Research on the memory management mechanism of Go language
Exploration on the memory management mechanism of Go language
As a modern programming language, Go language has attracted much attention for its efficient concurrency performance and convenient programming model. Among them, memory management is one of the important factors for its performance advantages. This article will delve into the memory management mechanism of the Go language and demonstrate its working principle through specific code examples.
Memory allocation and recycling
The memory management of the Go language uses a mechanism called "automatic garbage collection (GC)", that is, programmers do not need to manually manage the allocation and recycling of memory. This is automatically taken care of by the runtime system. In Go language, use make
and new
to allocate memory.
Use make
for memory allocation
make
The function is used to create objects of slice, map and channel types, and returns an initialized Example. The usage of the make
function is as follows:
slice := make([]int, 0, 10) m := make(map[string]int) ch := make(chan int)
In the above example, an empty slice, an empty map and an int type are created through the make
function. channel.
Use new
for memory allocation
new
The function is used to allocate memory space for a type and return a pointer of the type. new
The usage example of the function is as follows:
var i *int i = new(int)
In the above example, the memory space is allocated for the int type through the new
function and assigned to the pointeri
.
Garbage Collection (GC) Algorithm
The garbage collection algorithm of Go language adopts an algorithm based on mark and sweep. When an object is no longer referenced, the GC reclaims memory by marking all reachable objects and clearing unmarked objects.
The following is a simple example that demonstrates the garbage collection process:
package main import "fmt" type Node struct { data int next *Node } func main() { var a, b, c Node a.data = 1 b.data = 2 c.data = 3 a.next = &b b.next = &c c.next = nil // 断开a对b的引用 a.next = nil // 垃圾回收 fmt.Println("垃圾回收前:", a, b, c) // 手动触发垃圾回收 // runtime.GC() fmt.Println("垃圾回收后:", a, b, c) }
In the above example, when a.next
is assigned the value nil
, the reference of a to b is disconnected. At this time, the b object becomes an unreachable object and will be recycled by the garbage collector.
Handling of memory leaks
Although the Go language has an automatic garbage collection mechanism, memory leaks may still occur. A memory leak refers to a situation where the memory allocated in a program is not released correctly, resulting in excessive memory usage.
The following is a sample code that may cause a memory leak:
package main import ( "fmt" "time" ) func leakyFunc() { data := make([]int, 10000) // do something with data } func main() { for { leakyFunc() time.Sleep(time.Second) } }
In the above example, an int array with a length of 10000 is created in the leakyFunc
function, but The memory space occupied by the array is not released. If the leakyFunc
function is called frequently in a loop, memory leaks will occur.
In order to avoid memory leaks, developers should pay attention to promptly releasing memory resources that are no longer needed. They can reduce memory usage through defer
statements, reasonable use of cache pools, etc.
Summary
This article explores the memory management mechanism of Go language from the aspects of memory allocation, garbage collection algorithm and memory leak processing. By having an in-depth understanding of the memory management of the Go language, developers can better understand the memory usage of the program during runtime, avoid problems such as memory leaks, and improve the performance and stability of the program. Hope this article can be helpful to readers.
The above is the detailed content of Research on the memory management mechanism of Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










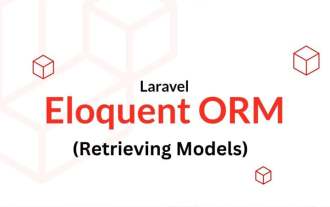
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
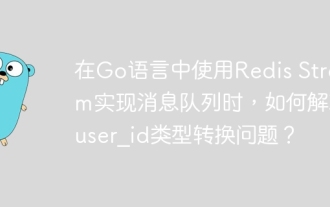
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
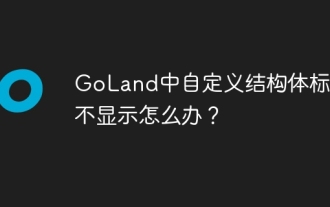
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
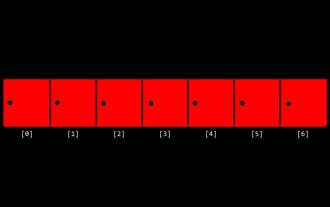
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
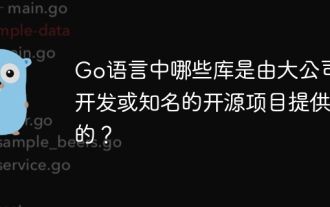
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
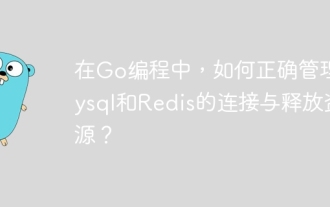
Resource management in Go programming: Mysql and Redis connect and release in learning how to correctly manage resources, especially with databases and caches...
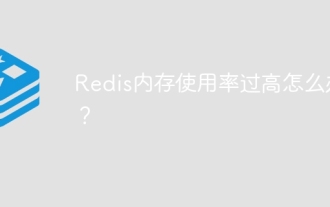
Redis memory soaring includes: too large data volume, improper data structure selection, configuration problems (such as maxmemory settings too small), and memory leaks. Solutions include: deletion of expired data, use compression technology, selecting appropriate structures, adjusting configuration parameters, checking for memory leaks in the code, and regularly monitoring memory usage.
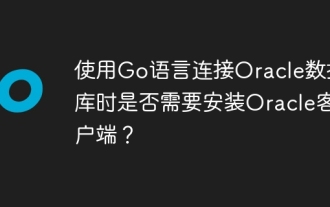
Do I need to install an Oracle client when connecting to an Oracle database using Go? When developing in Go, connecting to Oracle databases is a common requirement...
