Analyze the risk factors and solutions of Go language
Title: Analysis of risk factors and solutions of Go language
In recent years, Go language, as a rapidly developing programming language, has received more and more development attention and use. However, just like other programming languages, Go language also has some risk factors that may affect the smooth progress of the development process. This article will deeply analyze the risk factors of Go language and provide corresponding solutions. It will also combine specific code examples to deepen understanding.
1. Memory Leak
Memory leak is a common risk factor in Go language development. In Go, when a program no longer uses a variable or data structure, if the related memory space is not released correctly, it will cause a memory leak problem. This causes the program's memory footprint to continually increase, which may eventually lead to program crashes or performance degradation.
Solution: In the Go language, ensuring the timely release of resources by using the defer keyword is a common method to solve the memory leak problem. The following is a simple example:
func main() { file, err := os.Open("file.txt") if err != nil { log.Fatal(err) } defer file.Close() // 对file进行各种操作 }
In the above code example, using the defer keyword ensures that the file resource is closed after the main function is executed, avoiding memory leaks.
2. Concurrency control
The Go language is known for its natural concurrency support, but concurrent operations may bring some potential risks. When multiple goroutines access shared resources at the same time, without a good concurrency control mechanism, problems such as data competition and deadlock are prone to occur, affecting the correctness and performance of the program.
Solution: In the Go language, you can use the atomic operations, mutex locks, channels and other mechanisms provided by Go for concurrency control. The following is an example of using a mutex lock to solve concurrency problems:
var m sync.Mutex var balance int func deposit(amount int) { m.Lock() defer m.Unlock() balance += amount } func main() { wg := sync.WaitGroup{} for i := 0; i < 1000; i++ { wg.Add(1) go func() { deposit(100) wg.Done() }() } wg.Wait() fmt.Println(balance) }
In the above code example, the safe access of the balance variable between goroutines is ensured by using the sync.Mutex mutex lock, avoiding data Competition issues.
3. Security Issues
Since the Go language is a relatively new language, there may be some potential issues in terms of security, such as code injection, cross-border access, etc. These security issues may lead to programs being exploited for attacks, causing serious consequences.
Solution: In order to improve the security of Go programs, developers can take some measures, such as input validation, using the security functions provided by the standard library, and avoiding the use of unsafe operations. The following is a simple input validation example:
func processInput(input string) { if strings.Contains(input, "unsafe keyword") { log.Fatal("Input contains unsafe keyword") } // 处理input }
The above code example ensures the security of the program by verifying whether the input string contains unsafe keywords.
In summary, as a powerful programming language, Go language has some risk factors, but the impact of these risks can be effectively reduced through appropriate solutions. When developing using the Go language, developers should pay attention to these risk factors and choose appropriate solutions based on the actual situation to ensure the stability and security of the program.
The above is the detailed content of Analyze the risk factors and solutions of Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
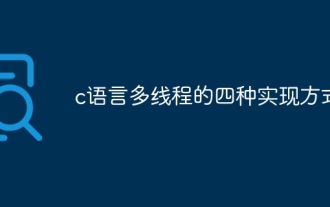
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
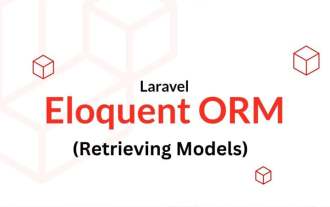
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
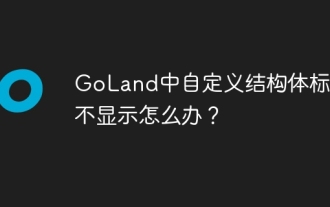
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
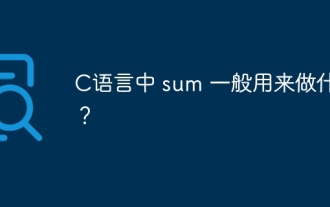
There is no function named "sum" in the C language standard library. "sum" is usually defined by programmers or provided in specific libraries, and its functionality depends on the specific implementation. Common scenarios are summing for arrays, and can also be used in other data structures, such as linked lists. In addition, "sum" is also used in fields such as image processing and statistical analysis. An excellent "sum" function should have good readability, robustness and efficiency.
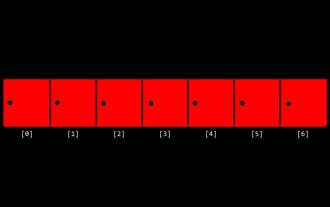
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
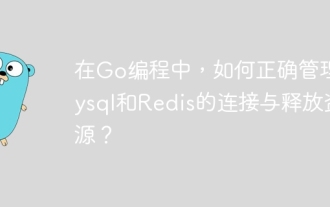
Resource management in Go programming: Mysql and Redis connect and release in learning how to correctly manage resources, especially with databases and caches...
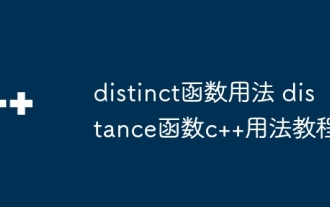
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
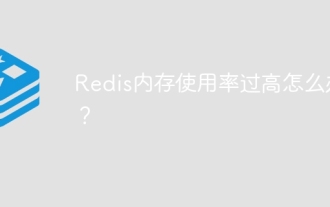
Redis memory soaring includes: too large data volume, improper data structure selection, configuration problems (such as maxmemory settings too small), and memory leaks. Solutions include: deletion of expired data, use compression technology, selecting appropriate structures, adjusting configuration parameters, checking for memory leaks in the code, and regularly monitoring memory usage.
