Decryption: Waiting strategy of main function in Go language
Decryption: The waiting strategy of the main function in Go language requires specific code examples
Go language is a concurrent programming language, and its waiting strategy of the main function is particularly important . The main function needs to ensure that it exits after all goroutines have been executed, otherwise it may cause the program to terminate prematurely. This article will introduce several common main function waiting strategies and provide specific code examples.
In the Go language, WaitGroup or channel in the sync package is usually used to implement the waiting of the main function. Below we will introduce the specific applications of these two methods respectively.
- Use WaitGroup in the sync package
WaitGroup is a synchronization mechanism that can be used to wait for the end of a group of goroutines. The Add method is mainly used to increase the number of waiting goroutines, the Done method is used to reduce the number, and the Wait method waits for all goroutines to be executed. The following is a sample code:
package main import ( "fmt" "sync" ) func worker(id int, wg *sync.WaitGroup) { defer wg.Done() fmt.Printf("Worker %d is working ", id) } func main() { var wg sync.WaitGroup for i := 1; i <= 3; i++ { wg.Add(1) go worker(i, &wg) } wg.Wait() fmt.Println("All workers have finished") }
In the above code, we define a worker function to simulate a goroutine that needs to be executed, and then start 3 worker goroutines in the main function and wait through the Wait method They are executed.
- Using channel
Another common main function waiting strategy is to use channel. We can create a channel and let each goroutine send a signal to this channel when it ends. The main function can receive this signal to determine whether all goroutines have been executed. The following is a sample code:
package main import "fmt" func worker(id int, ch chan bool) { fmt.Printf("Worker %d is working ", id) ch <- true } func main() { numWorkers := 3 ch := make(chan bool, numWorkers) for i := 1; i <= numWorkers; i++ { go worker(i, ch) } for i := 1; i <= numWorkers; i++ { <-ch } fmt.Println("All workers have finished") }
In this example, we create a channel with a capacity of numWorkers and let each worker goroutine send a value to this channel at the end. The main function receives these values to determine whether all goroutines have been executed.
Summary
Through the above two specific code examples, we have learned about two common ways to implement the waiting strategy of the main function in the Go language: using the WaitGroup in the sync package and using the channel . In actual development, it is very important to choose an appropriate waiting strategy according to the specific situation, so as to ensure that the program can correctly wait for all goroutines to finish executing before exiting during concurrent execution.
The above is the detailed content of Decryption: Waiting strategy of main function in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










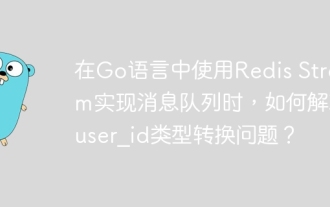
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
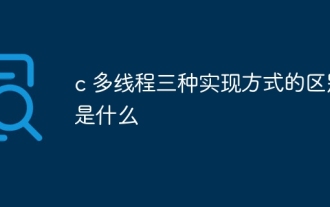
Multithreading is an important technology in computer programming and is used to improve program execution efficiency. In the C language, there are many ways to implement multithreading, including thread libraries, POSIX threads, and Windows API.
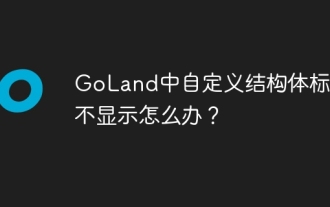
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
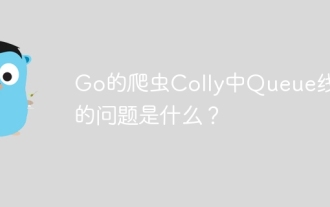
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
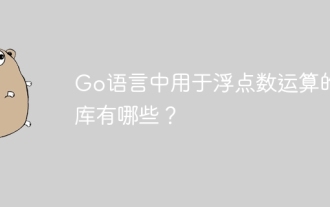
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
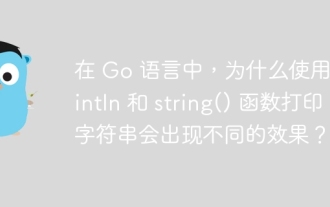
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
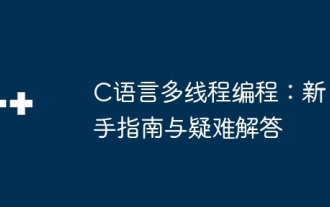
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
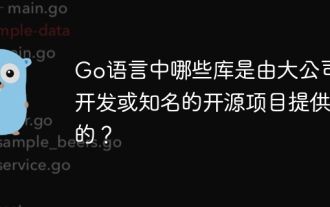
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
