


Overcoming the maze of Python syntax: making the code work for you
#python Basic syntax of syntax
Python is an interpreted language, which means that it executes code line by line without converting it into machine code like compiled languages. Python syntax is known for its simplicity and readability, which makes it an excellent choice for beginners and professionals alike.
Variables and data types
Variables are used to store information. In Python, you can use the equal sign (=) to assign a value to a variable. The data type determines the type of value stored in the variable, such as string, integer, or floating point number.
my_name = "John"# 字符串 age = 30# 整数 salary = 1200.50# 浮点数
data structure
Data structures are containers used to organize and store data. Python provides a variety of data structures, including lists, tuples, dictionaries, and sets.
A list is a mutable ordered collection used to store items.
my_list = [1, 2, 3, "apple", "banana"]
Tuple is an immutable ordered collection used to store items. Unlike lists, tuples cannot be modified.
my_tuple = (1, 2, 3, "apple", "banana")
Dictionary is an unordered collection of key-value pairs. It allows you to access values by key.
my_dict = {"name": "John", "age": 30, "salary": 1200.50}
Set is an unordered collection of unique elements. Unlike lists, there are no duplicate elements in sets.
my_set = {1, 2, 3, "apple", "banana"}
Conditional statements
Conditional statements are used to execute code based on specific conditions. Conditional statements in Python include if statements, elif statements, and else statements.
if age >= 18: print("你已成年。") elif age < 18: print("你未成年。") else: print("无效的年龄。")
loop statement
Loop statements are used to repeatedly execute a section of code. Loop statements in Python include for loops and while loops.
for loop is used to iterate through items in a sequence.
for item in my_list: print(item)
while loop is used to execute code as long as certain conditions are met.
while age < 18: print("你未成年。") age += 1
function
Functions are reusable blocks of code that receive parameters and return results.
def greet(name): return "你好," + name print(greet("John"))
kind
Classes are blueprints for creating objects. They define the object's state (properties and methods).
class Person: def __init__(self, name, age): self.name = name self.age = age john = Person("John", 30)
Master the syntax and unleash the power of Python
With a deep understanding of Python syntax, you can write clear, concise, and efficient code. It will provide you with a solid foundation for building powerful and scalable applications. By practicing and applying correct syntax principles, you'll be able to navigate the labyrinth of Python syntax and make the code work for you, unlocking Python's full potential.
The above is the detailed content of Overcoming the maze of Python syntax: making the code work for you. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










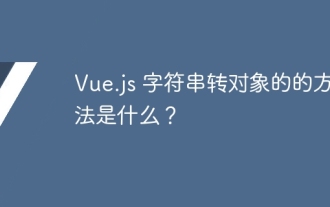
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.
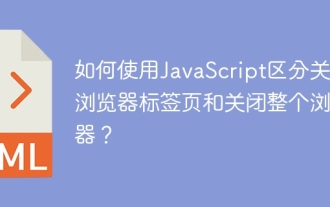
How to distinguish between closing tabs and closing entire browser using JavaScript on your browser? During the daily use of the browser, users may...
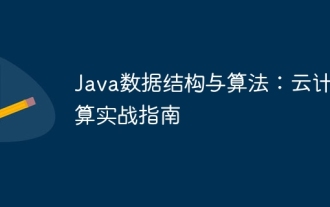
The use of data structures and algorithms is crucial in cloud computing for managing and processing massive amounts of data. Common data structures include arrays, lists, hash tables, trees, and graphs. Commonly used algorithms include sorting algorithms, search algorithms and graph algorithms. Leveraging the power of Java, developers can use Java collections, thread-safe data structures, and Apache Commons Collections to implement these data structures and algorithms.
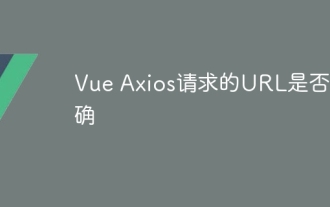
Yes, the URL requested by Vue Axios must be correct for the request to succeed. The format of url is: protocol, host name, resource path, optional query string. Common errors include missing protocols, misspellings, duplicate slashes, missing port numbers, and incorrect query string format. How to verify the correctness of the URL: enter manually in the browser address bar, use the online verification tool, or use the validateStatus option of Vue Axios in the request.

HadiDB: A lightweight, high-level scalable Python database HadiDB (hadidb) is a lightweight database written in Python, with a high level of scalability. Install HadiDB using pip installation: pipinstallhadidb User Management Create user: createuser() method to create a new user. The authentication() method authenticates the user's identity. fromhadidb.operationimportuseruser_obj=user("admin","admin")user_obj.
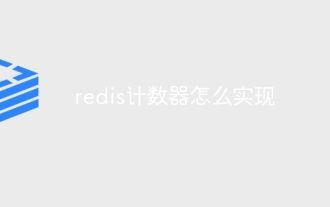
Redis counter is a mechanism that uses Redis key-value pair storage to implement counting operations, including the following steps: creating counter keys, increasing counts, decreasing counts, resetting counts, and obtaining counts. The advantages of Redis counters include fast speed, high concurrency, durability and simplicity and ease of use. It can be used in scenarios such as user access counting, real-time metric tracking, game scores and rankings, and order processing counting.
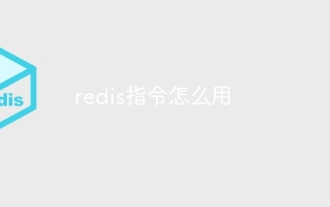
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
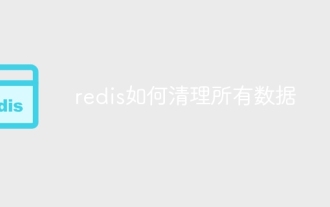
How to clean all Redis data: Redis 2.8 and later: The FLUSHALL command deletes all key-value pairs. Redis 2.6 and earlier: Use the DEL command to delete keys one by one or use the Redis client to delete methods. Alternative: Restart the Redis service (use with caution), or use the Redis client (such as flushall() or flushdb()).
