An in-depth discussion of the Map modification mechanism in Golang
The modification mechanism of Map in Golang refers to a series of rules and mechanisms involved in modifying the key-value pairs in the Map when using the Map type data structure. This article will introduce in detail the basic concepts, operation methods and modification mechanisms of Map in Golang, and use specific code examples to help readers have a deeper understanding of the Map modification mechanism.
1. The basic concept of Map in Golang
Map is an unordered collection of key-value pairs, where each key is unique. In Golang, Map is a reference type that can be created through the make function. The basic syntax of Map is as follows:
// 创建一个空Map mapName := make(map[keyType]valueType) // 创建并初始化一个Map mapName := map[keyType]valueType{ key1: value1, key2: value2, //... }
Among them, keyType is the type of key and valueType is the type of value. You can create an empty Map through the make function, or directly initialize the Map when declaring it.
2. Basic operation methods of Map
The basic operations of Map include inserting key-value pairs, obtaining key-value pairs, deleting key-value pairs, etc. The following are some commonly used Map operation methods:
- Insert key-value pairs:
mapName[key] = value
- Get key-value pairs:
value := mapName[key]
- Delete key-value pairs:
delete(mapName, key)
3. Map modification mechanism
In Golang, Map modification mechanism involves concurrent access issues. Map itself is an unsafe data structure for concurrent access, so when multiple goroutines read and write the same Map at the same time, it may lead to data race conditions and unexpected results. In order to avoid this situation, you can use the lock mechanism provided by the sync package to protect the read and write operations of the Map.
The following is a sample code that demonstrates how to use sync.Mutex to protect concurrent access to the Map:
package main import ( "fmt" "sync" ) func main() { var mu sync.Mutex m := make(map[string]int) // 启动多个goroutine同时对Map进行更新 for i := 0; i < 1000; i++ { go func() { mu.Lock() m["count"]++ mu.Unlock() }() } // 等待所有goroutine执行完成 for len(m) < 1000 { } fmt.Println(m) }
In the above example, use sync.Mutex to create a mutex mu , protect the read and write operations of Map m. When each goroutine updates the Map, it first calls mu.Lock() to lock it, and then calls mu.Unlock() to release the lock after the update is completed.
4. Summary
Through the above introduction and sample code, readers should have a deeper understanding of the Map modification mechanism in Golang. In actual development, especially in concurrent scenarios, you need to pay attention to the concurrent access issue of Map, and use the lock mechanism reasonably to protect Map operations and ensure the security and correctness of data. At the same time, to avoid frequent modification operations to the Map, you can consider using other concurrent and safe data structures such as channels to replace the Map to improve the performance and reliability of the program.
The above is the detailed content of An in-depth discussion of the Map modification mechanism in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










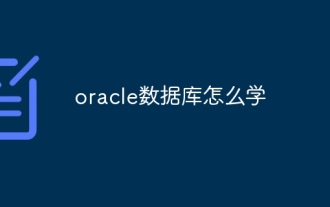
There are no shortcuts to learning Oracle databases. You need to understand database concepts, master SQL skills, and continuously improve through practice. First of all, we need to understand the storage and management mechanism of the database, master the basic concepts such as tables, rows, and columns, and constraints such as primary keys and foreign keys. Then, through practice, install the Oracle database, start practicing with simple SELECT statements, and gradually master various SQL statements and syntax. After that, you can learn advanced features such as PL/SQL, optimize SQL statements, and design an efficient database architecture to improve database efficiency and security.
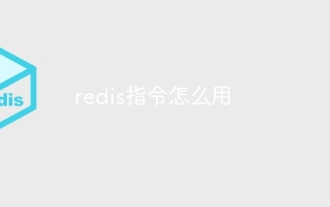
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
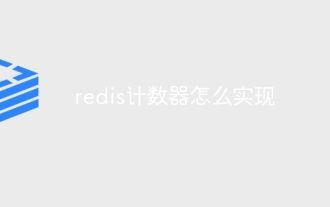
Redis counter is a mechanism that uses Redis key-value pair storage to implement counting operations, including the following steps: creating counter keys, increasing counts, decreasing counts, resetting counts, and obtaining counts. The advantages of Redis counters include fast speed, high concurrency, durability and simplicity and ease of use. It can be used in scenarios such as user access counting, real-time metric tracking, game scores and rankings, and order processing counting.
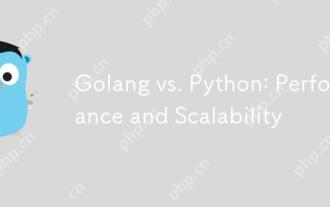
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
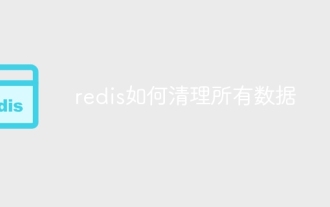
How to clean all Redis data: Redis 2.8 and later: The FLUSHALL command deletes all key-value pairs. Redis 2.6 and earlier: Use the DEL command to delete keys one by one or use the Redis client to delete methods. Alternative: Restart the Redis service (use with caution), or use the Redis client (such as flushall() or flushdb()).
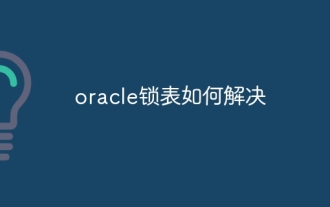
Oracle lock tables can be solved by viewing lock information and finding locked objects and sessions. Use the KILL command to terminate the idle locked session. Restart the database instance and release all locks. Use the ALTER SYSTEM KILL SESSION command to terminate a stubborn locked session. Use the DBMS_LOCK package for programmatic lock management. Optimize query to reduce lock frequency. Set lock compatibility level to reduce lock contention. Use concurrency control mechanisms to reduce locking requirements. Enable automatic deadlock detection, and the system will automatically roll back the deadlock session.
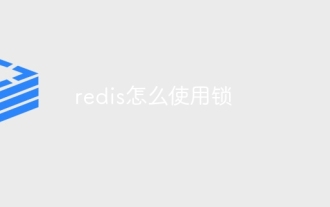
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
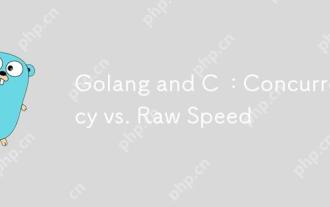
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
