


An in-depth analysis of Java multithreading: exploring different implementation methods
In-depth analysis of Java multithreading: exploring different implementation methods, requiring specific code examples
Abstract:
As a widely used programming language, Java provides Rich multi-threading support. This article will delve into the implementation of Java multi-threading, including inheriting the Thread class, implementing the Runnable interface, and using the thread pool. Through specific code examples, readers will be able to better understand and apply these methods.
- Introduction
Multi-threaded programming is an important technology that can make full use of multi-core processors and improve program performance. In Java, multi-threading can be implemented by inheriting the Thread class, implementing the Runnable interface, and using a thread pool. Different implementations are suitable for different scenarios, and they will be introduced and compared one by one next. - Inherit the Thread class
Inheriting the Thread class is a simple way to implement multi-threading. Define the execution logic of the thread by creating a subclass of the Thread class and overriding the run() method in the subclass. The following is a sample code that uses the inherited Thread class to implement multi-threading:
public class MyThread extends Thread { @Override public void run() { for (int i = 0; i < 10; i++) { System.out.println("Thread 1: " + i); } } } public class Main { public static void main(String[] args) { MyThread thread1 = new MyThread(); thread1.start(); for (int i = 0; i < 10; i++) { System.out.println("Main thread: " + i); } } }
- Implementing the Runnable interface
The way of inheriting the Thread class has certain limitations, because Java is single inheritance . In order to overcome this limitation, we can implement the Runnable interface and override the run() method in the implementation class to define the execution logic of the thread. The following is a sample code that uses the Runnable interface to implement multi-threading:
public class MyRunnable implements Runnable { @Override public void run() { for (int i = 0; i < 10; i++) { System.out.println("Thread 2: " + i); } } } public class Main { public static void main(String[] args) { MyRunnable myRunnable = new MyRunnable(); Thread thread2 = new Thread(myRunnable); thread2.start(); for (int i = 0; i < 10; i++) { System.out.println("Main thread: " + i); } } }
- Using a thread pool
Using a thread pool can better manage and reuse threads and avoid frequent creation and destroy the thread. Java provides the ExecutorService interface and its implementation class ThreadPoolExecutor to support the use of thread pools. The following is a sample code that uses a thread pool to implement multi-threading:
public class MyTask implements Runnable { private int taskId; public MyTask(int taskId) { this.taskId = taskId; } @Override public void run() { System.out.println("Task " + taskId + " is running."); } } public class Main { public static void main(String[] args) { ExecutorService executorService = Executors.newFixedThreadPool(5); for (int i = 0; i < 10; i++) { MyTask task = new MyTask(i); executorService.execute(task); } executorService.shutdown(); } }
- Summary
By inheriting the Thread class, implementing the Runnable interface and using the thread pool, we can effectively implement Java multi-threading thread. In actual development, we need to choose an appropriate implementation method according to specific needs. Inheriting the Thread class is suitable for simple thread tasks, implementing the Runnable interface is suitable for scenarios that require multiple inheritance, and using the thread pool can better manage threads. Through the introduction and sample code of this article, readers should have a deeper understanding and mastery of Java multithreading.
Reference:
- Oracle. (n.d.). The Java™ Tutorials - Lesson: Concurrency. Oracle. Retrieved from https://docs.oracle.com/ javase/tutorial/essential/concurrency/
The above is the detailed content of An in-depth analysis of Java multithreading: exploring different implementation methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










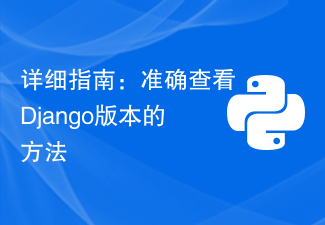
For an in-depth analysis of how to accurately view the Django version, specific code examples are required. Introduction: As a popular Python Web framework, Django often requires version management and upgrades. However, sometimes it may be difficult to check the Django version number in the project, especially when the project has entered the production environment, or uses a large number of custom extensions and partial modules. This article will introduce in detail how to accurately check the version of the Django framework, and provide some code examples to help developers better manage
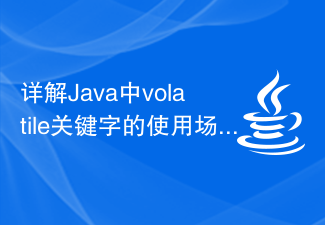
Detailed explanation of the role and application scenarios of the volatile keyword in Java 1. The role of the volatile keyword In Java, the volatile keyword is used to identify a variable that is visible between multiple threads, that is, to ensure visibility. Specifically, when a variable is declared volatile, any modifications to the variable are immediately known to other threads. 2. Application scenarios of the volatile keyword The status flag volatile keyword is suitable for some status flag scenarios, such as a
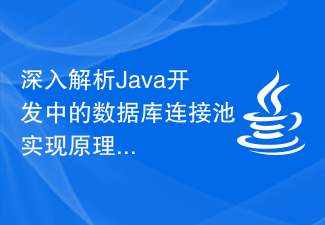
In-depth analysis of the implementation principle of database connection pool in Java development. In Java development, database connection is a very common requirement. Whenever we need to interact with the database, we need to create a database connection and then close it after performing the operation. However, frequently creating and closing database connections has a significant impact on performance and resources. In order to solve this problem, the concept of database connection pool was introduced. The database connection pool is a caching mechanism for database connections. It creates a certain number of database connections in advance and
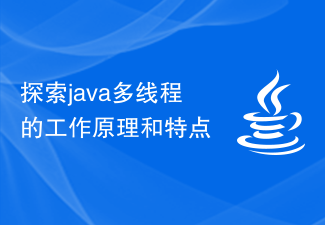
Explore the working principles and characteristics of Java multithreading Introduction: In modern computer systems, multithreading has become a common method of concurrent processing. As a powerful programming language, Java provides a rich multi-threading mechanism, allowing programmers to better utilize the computer's multi-core processor and improve program running efficiency. This article will explore the working principles and characteristics of Java multithreading and illustrate it with specific code examples. 1. The basic concept of multi-threading Multi-threading refers to executing multiple threads at the same time in a program, and each thread processes different
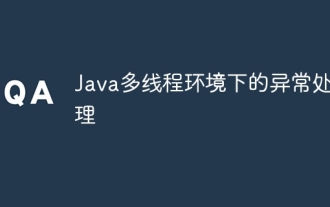
Key points of exception handling in a multi-threaded environment: Catching exceptions: Each thread uses a try-catch block to catch exceptions. Handle exceptions: print error information or perform error handling logic in the catch block. Terminate the thread: When recovery is impossible, call Thread.stop() to terminate the thread. UncaughtExceptionHandler: To handle uncaught exceptions, you need to implement this interface and assign it to the thread. Practical case: exception handling in the thread pool, using UncaughtExceptionHandler to handle uncaught exceptions.
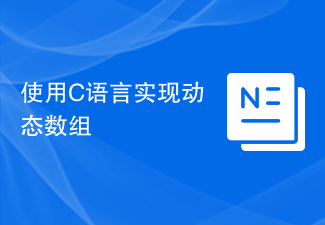
Dynamic array C language implementation method Dynamic array refers to a data structure that can dynamically allocate and release memory as needed during program running. Compared with static arrays, the length of dynamic arrays can be dynamically adjusted at runtime, thus more flexibly meeting the needs of the program. In C language, the implementation of dynamic arrays relies on the dynamic memory allocation functions malloc and free. The malloc function is used to apply for a memory space of a specified size, while the free function is used to release the previously applied memory space. Below is an example
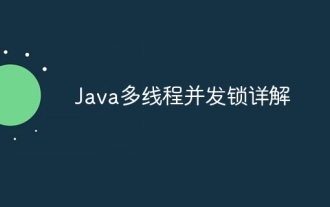
The Java concurrency lock mechanism ensures that shared resources are accessed by only one thread in a multi-threaded environment. Its types include pessimistic locking (acquire the lock and then access) and optimistic locking (check for conflicts after accessing). Java provides built-in concurrency lock classes such as ReentrantLock (mutex lock), Semaphore (semaphore) and ReadWriteLock (read-write lock). Using these locks can ensure thread-safe access to shared resources, such as ensuring that when multiple threads access the shared variable counter at the same time, only one thread updates its value.
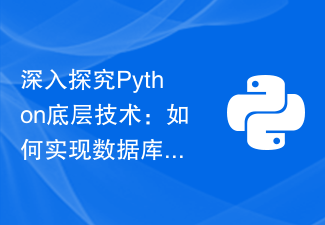
In-depth exploration of Python's underlying technology: how to implement database connection pooling Introduction: In modern application development, the database is an indispensable part. For database connections and management, connection pooling is a very important technology. This article will delve into how to implement a simple database connection pool in Python and provide specific code examples. 1. What is a database connection pool? A database connection pool is a technology for managing database connections. It maintains a certain number of database connections and effectively manages and manages the connections.
