


Golang code optimization: the role and practice of macro definitions
Title: Golang Code Optimization: The Role and Practice of Macro Definition
In Golang, macro definition is a convenient code optimization tool that can be used at compile time Replace code to reduce code duplication and improve code readability and maintainability. This article will introduce the role and practical methods of macro definitions, and use specific code examples to illustrate how to use macro definitions for code optimization in Golang.
What is a macro definition?
Macro definition is a preprocessing instruction that performs replacement operations during compilation. Similar functions can be achieved in Golang by using the go generate
instruction and the text/template
package. . Through macro definition, we can define some commonly used code snippets and then reference them where needed, thereby avoiding code duplication and improving code reusability.
The role of macro definition
- Code reuse: Macro definition can encapsulate some commonly used code snippets into macros and reference them in multiple codes. Reduce the repetitive writing of the same code and improve code reusability.
- Improve code readability: Through macro definition, we can encapsulate some complex code logic into simple macro commands, making the code more readable and understandable.
- Easy to maintain: Macro definitions can encapsulate some code fragments that need to be modified frequently into macros. When modifications are needed, you only need to modify the macro definitions to improve the maintainability of the code.
Practical method
In Golang, we can use the go generate
instruction to implement the function of macro definition. The following is a simple practical method:
- Create a
templates
folder in the project root directory to store macro-defined template files. - Create a file named
gen.go
, write the macro definition template in the file, and use thetext/template
package for processing.
//go:generate go run gen.go package main import ( "os" "text/template" ) func main() { templates := template.New("macros") template.Must(templates.ParseFiles("templates/macros.tmpl")) // 定义宏替换的参数 params := struct { Name string Number int }{ Name: "Macro", Number: 10, } // 宏替换 templates.ExecuteTemplate(os.Stdout, "macros.tmpl", params) }
- Create a
macros.tmpl
file in thetemplates
folder to store the template code for macro definitions. The sample code is as follows:
package main import "fmt" func {{.Name}}(x int) int { return x * {{.Number}} }
- Run the
go generate
command to generate a macro definition file:
go generate
Code example
Let’s take a look at a specific code example to show how to use macro definitions to optimize code in Golang:
package main import "fmt" //go:generate go run gen.go // 定义宏替换的参数 var params = struct { Name string Number int }{ Name: "Macro", Number: 10, } // 使用宏定义的函数 func main() { result := Macro(5) fmt.Println(result) }
Through the above steps, we have successfully used macro definitions to optimize code and avoid repeatedly writing code. Fragments improve code reusability.
Summary: In Golang, macro definition is a powerful code optimization tool that can help us reduce code duplication and improve code readability and maintainability. By understanding the role and practice of macro definitions, we can use macro definitions more flexibly in actual project development, optimize code structure, and improve code quality. Hope this article is helpful to you.
The above is the detailed content of Golang code optimization: the role and practice of macro definitions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
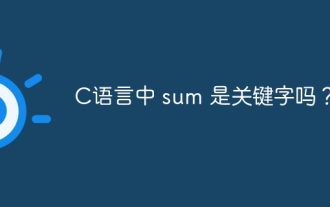
The sum keyword does not exist in C language, it is a normal identifier and can be used as a variable or function name. But to avoid misunderstandings, it is recommended to avoid using it for identifiers of mathematical-related codes. More descriptive names such as array_sum or calculate_sum can be used to improve code readability.
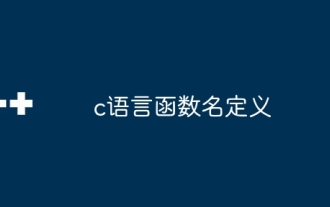
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
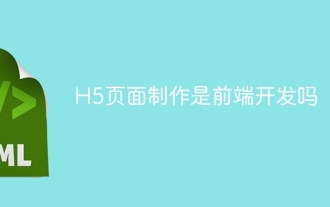
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
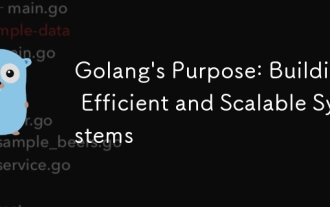
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
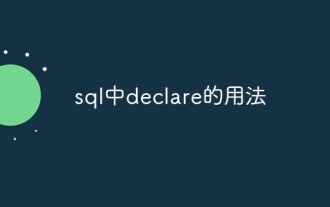
The DECLARE statement in SQL is used to declare variables, that is, placeholders that store variable values. The syntax is: DECLARE <Variable name> <Data type> [DEFAULT <Default value>]; where <Variable name> is the variable name, <Data type> is its data type (such as VARCHAR or INTEGER), and [DEFAULT <Default value>] is an optional initial value. DECLARE statements can be used to store intermediates
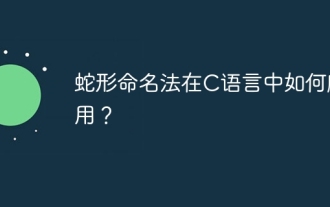
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
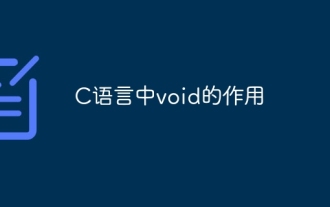
In C language, void is a keyword that indicates no return value. It is used in various scenarios, such as: a function that declares no return value: void print_message(); a function that declares no parameter: void print_message(void); a function that defines no return value: void print_message() { printf(&quot;Hello world\n&quot;); } A function that defines no parameter: void print_message(void) { printf(&quot;Hell
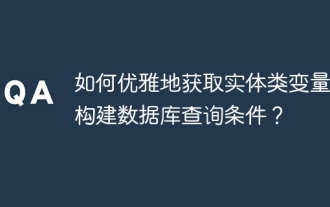
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
