gRPC does not return a boolean value if the value is false
When php editor Baicao introduced the use of gRPC, he pointed out that if the specified value is false in the gRPC request, then gRPC will not return a Boolean value. This means that when using gRPC, we need to pay attention to how return values are handled to avoid confusion and errors. Understanding this detail will help us better understand and apply the functions of gRPC, and improve our programming efficiency and code quality. Let's delve into more features and usage of gRPC to bring better performance and scalability to our projects.
Question content
func (m *todoserver) gettodos(ctx context.context, empty *emptypb.empty) (*desc.gettodosresponse, error) { todos, err := m.todoservice.gettodos() if err != nil { return nil, err } todosresp := make([]*desc.gettodosresponse_todo, 0, len(todos)) for _, todo := range todos { todosresp = append(todosresp, &desc.gettodosresponse_todo{ id: todo.id, title: todo.title, iscompleted: todo.iscompleted, }) } return &desc.gettodosresponse{todos: todosresp}, nil }
service TodoService { rpc GetTodos(google.protobuf.Empty) returns (GetTodosResponse) {} } message GetTodosResponse { repeated Todo todos = 1; message Todo { int64 id = 1; string title = 2; bool is_completed = 3; } }
service TodoService { rpc GetTodos(google.protobuf.Empty) returns (GetTodosResponse) {} } message GetTodosResponse { repeated Todo todos = 1; message Todo { int64 id = 1; string title = 2; bool is_completed = 3; } }
I have a record in the database |Number |Title |Completed | |-|-|-| | 1 |Ahhh|False|
The above function returns {"todos": [{"id": "1", "title": "aaa"}]}
But once I change is_completed
is true
, the result is correct{"todos ": [{"id": "1", "title": "aaa", "iscompleted": true}]}
Solution
This is by design and to improve efficiency.
The "zero" value ofbool
is false
- so when a protobuf
structure is initialized with a false
value, when using the standard This field does not need to be explicitly declared when using the library's encoding/json
unmarshaller. On the encoding side, if the field's json tag contains the omitempty
qualifier, the standard library's encoding/json
marshaler will remove any zero values - this is what you see of.
You will see the same behavior if the title
string field is ""
(i.e. the zero value of the string).
View the generated code (*.pb.go
), the bool
field definition of the structure will be as follows:
type todo struct { // ... iscompleted bool `protobuf:"varint,5,opt,name=is_complete,proto3" json:"is_complete,omitempty"` }
Thus json:"...,omitempty"
instructs the encoding/json
marshaler to omit any zero values during marshaling using these tags.
If you want to override this behavior:
- It is possible to remove the
omitempty
directive from the generated code (not recommended - due to the need to manage editing during the development life cycle). But if you must do this, see this answer; - If using
grpc-gateway
, please override it at runtime, for example
gwmux := runtime.newservemux(runtime.withmarshaleroption(runtime.mimewildcard, &runtime.jsonpb{origname: true, emitdefaults: true}))
- Or, if you export json yourself, instead of using the standard library (
encoding/json
), use thejson
marshaler" in this package google.golang.org/protobuf/encoding/protojson"
:
protojson.Marshaler{EmitDefaults: true}.Marshal(w, resp)
As mentioned in this answer.
The above is the detailed content of gRPC does not return a boolean value if the value is false. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










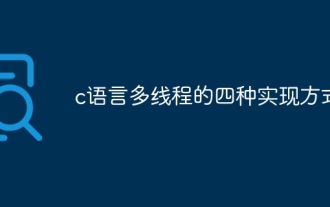
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
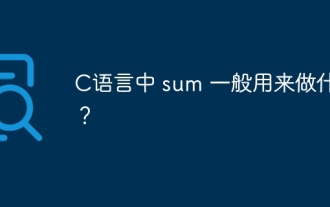
There is no function named "sum" in the C language standard library. "sum" is usually defined by programmers or provided in specific libraries, and its functionality depends on the specific implementation. Common scenarios are summing for arrays, and can also be used in other data structures, such as linked lists. In addition, "sum" is also used in fields such as image processing and statistical analysis. An excellent "sum" function should have good readability, robustness and efficiency.
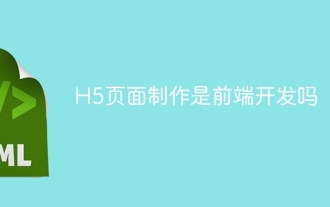
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
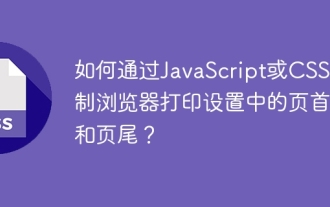
How to use JavaScript or CSS to control the top and end of the page in the browser's printing settings. In the browser's printing settings, there is an option to control whether the display is...
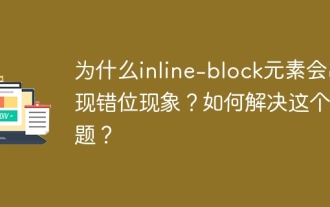
Regarding the reasons and solutions for misaligned display of inline-block elements. When writing web page layout, we often encounter some seemingly strange display problems. Compare...
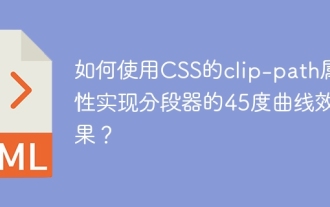
How to achieve the 45-degree curve effect of segmenter? In the process of implementing the segmenter, how to make the right border turn into a 45-degree curve when clicking the left button, and the point...
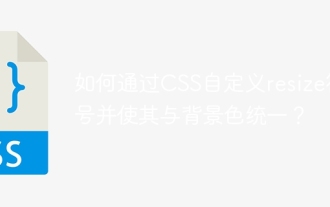
The method of customizing resize symbols in CSS is unified with background colors. In daily development, we often encounter situations where we need to customize user interface details, such as adjusting...
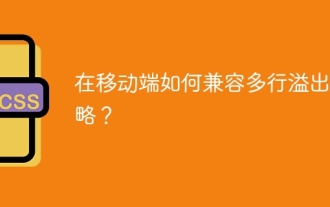
Compatibility issues of multi-row overflow on mobile terminal omitted on different devices When developing mobile applications using Vue 2.0, you often encounter the need to overflow text...
