Java Polymorphism: Uncovering the magic hidden in your code
The principle of polymorphism
Java polymorphism is an important concept in object-oriented programming, making the code more flexible and scalable. PHP editor Banana will reveal the magic of polymorphism hidden in the code for you, allowing you to have an in-depth understanding of the principles and applications of polymorphism. Through this article, you will master the core concepts of polymorphism, explore its practical application in Java programming, help you better use polymorphism features, and improve the readability and flexibility of your code. Let us uncover the mystery of Java polymorphism and explore its mysteries!
For example, we can define a base class Animal
that has a method named makeSound()
that returns the sound made by the animal. Then, we can create derived classes Cat
and Dog
to inherit the Animal
class:
public class Animal { public String makeSound() { return "Unknown animal sound"; } } public class Cat extends Animal { @Override public String makeSound() { return "Meow"; } } public class Dog extends Animal { @Override public String makeSound() { return "Woof"; } }
Now, we can use the reference of the base class Animal
to point to the object of the derived class. This allows us to handle different types of animals uniformly in our programs without having to worry about their specific implementation details. For example, we could write the following code to make all animals make sounds:
List<Animal> animals = new ArrayList<>(); animals.add(new Cat()); animals.add(new Dog()); for (Animal animal : animals) { System.out.println(animal.makeSound()); }
The output result is:
Meow Woof
From the above example, we can see that polymorphism allows us to use a unified interface to handle different types of objects, thus simplifying the code and improving reusability.
Benefits of polymorphism
Using polymorphism can bring many benefits, including:
- Improve the scalability of the code: When we need to add a new animal type, we only need to create a new derived class and implement the
makeSound()
method without modifying the base class or other Derived class. - Improve code reusability: We can use base class references to point to objects of derived classes, so that the same code can be reused in different parts of the program.
- Improve the maintainability of code: Polymorphism makes the code easier to understand and maintain because we can use a unified interface to handle different types of objects.
Application scenarios of polymorphism
Polymorphism has many application scenarios in actual development, such as:
- Graphical User Interface (GUI): In GUI, we can use polymorphism to create different controls, such as buttons, text boxes, drop-down lists, etc., and use a unified interface to handle these controls.
- Data access: In data access, we can use polymorphism to create different data access objects (DAO), such as JDBC, Hibernate, mybatis, etc., and use a unified interface to accessdatabase.
- Network Programming: In NetworkProgramming, we can use polymorphism to create different network protocols, such as tcp , UDP, Http, etc., and use a unified interface to send and receive data.
in conclusion
Polymorphism is a very important concept in Java programming, which can help us write more flexible, more extensible and more reusable code. This article introduces the principles, benefits and application scenarios of polymorphism, and hopes to be helpful to readers.
The above is the detailed content of Java Polymorphism: Uncovering the magic hidden in your code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










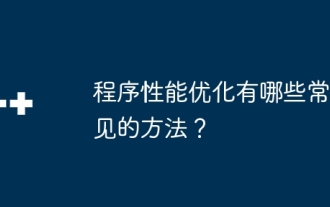
Program performance optimization methods include: Algorithm optimization: Choose an algorithm with lower time complexity and reduce loops and conditional statements. Data structure selection: Select appropriate data structures based on data access patterns, such as lookup trees and hash tables. Memory optimization: avoid creating unnecessary objects, release memory that is no longer used, and use memory pool technology. Thread optimization: identify tasks that can be parallelized and optimize the thread synchronization mechanism. Database optimization: Create indexes to speed up data retrieval, optimize query statements, and use cache or NoSQL databases to improve performance.
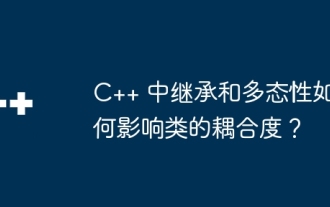
Inheritance and polymorphism affect the coupling of classes: Inheritance increases coupling because the derived class depends on the base class. Polymorphism reduces coupling because objects can respond to messages in a consistent manner through virtual functions and base class pointers. Best practices include using inheritance sparingly, defining public interfaces, avoiding adding data members to base classes, and decoupling classes through dependency injection. A practical example showing how to use polymorphism and dependency injection to reduce coupling in a bank account application.
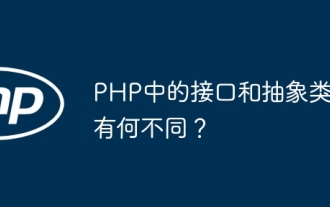
Interfaces and abstract classes are used to create extensible PHP code, and there is the following key difference between them: Interfaces enforce through implementation, while abstract classes enforce through inheritance. Interfaces cannot contain concrete methods, while abstract classes can. A class can implement multiple interfaces, but can only inherit from one abstract class. Interfaces cannot be instantiated, but abstract classes can.
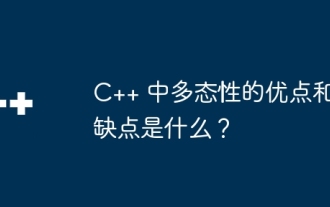
Advantages and Disadvantages of C++ Polymorphism: Advantages: Code Reusability: Common code can handle different object types. Extensibility: Easily add new classes without modifying existing code. Flexibility and maintainability: separation of behavior and type improves code flexibility. Disadvantages: Runtime overhead: Virtual function dispatch leads to increased overhead. Code Complexity: Multiple inheritance hierarchies add complexity. Binary size: Virtual function usage increases binary file size. Practical case: In the animal class hierarchy, polymorphism enables different animal objects to make sounds through Animal pointers.
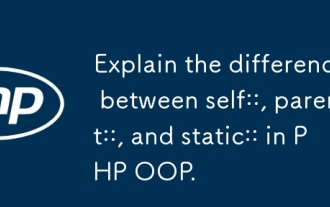
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
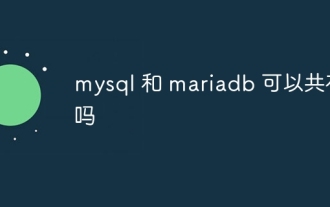
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
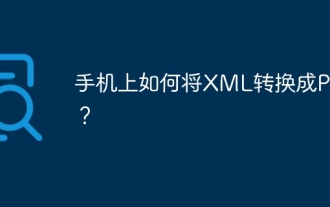
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
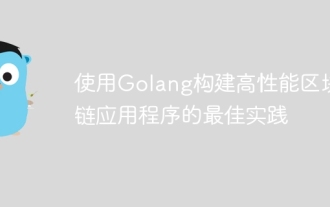
Best practices for building high-performance blockchain applications with GoLang: Concurrency: Use goroutines and channels for concurrent task processing to avoid blocking. Memory management: Use object pools and caches to reduce garbage collection overhead, and choose efficient data structures such as slicing. Data structure selection: Select appropriate data structures, such as hash tables and B-trees, according to application requirements to optimize data access patterns. Performance Analysis and Optimization: Use performance analysis tools to identify bottlenecks, optimize algorithms and data structures, and fine-tune performance through benchmarking.
