


Python logging module: Solve your toughest knowledge points
#python's logging module is a powerful tool that helps you log events and messages in your application. It provides a unified interface to configure and manage log records, allowing you to easily handle logging tasks. This article will take an in-depth look at the logging module, address common knowledge points, and provide practical examples.
Configuring logging
In order to enable logging, you must first configure a logger. This can be done via the logging.basicConfig()
function. Here's how to configure a basic logger:
import logging # 配置日志记录 logging.basicConfig( level=logging.INFO, fORMat="%(asctime)s - %(levelname)s - %(message)s", filename="my_log.log", )
In this example, we set the logging level to INFO, and specified the format of the log message and the file name of the log file.
Logging level
The logging module defines five logging levels:
- DEBUG
- INFO
- WARNING
- ERROR
- CRITICAL
The logging level determines which types of messages are logged. For example, if you set the level to INFO, only INFO level messages and higher level messages (such as WARNING and ERROR) are logged.
Log message
Use logging.info()
, logging.warning()
and other functions to log messages. The message can contain any string or object, for example:
logging.info("这是信息消息.") logging.warning("这是警告消息.")
filter
Filters allow you to control which messages are logged. You can create a custom filter class or use a built-in filter such as logging.Filter
. The following example uses logging.Filter
to filter out messages containing a specific string:
class MyFilter(logging.Filter): def filter(self, record): return "my_string" not in record.msg logging.basicConfig( ... filters=[MyFilter()] )
Log handler
The log handler is responsible for processing log messages. The logging module provides various built-in handlers, such as logging.StreamHandler
and logging.FileHandler
. Here's how to use logging.StreamHandler
to output log messages to the console:
handler = logging.StreamHandler() handler.setLevel(logging.INFO) logging.getLogger().addHandler(handler)
Customized logging
The logging module allows you to create custom logging configurations and handlers. You can customize logging formats, create custom logging levels, and use custom filtering and processing logic.
troubleshooting
Logging is critical for troubleshooting and debugging applications. By viewing the log files, you can understand the behavior of the application and identify the source of the problem. Here are some common troubleshooting tips:
- Check the log file for error messages.
- Use
logging.getLogger().getEffectiveLevel()
to check the logging level. - Verify that the logging handler is configured correctly.
- Use filters to narrow the scope of log messages.
in conclusion
Python’s logging module is a powerful tool that can enhance your application’s logging and debugging capabilities. With the knowledge provided in this guide, you will be able to effectively configure and use the logging module to solve the toughest programming challenges.
The above is the detailed content of Python logging module: Solve your toughest knowledge points. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










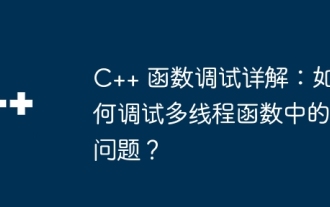
C++ multi-thread debugging can use GDB: 1. Enable debugging information compilation; 2. Set breakpoints; 3. Use infothreads to view threads; 4. Use thread to switch threads; 5. Use next, stepi, and locals to debug. Actual case debugging deadlock: 1. Use threadapplyallbt to print the stack; 2. Check the thread status; 3. Single-step the main thread; 4. Use condition variables to coordinate access to solve the deadlock.
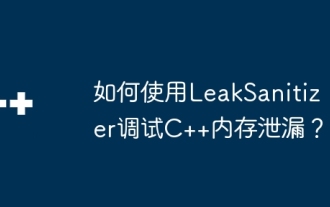
How to use LeakSanitizer to debug C++ memory leaks? Install LeakSanitizer. Enable LeakSanitizer via compile flag. Run the application and analyze the LeakSanitizer report. Identify memory allocation types and allocation locations. Fix memory leaks and ensure all dynamically allocated memory is released.
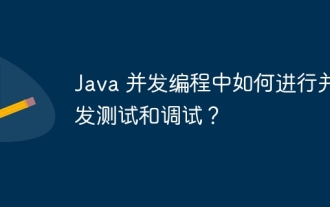
Concurrency testing and debugging Concurrency testing and debugging in Java concurrent programming are crucial and the following techniques are available: Concurrency testing: Unit testing: Isolate and test a single concurrent task. Integration testing: testing the interaction between multiple concurrent tasks. Load testing: Evaluate an application's performance and scalability under heavy load. Concurrency Debugging: Breakpoints: Pause thread execution and inspect variables or execute code. Logging: Record thread events and status. Stack trace: Identify the source of the exception. Visualization tools: Monitor thread activity and resource usage.
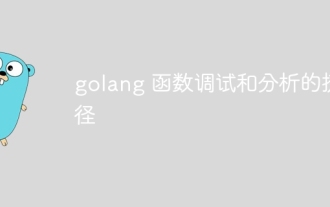
This article introduces shortcuts for Go function debugging and analysis, including: built-in debugger dlv, which is used to pause execution, check variables, and set breakpoints. Logging, use the log package to record messages and view them during debugging. The performance analysis tool pprof generates call graphs and analyzes performance, and uses gotoolpprof to analyze data. Practical case: Analyze memory leaks through pprof and generate a call graph to display the functions that cause leaks.
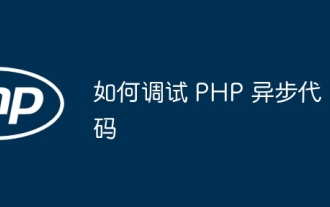
Tools for debugging PHP asynchronous code include: Psalm: a static analysis tool that can find potential errors. ParallelLint: A tool that inspects asynchronous code and provides recommendations. Xdebug: An extension for debugging PHP applications by enabling a session and stepping through the code. Other tips include using logging, assertions, running code locally, and writing unit tests.
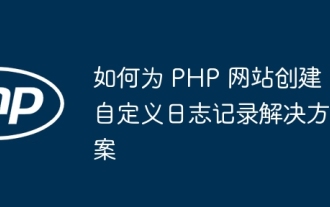
There are several ways to create a custom logging solution for your PHP website, including: using a PSR-3 compatible library (such as Monolog, Log4php, PSR-3Logger) or using PHP native logging functions (such as error_log(), syslog( ), debug_print_backtrace()). Monitoring the behavior of your application and troubleshooting issues can be easily done using a custom logging solution, for example: Use Monolog to create a logger that logs messages to a disk file.
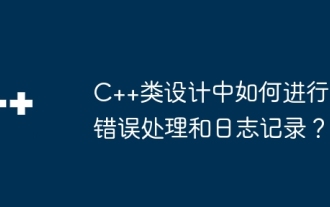
Error handling and logging in C++ class design include: Exception handling: catching and handling exceptions, using custom exception classes to provide specific error information. Error code: Use an integer or enumeration to represent the error condition and return it in the return value. Assertion: Verify pre- and post-conditions, and throw an exception if they are not met. C++ library logging: basic logging using std::cerr and std::clog. External logging libraries: Integrate third-party libraries for advanced features such as level filtering and log file rotation. Custom log class: Create your own log class, abstract the underlying mechanism, and provide a common interface to record different levels of information.
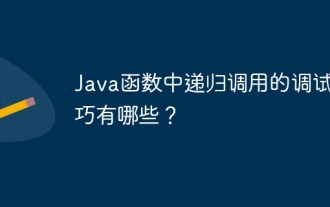
The following techniques are available for debugging recursive functions: Check the stack traceSet debug pointsCheck if the base case is implemented correctlyCount the number of recursive callsVisualize the recursive stack
