Explore the special data types in Go language
Special data types in the Go language include pointers (used for indirect access to values), arrays (fixed-length collections of elements), slices (variable-length arrays), structures (custom data types), and interfaces (defined methods sign). These data types provide simplicity, efficiency, and type safety, which are useful when dealing with specific needs.
Explore the special data types in the Go language
The Go language provides some special data types to handle specific needs. They provide simplicity, efficiency, and type safety.
1. Pointer (*Type)
A pointer is a data type that refers to a memory address, which allows indirect access to the underlying value. Using pointers, you can modify the underlying value without returning a new value.
func main() { // 定义一个指向 int 变量的指针 ptr := new(int) // 通过指针修改 int 值 *ptr = 10 fmt.Println(*ptr) // 输出: 10 }
2. Array ([n]Type)
An array is a fixed-size collection of elements, all of which have the same type. Arrays are value types, not reference types.
func main() { // 定义一个长度为 5 的 int 数组 arr := [5]int{1, 2, 3, 4, 5} // 访问数组元素 fmt.Println(arr[2]) // 输出: 3 }
3. Slice ([]Type)
A slice is a variable-length version of an array. Slices can change size dynamically without specifying a length limit. Unlike arrays, slices are reference types.
func main() { // 定义一个 int 切片,初始化容量为 5 s := make([]int, 0, 5) // 添加元素到切片 s = append(s, 1, 2, 3) fmt.Println(s) // 输出: [1 2 3] }
4. Structure (struct)
Structure is a custom data type that allows different types of data to be organized into a unit. Structure members can be accessed by name.
type Person struct { Name string Age int } func main() { // 定义一个 Person 结构体 person := Person{Name: "John Doe", Age: 30} fmt.Println(person.Name) // 输出: John Doe }
5. Interface
An interface defines a set of method signatures without implementation. Any type can implement an interface as long as it implements all methods defined in the interface.
type Shape interface { Area() float64 } type Circle struct { Radius float64 } func (c Circle) Area() float64 { return math.Pi * c.Radius * c.Radius } func main() { // 定义一个 Circle 类型的值 circle := Circle{Radius: 5} // 将 Circle 值转换为实现了 Shape 接口 var shape Shape = circle fmt.Println(shape.Area()) // 输出: 78.53981633974483 }
Practical case:
Use pointers to optimize function performance
Pass large structures or slices as function parameters by using pointers Efficiency can be improved because the function can modify the underlying value without having to return a new copy.
Processing data using arrays and slices
Arrays and slices are widely used to store and process data. You can use loops and built-in functions to efficiently traverse, sort, and filter data.
Use structures to organize related data
Structures allow the creation of complex custom types to organize related fields into an entity. This simplifies the presentation and manipulation of data.
Use interfaces to achieve code reusability
Interfaces enable different types to have the same behavior. This promotes code reusability and extensibility.
The above is the detailed content of Explore the special data types in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










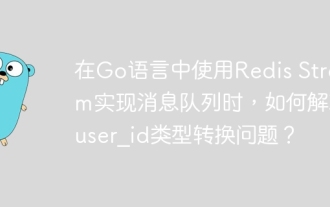
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
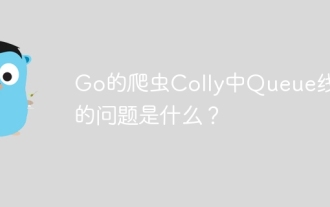
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
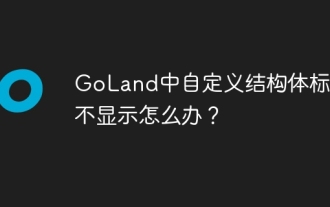
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
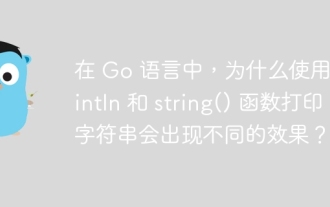
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
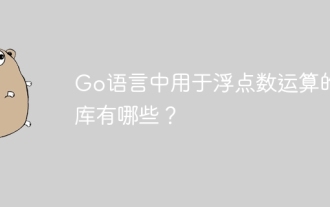
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
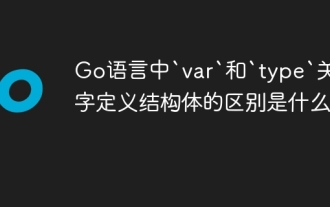
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
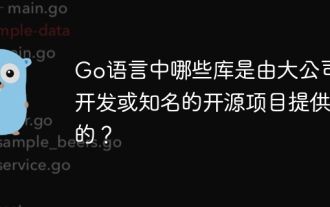
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
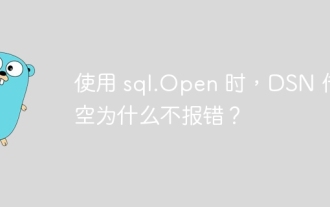
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
