


Using Golang reflection to implement structure field traversal and modification
Go reflection can be used to traverse and modify structure fields. Field traversal: Use reflect.TypeOf and reflect.Field to traverse structure fields. Field modification: Access and modify the values of structure fields through Elem and Set. Practical case: Use reflection to convert the structure into a map, and then convert the map into JSON.
Use Go reflection to implement structure field traversal and modification
Go reflection is a powerful technology that allows us to check and Modify types and values in the program. It is ideal for working with unknown or dynamic data, as well as reflecting on existing structures for introspection operations.
Field traversal
To traverse the fields of a structure, you can use the reflect.TypeOf
function to retrieve the type information of the structure, and then use NumField
method gets the number of fields. Each field can be accessed through the Field
method, which returns a reflect.StructField
object.
type Person struct { Name string Age int } func main() { person := Person{"Alice", 25} t := reflect.TypeOf(person) for i := 0; i < t.NumField(); i++ { field := t.Field(i) fmt.Println(field.Name, field.Type) } }
Field modification
You can access the value of the structure field through the Elem
method, which will return reflect.Value
Object. To modify a field, you can use the Set
method, which accepts a reflect.Value
parameter:
// 使用 Elem 访问字段值 nameField := reflect.ValueOf(person).Elem().FieldByName("Name") // 使用 Set 修改字段值 nameField.SetString("Bob") fmt.Println(person.Name) // 输出:Bob
Practical case
Let's say we have a struct that stores a user's personal information, but we want to return it in JSON format. We can use reflection to convert the structure into map[string]interface{}
:
type User struct { Name string Age int Password string } func main() { user := User{"Alice", 25, "secret"} // 将结构体转换为 map userMap := make(map[string]interface{}) t := reflect.TypeOf(user) for i := 0; i < t.NumField(); i++ { field := t.Field(i) value := reflect.ValueOf(user).Elem().FieldByName(field.Name) userMap[field.Name] = value.Interface() } // 将 map 转换为 JSON json, err := json.Marshal(userMap) if err != nil { // 处理错误 } fmt.Println(string(json)) // 输出:{"Name":"Alice","Age":25} }
Conclusion
Go reflection provides a powerful way to traverse and modify structure fields. By using reflect.TypeOf
, reflect.StructField
, reflect.Value
and reflect.Set
we can handle unknown or dynamic data, And perform reflection operations on existing structures to achieve code scalability and flexibility.
The above is the detailed content of Using Golang reflection to implement structure field traversal and modification. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
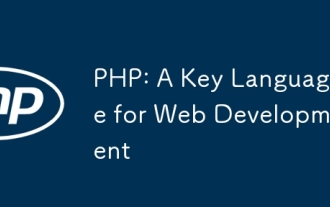
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
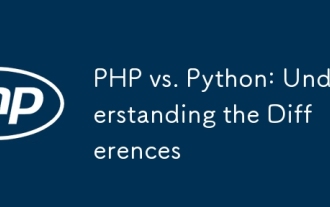
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
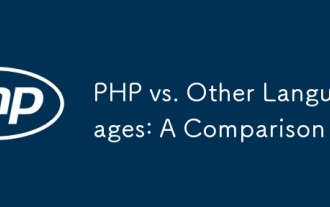
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
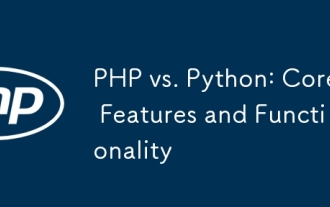
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
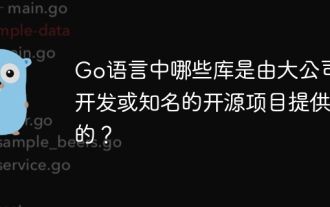
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
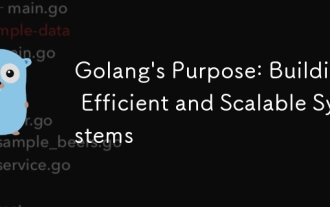
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
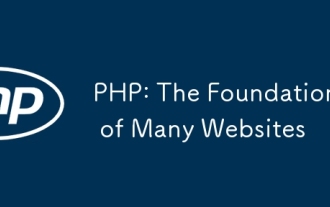
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
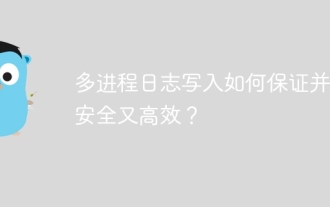
Efficiently handle concurrency security issues in multi-process log writing. Multiple processes write the same log file at the same time. How to ensure concurrency is safe and efficient? This is a...
