ) type User struct { Name string `json:"name"` Email string `json:"email"` } func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { if r.Method == "POST" { bodyBytes, _ := ioutil.ReadAll(r.Body) var user User json.Unmarshal(bodyBytes, &user) fmt.Fprintf(w, "Name: %s\nEmail: %s", user.Name, user.Email) } }) http.ListenAndServe(":8080", nil) }
In the above example, we use the encoding/json
package to parse JSON data into Go's structs. This allows us to easily access individual fields of the requested data.
Practical Cases
The following are some practical cases showing how to use Go language to parse POST requests:
- Using form data for user registration
- Use JSON data to update user information
- Use POST request to submit file upload
The above is the detailed content of Mastering Go: Anatomy of a POST Request. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
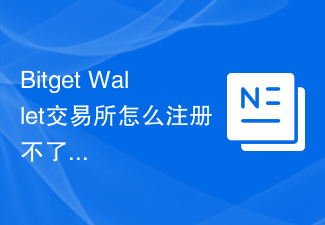
There are various reasons for being unable to register for the BitgetWallet exchange, including account restrictions, unsupported regions, network issues, system maintenance and technical failures. To register for the BitgetWallet exchange, please visit the official website, fill in the information, agree to the terms, complete registration and verify your identity.
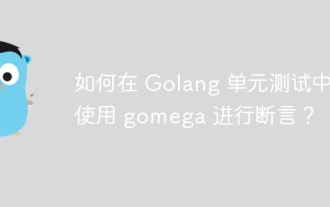
How to use Gomega for assertions in Golang unit testing In Golang unit testing, Gomega is a popular and powerful assertion library that provides rich assertion methods so that developers can easily verify test results. Install Gomegagoget-ugithub.com/onsi/gomega Using Gomega for assertions Here are some common examples of using Gomega for assertions: 1. Equality assertion import "github.com/onsi/gomega" funcTest_MyFunction(t*testing.T){

DeepSeek's official website is now launching multiple discount activities to provide users with a shopping experience. New users sign up to get a $10 coupon, and enjoy a 15% limited time discount for the entire audience. Recommend friends can also earn rewards, and you can accumulate points for redemption of gifts when shopping. The event deadlines are different. For details, please visit the DeepSeek official website for inquiries.
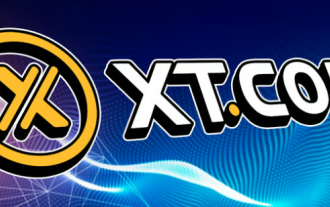
Mainland users can register on the XT.COM exchange through the following steps: Visit the XT.COM official website. Click the "Register" button in the upper right corner. Select the "Mobile Registration" option. Enter your mainland mobile phone number, obtain and enter the verification code. Set a password. Complete authentication. Registration completed.
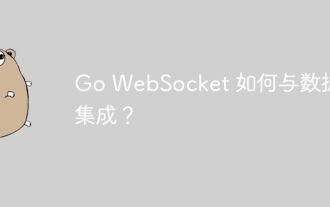
How to integrate GoWebSocket with a database: Set up a database connection: Use the database/sql package to connect to the database. Store WebSocket messages to the database: Use the INSERT statement to insert the message into the database. Retrieve WebSocket messages from the database: Use the SELECT statement to retrieve messages from the database.
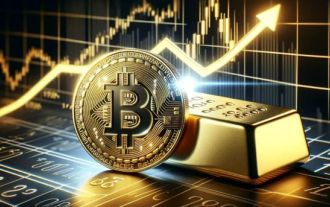
Gate.io Sesame Open is the world's leading blockchain digital asset trading platform, including fiat currency trading, currency trading, leveraged trading, perpetual contracts, ETF leveraged tokens, wealth management, Startup initial public offering and other sections, providing users with security, stability, openness and transparency.
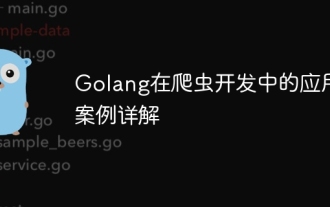
The Go language is known for its concurrency and high performance, making it an ideal choice for web crawler development. Create a website crawler: Go language provides simple and easy-to-learn syntax, suitable for quickly writing crawlers. Distributed crawlers: Go's goroutines and message queues support the creation of scalable and reliable distributed crawlers. Deployment and monitoring: Go’s portability and monitoring tools enable easy deployment and monitoring of crawler performance and reliability.
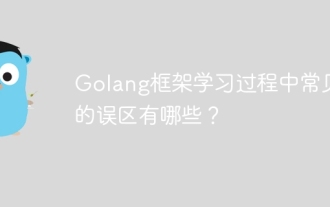
There are five misunderstandings in Go framework learning: over-reliance on the framework and limited flexibility. If you don’t follow the framework conventions, the code will be difficult to maintain. Using outdated libraries can cause security and compatibility issues. Excessive use of packages obfuscates code structure. Ignoring error handling leads to unexpected behavior and crashes.
