


Detailed explanation of function parameter passing method in Go language
The Go language is an open source programming language developed by Google and is designed to improve developer productivity and code reliability. In the Go language, the way function parameters are passed is very important, as it can affect the performance and memory usage of the program.
1. Value passing
Value passing refers to copying the value of the parameter and passing it to the function. Operations on parameters inside the function will not affect the values of external variables. Here is a simple value passing example:
package main import "fmt" func modifyValue(x int) { x = x * 2 fmt.Println("Inside function:", x) } func main() { num := 10 modifyValue(num) fmt.Println("Outside function:", num) }
In the above example, the modifyValue
function receives an integer parameter and multiplies the parameter by 2. After the function call, the value of the external variable num
has not changed.
2. Passing by reference
Passing by reference means passing the address of the parameter to the function, and the function can modify the value of the external variable through the address. In the Go language, reference types such as slices, maps, and channels are passed by reference by default. Here is an example of passing by reference:
package main import "fmt" func modifySlice(s []int) { s[0] = 100 fmt.Println("Inside function:", s) } func main() { slice := []int{1, 2, 3} modifySlice(slice) fmt.Println("Outside function:", slice) }
In the above example, the modifySlice
function receives a slice parameter and modifies the first element to 100. After the function call, the value of the outer slice slice
has also changed.
3. Pass pointer
By passing the pointer of the parameter, the function can directly operate the value in the memory address pointed to by the parameter. This method can reduce the overhead of copying parameters, and is especially suitable for large data structures and situations that require frequent modifications. Here is an example of passing a pointer:
package main import "fmt" func modifyPointer(x *int) { *x = *x * 2 fmt.Println("Inside function:", *x) } func main() { num := 10 modifyPointer(&num) fmt.Println("Outside function:", num) }
In the above example, the modifyPointer
function receives an integer pointer parameter and multiplies the value pointed to by the pointer by 2. After the function call, the value of the external variable num
has also changed.
Through the above examples, we can see the application and impact of different parameter passing methods in the Go language. In actual development, choosing the appropriate delivery method can improve the performance and maintainability of the program. I hope this article is helpful to readers, thank you for reading!
The above is the detailed content of Detailed explanation of function parameter passing method in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










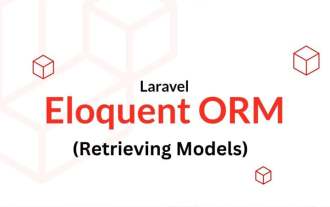
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
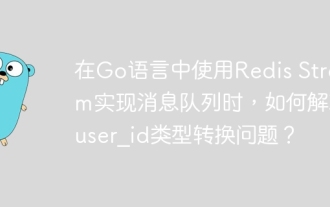
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
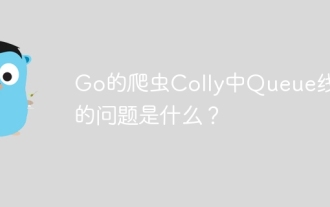
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
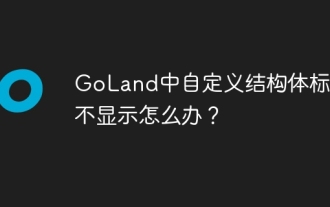
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
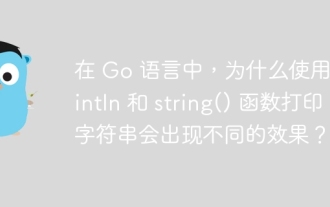
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
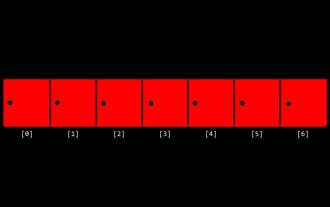
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
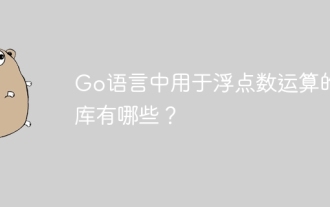
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
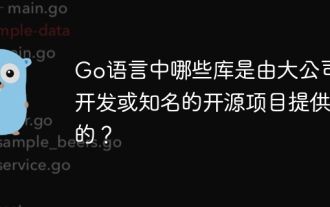
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
