Golang's potential and challenges in operating system development
The potential and challenges of Golang in operating system development
In recent years, due to the efficiency and simplicity of Golang (Go language), More and more developers are beginning to apply it to various fields, including operating system development. As a compiled language with strong concurrency and excellent performance, Golang brings new possibilities to operating system development, but it also faces some challenges. This article will explore the potential and challenges of Golang in operating system development and provide some specific code examples.
1. The potential of Golang
1. Concurrency performance advantages
As a language with powerful concurrency, Golang has the concept of lightweight threads (goroutine), which can Easily implement efficient concurrent programming. In operating system development, a large number of concurrent tasks need to be processed, and Golang can provide good support. The following is a simple concurrency example:
package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup wg.Add(2) go func() { defer wg.Done() //Here is the code for the first concurrent task fmt.Println("Hello from goroutine 1") }() go func() { defer wg.Done() //Here is the code for the second concurrent task fmt.Println("Hello from goroutine 2") }() wg.Wait() }
2. Performance Optimization
Golang performs well in terms of performance. Optimized Golang programs can achieve performance levels comparable to C/C programs. In operating system development, efficient code execution speed is required, and Golang can help developers achieve this goal. The following is a simple performance optimization example:
package main import "fmt" func main() { const n = 1000000 var sum int for i := 0; i < n; i { sum = i } fmt.Println(sum) }
2. Challenges of Golang
1. There is less underlying support
Compared with traditional operating system development languages (such as C/C), Golang has The underlying operating system development support is relatively lacking. The implementation of some underlying operating system features may require the use of C language interfaces, which adds a certain degree of complexity. The following is an example using the C language interface:
package main /* #include <stdio.h> void cFunction() { printf("Hello from C function "); } */ import "C" func main() { C.cFunction() }
2. Memory management challenges
In operating system development, memory management is crucial and needs to ensure efficiency and security. Golang's garbage collection mechanism may affect the real-time performance and stability of the operating system, so memory management issues need to be handled carefully. The following is an example of a memory management challenge:
package main import "fmt" func main() { slice := make([]int, 1000000) // A lot of memory allocation // May trigger garbage collection // ... // Continue executing other code here }
Conclusion
Although Golang faces some challenges in operating system development, its powerful concurrency performance and performance optimization capabilities give it the potential to play an important role in operating system development. By demonstrating the application of Golang in operating system development in code examples, we can better understand Golang's unique advantages and challenges in this field. It is hoped that Golang can show greater potential in the field of operating system development in the future and bring more possibilities to technological innovation.
(Number of words: about 850 words)
The above is the detailed content of Golang's potential and challenges in operating system development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
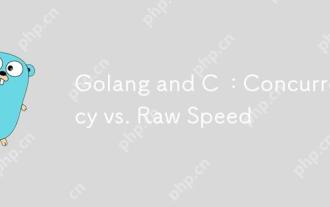
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
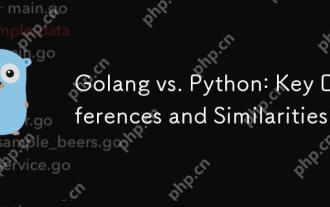
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
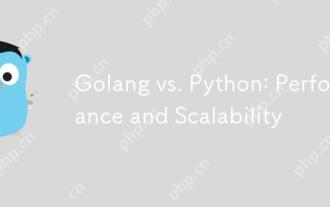
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
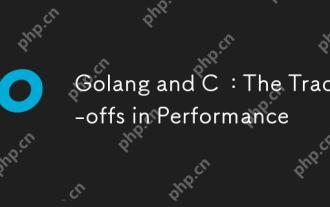
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
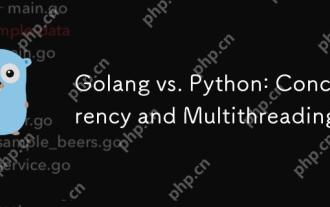
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
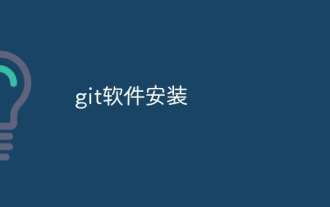
Installing Git software includes the following steps: Download the installation package and run the installation package to verify the installation configuration Git installation Git Bash (Windows only)
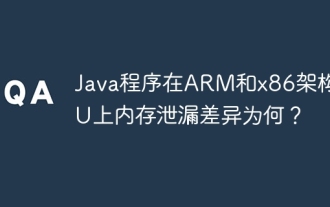
Analysis of memory leak phenomenon of Java programs on different architecture CPUs. This article will discuss a case where a Java program exhibits different memory behaviors on ARM and x86 architecture CPUs...
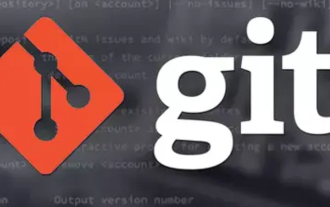
There are many ways to customize a development environment, but the global Git configuration file is one that is most likely to be used for custom settings such as usernames, emails, preferred text editors, and remote branches. Here are the key things you need to know about global Git configuration files.
