Python CPython performance optimization tips
#python is widely used in various fields and is highly regarded for its ease of use and powerful functions. However, its performance can become a bottleneck in some cases. Through an in-depth understanding of CPython Virtual Machine and some clever Optimization techniques, the running efficiency of Python programs can be significantly improved.
1. Understand the CPython virtual machine
CPython is the most popular implementation of Python, which uses a virtual machine (VM) to execute Python code. The VM interprets bytecodes into machine instructions, which incurs a certain amount of time overhead. Understanding how VMs work helps us identify and optimize performance bottlenecks.
2. Garbage collection
Python uses a reference counting mechanism for garbage collection, but it may cause periodic garbage collection pauses, thus affecting the responsiveness of the program. To lessen the impact, you can use the following techniques:
-
Use
del
Release objects that are no longer used: Release objects that are no longer needed early to reduce the burden of garbage collection. - Use weak references: Use weak references to cache objects, and the system will automatically release them when they are no longer used.
- Disable circular references: Avoid forming circular references between objects, which will cause them to never be released.
3. Global Interpreter Lock (GIL)
The GIL is a mechanism that allows only one thread to execute Python code at a time. This may limit the parallelism of multithreaded programs. Although CPython 3.11 introduces partial GIL release, the following optimization tips still need to be considered:
- Use a thread pool: Batch tasks and execute them asynchronously via a thread pool .
- Use C extensions: Write C extensions for critical code, bypassing the GIL.
- Consider using other interpreters: such as PyPy or Jython, which use different GIL implementations or do not use the GIL at all.
4. Optimize data structures and algorithms
Appropriate data structures and algorithms are crucial to program performance. Choose the best data structure according to specific needs, for example:
- List: For sequential access and modification.
- Tuple: For immutable data.
- Dictionary: For quick search and insertion.
- Collection: Used for quick membership testing .
5. Code analysis and optimization
Use a performance analysis tool such as cProfile or LineProfiler to identify performance bottlenecks in your program. Perform targeted optimizations by refactoring code, simplifying algorithms, or using more optimized libraries.
6. Use optimized libraries
There are many optimized libraries in the Python ecosystem that can be used to improve performance. For example:
- NumPy: is used for numerical calculations.
- SciPy: is used for scientific computing.
- Pandas: Used for data analysis and manipulation.
7. Avoid unnecessary duplication
Avoid unnecessary copying of objects in Python. Use the copy
and deepcopy
functions to copy only when needed.
Demo code:
# 使用 `del` 释放不再需要的对象 my_dict = {"key": "value"} del my_dict # 使用弱引用对缓存对象进行引用 from weakref import WeakKeyDictionary cache = WeakKeyDictionary() cache[my_obj] = "data" # 使用线程池异步执行任务 from concurrent.futures import ThreadPoolExecutor with ThreadPoolExecutor() as executor: results = executor.map(my_function, my_inputs)
in conclusion
By understanding the CPython virtual machine, adopting garbage collection optimization strategies, avoiding the impact of GIL, optimizing data structures and algorithms, utilizing optimized libraries, and avoiding unnecessary copies, we can effectively improve the performance of Python programs. These tips can help devers create smoother, more responsive applications that take full advantage of the power of Python.
The above is the detailed content of Python CPython performance optimization tips. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










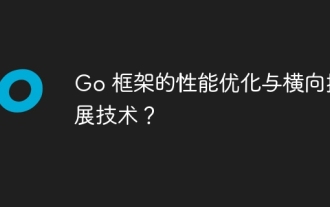
In order to improve the performance of Go applications, we can take the following optimization measures: Caching: Use caching to reduce the number of accesses to the underlying storage and improve performance. Concurrency: Use goroutines and channels to execute lengthy tasks in parallel. Memory Management: Manually manage memory (using the unsafe package) to further optimize performance. To scale out an application we can implement the following techniques: Horizontal Scaling (Horizontal Scaling): Deploying application instances on multiple servers or nodes. Load balancing: Use a load balancer to distribute requests to multiple application instances. Data sharding: Distribute large data sets across multiple databases or storage nodes to improve query performance and scalability.
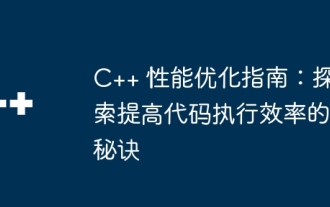
C++ performance optimization involves a variety of techniques, including: 1. Avoiding dynamic allocation; 2. Using compiler optimization flags; 3. Selecting optimized data structures; 4. Application caching; 5. Parallel programming. The optimization practical case shows how to apply these techniques when finding the longest ascending subsequence in an integer array, improving the algorithm efficiency from O(n^2) to O(nlogn).
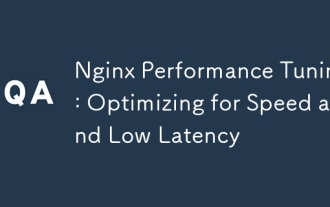
Nginx performance tuning can be achieved by adjusting the number of worker processes, connection pool size, enabling Gzip compression and HTTP/2 protocols, and using cache and load balancing. 1. Adjust the number of worker processes and connection pool size: worker_processesauto; events{worker_connections1024;}. 2. Enable Gzip compression and HTTP/2 protocol: http{gzipon;server{listen443sslhttp2;}}. 3. Use cache optimization: http{proxy_cache_path/path/to/cachelevels=1:2k
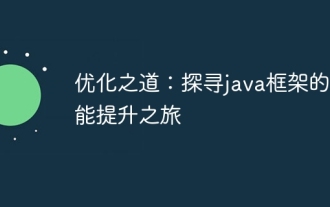
The performance of Java frameworks can be improved by implementing caching mechanisms, parallel processing, database optimization, and reducing memory consumption. Caching mechanism: Reduce the number of database or API requests and improve performance. Parallel processing: Utilize multi-core CPUs to execute tasks simultaneously to improve throughput. Database optimization: optimize queries, use indexes, configure connection pools, and improve database performance. Reduce memory consumption: Use lightweight frameworks, avoid leaks, and use analysis tools to reduce memory consumption.
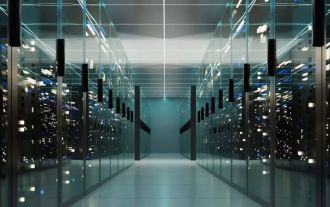
According to news from this site on May 24, Broadcom carried out drastic reforms after acquiring VMware. It sold non-core businesses such as the end-user computing department for US$4 billion, ended 59 products, and focused on providing support for large enterprises. The subscription method attracts enterprises to adopt. Technology media Techspot reported that large enterprises may not be able to afford VMware's price increases. Computershare, an Australian company with 24,000 virtual machines, may abandon VMware and focus on Nutanix products. Note from this site: Computershare mainly provides financial products and investor services to stock exchanges around the world. After Broadcom acquired VMware
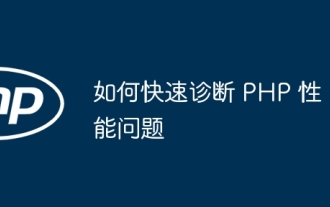
Effective techniques for quickly diagnosing PHP performance issues include using Xdebug to obtain performance data and then analyzing the Cachegrind output. Use Blackfire to view request traces and generate performance reports. Examine database queries to identify inefficient queries. Analyze memory usage, view memory allocations and peak usage.
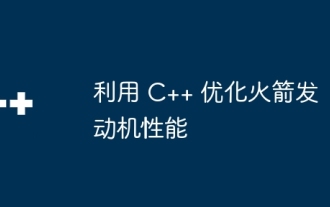
By building mathematical models, conducting simulations and optimizing parameters, C++ can significantly improve rocket engine performance: Build a mathematical model of a rocket engine and describe its behavior. Simulate engine performance and calculate key parameters such as thrust and specific impulse. Identify key parameters and search for optimal values using optimization algorithms such as genetic algorithms. Engine performance is recalculated based on optimized parameters to improve its overall efficiency.
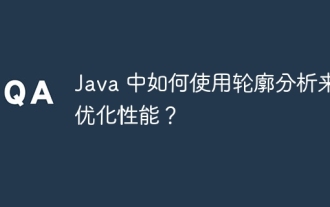
Profiling in Java is used to determine the time and resource consumption in application execution. Implement profiling using JavaVisualVM: Connect to the JVM to enable profiling, set the sampling interval, run the application, stop profiling, and the analysis results display a tree view of the execution time. Methods to optimize performance include: identifying hotspot reduction methods and calling optimization algorithms
